46 Audio book maker using python
Education
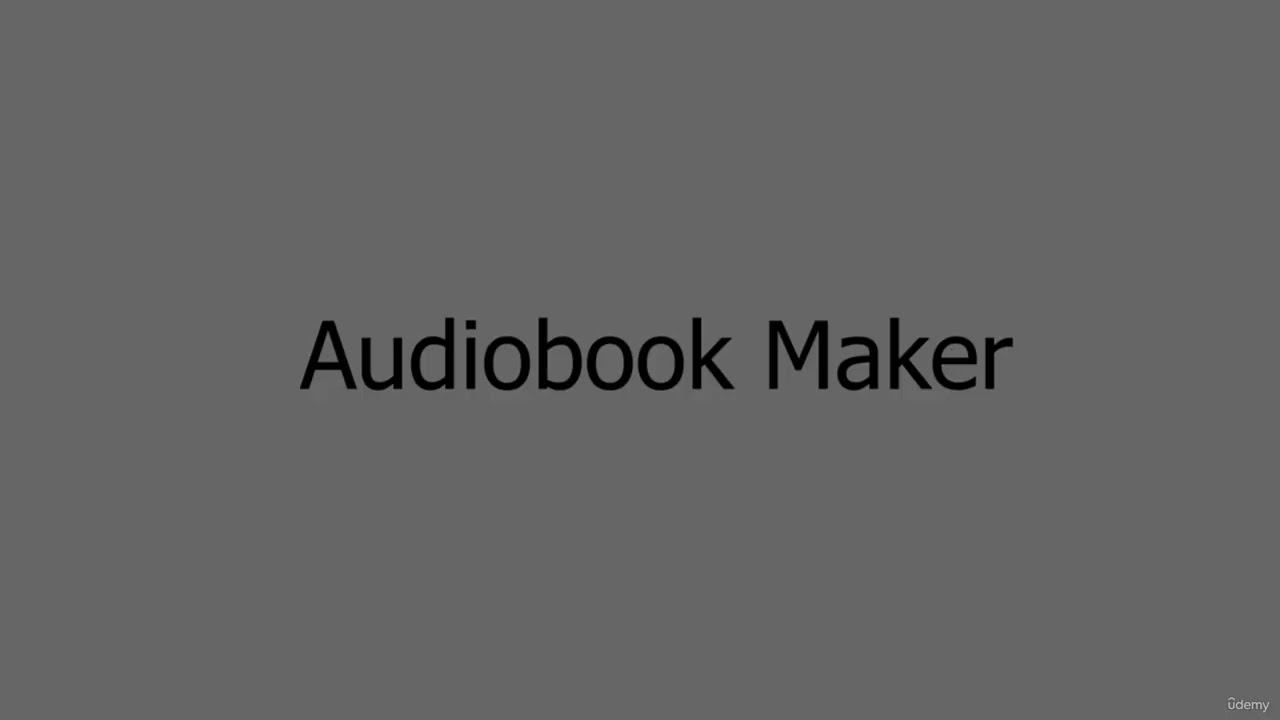
Introduction
In this tutorial, we will create a simple audio book maker leveraging Python's capabilities. We will utilize two important libraries: PyPDF2
for reading PDF files and pyttsx3
for converting text to speech. This tutorial assumes you have a basic understanding of Python programming.
Step 1: Install Required Packages
Before we start coding, we need to install the necessary packages. Open your terminal or command prompt and run the following commands:
pip install PyPDF2
pip install pyttsx3
Note: If you already have PyPDF2
, you should see a message saying "requirement already fulfilled". If not, it will be installed for you.
Step 2: Create Your Python Script
Now, let's create a new Python script for our audio book maker.
- Import Required Libraries:
The first line of the code will import the
PyPDF2
library.
import PyPDF2
import pyttsx3
- Define Your PDF File:
Specify the PDF file you want to read. In this example, we will use
biotechnology.pdf
.
pdf_file_path = 'biotechnology.pdf'
- Read the PDF File: Open the PDF file and create a PDF reader object.
with open(pdf_file_path, 'rb') as file:
pdf_reader = PyPDF2.PdfFileReader(file)
- Initialize Text-to-Speech Engine:
Initialize the
pyttsx3
engine, which will convert the text into speech.
speaker = pyttsx3.init()
- Iterate Over Each Page: Use a loop to read through all the pages of the PDF and extract the text.
for page_number in range(pdf_reader.getNumPages()):
page = pdf_reader.getPage(page_number)
text = page.extractText()
# Make the speaker say the extracted text
speaker.say(text)
speaker.runAndWait() # Wait until the speech is finished
## Introduction
speaker.stop()
- Save the Audio: While the basic example above reads and speaks the text, you might want to save the audio output as an .mp3 file. To do this, you would need to make further modifications and handle audio file saving, which may involve different libraries or methods.
Conclusion
The audio book maker developed in this tutorial demonstrates how to read text from a PDF file and convert it into speech using Python. You can enhance this project further by creating a GUI or adding more functionalities to customize the user experience.
Thank you for following along, and happy coding!
Keywords
- Python
- Audio Book Maker
- PyPDF2
- pyttsx3
- Text-to-Speech
- PDF Reader
FAQ
What is PyPDF2
used for?
PyPDF2
is a Python library for reading and manipulating PDF files, which allows users to extract text, merge, and split PDFs.
What does pyttsx3
do?
pyttsx3
is a text-to-speech conversion library in Python that converts given text into spoken words.
How do I install the required packages?
You can install the needed packages using the pip command: pip install PyPDF2 pyttsx3
.
Can I make an audio book from any PDF?
Yes, you can create an audio book from any PDF that contains extractable text.
Is it possible to save the spoken text as an audio file?
Saving the audio requires additional steps and modifications to the basic script, potentially using other libraries or methods designed for audio file handling.