Use Artificial Intelligence (AI) to Predict the Stock Market with Python
Science & Technology
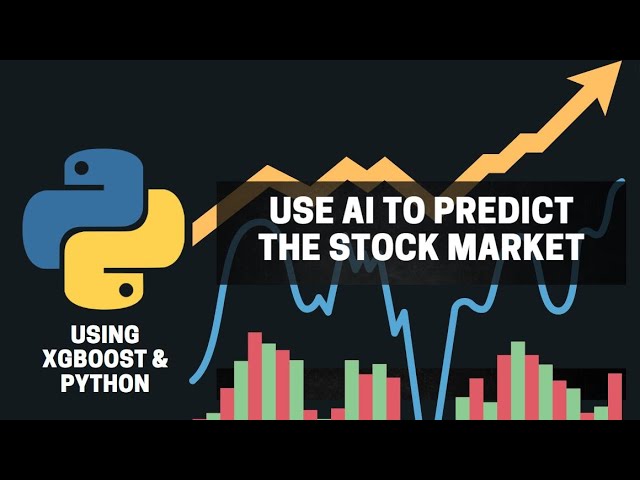
Use Artificial Intelligence (AI) to Predict the Stock Market with Python
In this article, we will explore how to use artificial intelligence (AI) to predict the price of the S&P 500 using the XGBoost machine learning algorithm in Python. We will walk through the process step by step, analyzing and visualizing the data, training the model, making predictions, and evaluating the model's accuracy.
Importing the Libraries
We will start by importing the necessary libraries for this program. We will need the pandas library for data manipulation, the XGBoost library (xgboost) for the machine learning algorithm, and the Matplotlib library (matplotlib.pyplot) for data visualization. Here is the code to import these libraries:
import pandas as pd
import xgboost as xgb
import matplotlib.pyplot as plt
Loading the Dataset
Next, we will load the dataset for the S&P 500. We will use the pandas library to read a CSV file containing the data. We will create a variable called data
and set it equal to pd.read_csv('spy.csv')
, where 'spy.csv' is the name of the file. Here is the code to load the dataset:
data = pd.read_csv('spy.csv')
Analyzing and Visualizing the Data
To get a better understanding of the data, we can analyze and visualize it. The dataset contains columns such as date, open price, high price, low price, close price, adjusted close price, and volume.
To display the data, we can simply run data
in the code. Additionally, we can use the Matplotlib library to plot the closing price of the S&P 500. Here is the code for plotting:
plt.plot(data['close'])
plt.show()
Splitting the Data into Training and Testing Sets
Before training our model, we need to split the data into training and testing sets. The training set will be used to train the model, while the testing set will be used to evaluate the model's performance.
We can use the pandas library to split the data. We'll create variables called train_data
and test_data
to hold the training and testing sets, respectively. Here is the code to split the data:
train_data = data.iloc[:int(0.99*len(data)), :]
test_data = data.iloc[int(0.99*len(data)):, :]
Defining the Features and Target Variable
Next, we need to define the features we want to use and the target variable. In this case, we will use the opening price and volume as features to predict the closing price.
We'll create a variable called features
and set it equal to ['open', 'volume']
. We'll also create a variable called target
and set it equal to 'close'
. Here is the code to define the features and target:
features = ['open', 'volume']
target = 'close'
Creating and Training the XGBoost Model
Now, we'll create and train the XGBoost model. We'll use the XGBoost regressor to perform regression, as we are predicting numerical values. We'll create a variable called model
and set it equal to xgb.XGBRegressor()
. Then we'll train the model using the training data, features, and target variable. Here is the code to create and train the model:
model = xgb.XGBRegressor()
model.fit(train_data[features], train_data[target])
Making Predictions and Evaluating the Model
After training the model, we can make predictions on the test data and evaluate the model's accuracy. We'll create a variable called predictions
and set it equal to model.predict(test_data[features])
. Then, we can print the model's predictions and the actual values from the test data. Finally, we'll calculate and print the accuracy of the model. Here is the code to make predictions and evaluate the model:
print('Model Predictions:')
print(predictions)
print('Actual Values:')
print(test_data[target])
accuracy = model.score(test_data[features], test_data[target])
print('Model Accuracy:', accuracy)
Plotting the Predictions and Actual Values
To visualize the predictions and actual values, we can plot them on a line graph. We'll use the Matplotlib library to plot the closing price from the test data as blue and the predictions as an orange-like color. Here is the code to plot the predictions and actual values:
plt.plot(data['close'], label='Close Price')
plt.plot(test_data.index, predictions, label='Predictions')
plt.legend()
plt.show()
Conclusion
In this article, we have seen how to use artificial intelligence (AI) and the XGBoost algorithm to predict the price of the S&P 500. We loaded and analyzed the data, split it into training and testing sets, defined the features and target variable, created and trained the model, made predictions, evaluated the model's accuracy, and visualized the results. Remember, the model's predictions are not guaranteed, and investing should be done with caution and thorough research.
Keywords: Python, AI, artificial intelligence, machine learning, XGBoost, S&P 500, prediction, data analysis, data visualization, accuracy.
FAQ
Q: What is XGBoost? A: XGBoost stands for Extreme Gradient Boosting, which is a machine learning library used to build models for regression, classification, and ranking.
Q: How accurate is the model? A: The model's accuracy can vary, but in this case, it achieved an accuracy of about 70%.
Q: Can this model be used to predict other stocks? A: Yes, the same approach can be applied to other stocks by using their respective datasets.
Q: Should I solely rely on this model for my investment decisions? A: No, it is essential to consider this model's predictions alongside other factors and conduct your own thorough research before making investment decisions.
Q: Are there any limitations to using AI for stock market prediction? A: Yes, stock market prediction is a complex task, and AI models may not account for all factors that affect stock prices. It is crucial to be cautious and not solely rely on AI predictions.
Q: How can I further improve the accuracy of the model? A: You can experiment with different features, optimize model parameters, and consider using more advanced techniques such as ensemble methods or deep learning models.
Q: Can I apply this approach to other financial markets? A: Yes, the same approach can be applied to other financial markets, such as forex or commodity markets, by using their respective datasets.
Q: Where can I find more resources to learn about AI and machine learning for stock market prediction? A: There are various online courses, books, and tutorials available that cover AI and machine learning for stock market prediction. Some popular platforms include Coursera, Udemy, and Kaggle.
Q: Can I use a different machine learning algorithm instead of XGBoost? A: Yes, there are several other algorithms available for stock market prediction, such as random forests, support vector machines, or neural networks. It is worth experimenting with different algorithms to find the one that best fits your specific problem.
Q: How can I download the code and dataset used in this article? A: You can find the code and dataset on the author's Patreon page by supporting the channel. The link will be available in the description of the article.