1.2 Array Operations - Traversal, Insertion | Explanation with C Program | DSA Course
Education
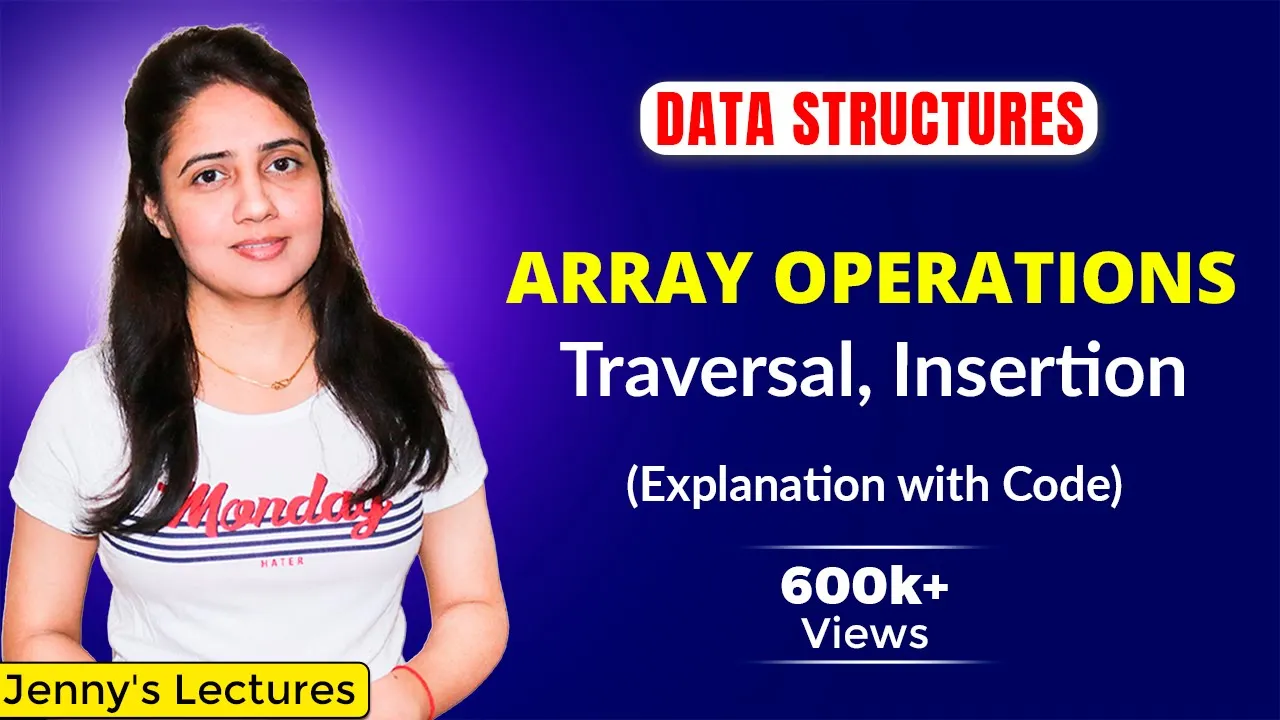
Introduction
In this article, we will explore various operations performed on one-dimensional arrays in data structures, specifically focusing on traversal and insertion. Understanding these operations is fundamental in grasping how arrays work in programming.
Overview of Array Operations
Arrays are a significant data structure that allows for the storage of multiple elements of the same type. The major operations that can be performed on arrays include:
- Traversal of an Array: Visiting every element of the array exactly once.
- Insertion in an Array: Adding elements at the beginning, end, or specific positions.
- Deletion of Data: Removing elements from the array.
- Searching for Elements: Checking if a specific element exists in the array.
- Sorting of Array: Arranging elements in a specific order.
Traversal of an Array
Traversal means accessing each element of the array to utilize its value. For instance, consider an array of integers. If we have the following data:
int a[5] = (6, 2, 4, 5, 0);
To traverse this array, we utilize a loop that iterates from 0 to the length of the array minus one, printing each element as we go.
C Code for Traversing an Array
#include <stdio.h>
int main() (
int a[5], size, i;
// Asking the user for the size of the array
printf("Enter size of array (max 5): ");
scanf("%d", &size);
// Ensure size does not exceed the allocated space
if (size > 5) {
printf("Invalid size. Maximum size is 5.\n");
return 0;
)
// Taking input from the user
printf("Enter elements of the array:\n");
for (i = 0; i < size; i++) (
scanf("%d", &a[i]);
)
// Traversing the array
printf("Elements in array are:\n");
for (i = 0; i < size; i++) (
printf("%d ", a[i]);
)
return 0;
}
Insertion in an Array
Inserting data into an array involves placing a new value into a specific location, pushing subsequent elements in the array if necessary. There are three types of insertions:
- At the Beginning: Shifting all existing elements one position to the right.
- At the End: Adding the new value at the end of the array without shifting.
- At a Specific Position: Shifting elements to make space for the new value.
Insertion Example
We will demonstrate how to insert a number into a specified position within an array.
C Code for Insertion at a Specific Position
#include <stdio.h>
int main() (
int a[50], size, pos, num, i;
// Maximum size of the array
printf("Enter size of array (max 50): ");
scanf("%d", &size);
// Check for overflow
if (size > 50) {
printf("Invalid size. Maximum size is 50.\n");
return 0;
)
// Input existing elements
printf("Enter elements of the array:\n");
for (i = 0; i < size; i++) (
scanf("%d", &a[i]);
)
// Taking input for number to insert and position
printf("Enter number to insert: ");
scanf("%d", &num);
printf("Enter position to insert (1 - %d): ", size + 1);
scanf("%d", &pos);
// Validate position
if (pos < 1 || pos > size + 1) (
printf("Invalid position.\n");
return 0;
)
// Shift elements to the right
for (i = size; i >= pos; i--) (
a[i] = a[i - 1];
)
// Insert new element
a[pos - 1] = num;
size++; // Increment size
// Output the updated array
printf("Updated array:\n");
for (i = 0; i < size; i++) (
printf("%d ", a[i]);
)
return 0;
}
Conclusion
Array traversal and insertion are key operations that enhance a programmer's ability to manipulate data structures effectively. Understanding how to work with these operations is crucial for any aspiring developer.
Keywords
Array operations, traversal, insertion, C programming, data structure, insertion at beginning, insertion at end, insertion at specific position, overflow condition, elements.
FAQ
Q1: What is array traversal?
A1: Array traversal is the process of accessing each element of the array sequentially to utilize or display its values.
Q2: How do I insert an element into an array in C?
A2: You can insert an element by shifting existing elements to make space at the desired position, and then placing the new element at that position.
Q3: What happens if I try to insert an element when the array is full?
A3: An overflow condition occurs, meaning you cannot insert any additional elements beyond the declared size of the array. It is essential to check the size before performing operations.
Q4: Can I insert an element at a position outside of the array's current size?
A4: No, you cannot insert an element outside the current valid range of positions for the array. Attempting to do so will lead to an error.