2490. Circular Sentence | LeetCode 2490 Explained in Java | Code Explanation & Dry Run
People & Blogs
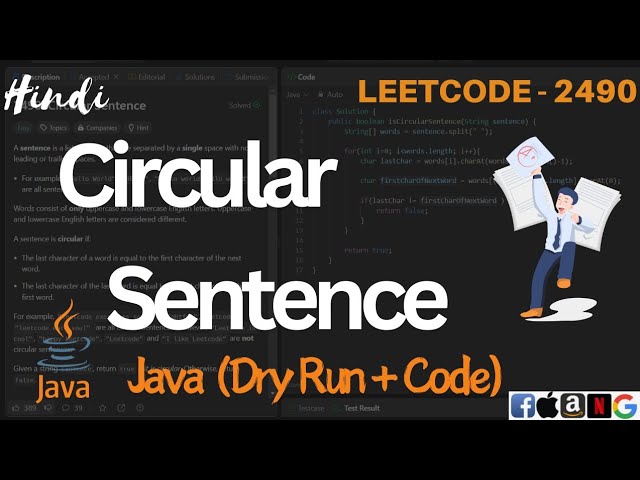
Introduction
In this article, we will discuss LeetCode Problem 2490, titled "Circular Sentence." This problem revolves around checking whether a sentence forms a circular pattern based on specific conditions we will outline.
Understanding Circular Sentences
A sentence is defined as a list of words separated by a single space, without leading or trailing spaces. Each word consists of upper and lower case English letters. Notably, upper case and lower case characters are treated as distinct.
Definition of a Circular Sentence
A sentence can be called circular if:
- The last character of a word matches the first character of the subsequent word.
- The last character of the last word matches the first character of the first word.
Examples
To illustrate this definition, consider the example:
Sentence:
"hello world"
- Here, the last character of "hello" (
o
) does not match the first character of "world" (w
), so this is not a circular sentence.
- Here, the last character of "hello" (
Sentence:
"NEET code"
- Last character of "NEET" (
T
) matched with the first character of "code" (c
). Hence, this is not a circular sentence as well but will be for sentences like"Code Editor"
where the last matches with the first.
- Last character of "NEET" (
These patterns provide a foundation for determining if a sentence is circular.
Solution Approach
To solve this problem, we need to:
- Split the sentence into words.
- Traverse the words and check the required conditions.
- If any condition fails, return false. If all pass, return true.
Code Explanation
The following is a Java implementation to address the problem effectively:
public class CircularSentenceChecker (
public boolean isCircularSentence(String sentence) {
String[] words = sentence.split(" ");
for (int i = 0; i < words.length; i++) {
char lastChar = words[i].charAt(words[i].length() - 1);
char nextChar = words[(i + 1) % words.length].charAt(0);
if (lastChar != nextChar) {
return false;
)
}
return true;
}
}
Dry Run
- Input:
"Code Editor"
- Split into words:
["Code", "Editor"]
- Check conditions:
- Word 0: Last character
e
(from "Code"), matches First characterE
(from "Editor"). - Word 1: Last character
r
(from "Editor"), would match first character of "Code" again, validates the circular condition.
- Word 0: Last character
- Output: True (the sentence is circular).
- Split into words:
Time and Space Complexity
- Time Complexity: O(n), where n is the number of words in the sentence.
- Space Complexity: O(n) due to the words array.
Conclusion
With these principles and a methodical approach, we can effectively determine if a sentence is circular. Thank you for following along with this detailed explanation.
Keyword
- Circular Sentence
- Java Implementation
- String Split
- Time Complexity
- Space Complexity
- Character Matching
FAQ
Q1: What is a Circular Sentence?
A1: A circular sentence is one where the last character of each word matches the first character of the next word, including the last word's last character matching the first word's first character.
Q2: How do you check if a sentence is circular?
A2: By splitting the sentence into words and checking the character conditions as described, we can determine if it is a circular sentence.
Q3: What is the time complexity of the solution?
A3: The time complexity is O(n), where n is the number of words in the sentence.
Q4: Can upper and lower case be considered the same in a circular sentence?
A4: No, upper case and lower case English letters are treated as distinct, hence comparisons are case-sensitive.
Q5: What happens if there are leading or trailing spaces in the sentence?
A5: The problem states that the sentence will not have leading or trailing spaces, thus they need to be ensured during input.
By following this guide, you should be well-equipped to tackle the problem of determining circular sentences. Happy coding!