AI Blog: Laravel & Vue.js with TypeScript Part 3
Education
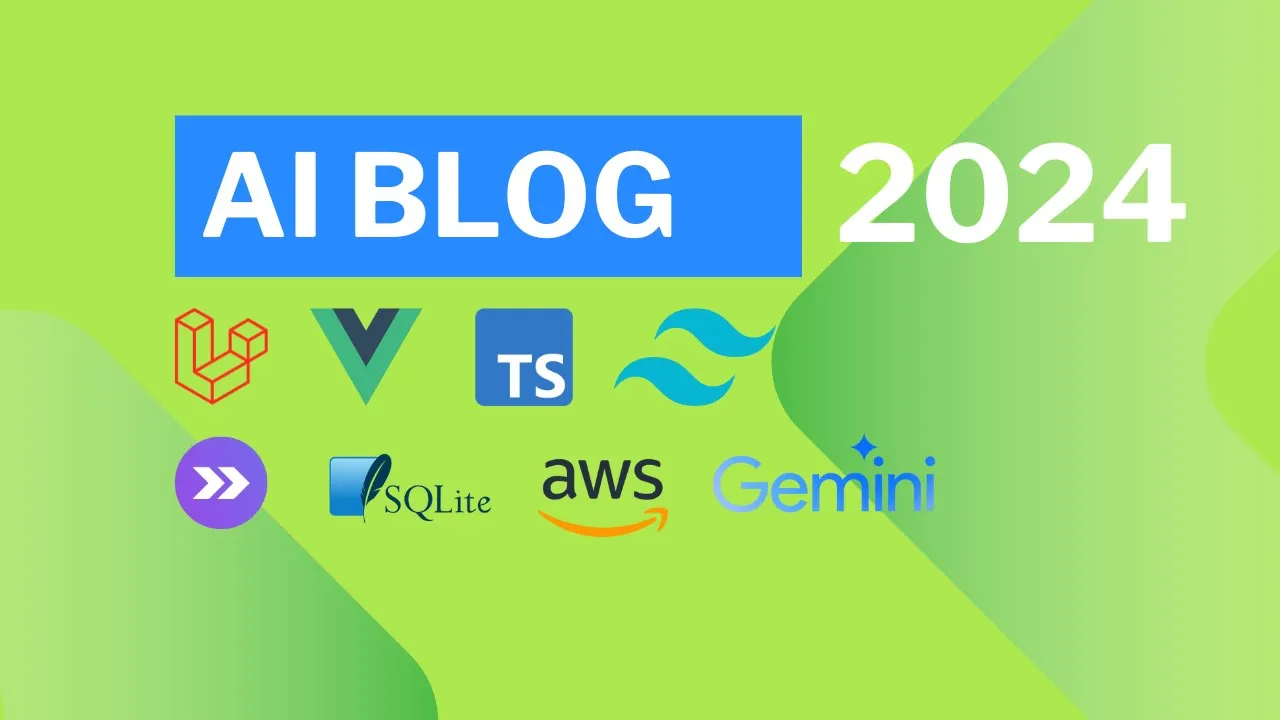
Introduction
Introduction
In this third part of our blog series on integrating AI with Laravel and Vue.js, we continue our exploration of generating content using Jiminy AI. This part focuses on how to create and push content to GitHub, programming the UI to gather the AI-generated text, and ensuring a successful integration with our existing Laravel project.
Extended Tutorial Duration
This part of the tutorial is longer than usual due to the detailed explanation of documentation that has taken about one hour per part.
Setting Up the Environment
Project and GitHub Repository
We first mention pushing the project into the GitHub repository, which can be found in the YouTube description. To ensure continuous and easy access to our code, we will focus on the following file location: create.vue
.
Adding Features to the User Interface
Creating a Button for AI Content Generation
We begin by positioning an AI-triggering button next to our text area input box. Using Tailwind CSS, we specify the button styles and make it clickable.
<span class="absolute right-0 bg-blue-500 rounded-full cursor-pointer h-8 w-8">
<svg class="w-12 h-12 text-white"></svg>
</span>
Integrating AI Drawer Component
Leaning on the Element Plus library, we import and implement a drawer component. This drawer will fetch and display AI-generated content.
import ( ElDrawer ) from 'element-plus';
The drawer will appear from the bottom of the screen upon clicking the AI button, showing inputs for AI prompts and generated results.
Programming Content Generation Logic
Setting Up Routes
We set up a new route for our AI content generation in the web.php
file.
Route::post('blog/create', [BlogPostController::class, 'generateAI']);
AI Configuration
To interact with Jiminy AI
, ensure you have your API key:
jiminy.api_key= your_api_key_here
API Interaction Using Laravel Controller
Inside the BlogPostController
, we create a function to handle content generation.
public function generateAI(Request $request)
(
$apiKey = config('services.jiminy.api_key');
$prompt = $request->input('prompt');
// Call Jiminy AI API
$generatedContent = ...;
return view('blog.create', ['content' => $generatedContent]);
)
Handling Responses and User Interface Updates
Frontend Handling
We utilize Vue.js with additional properties to manage and display the generated content effectively.
const generatePOEM = async () => (
try {
// Process submission to server and wait for AI-generated content
const response = await axios.post('/blog/create', { prompt: content.value ));
content.value = response.data.content;
} catch (error) (
console.error('Error generating content:', error);
)
};
Notifications and UX Improvements
We integrate an element notification component to provide users with real-time feedback.
import ( ElNotification ) from 'element-plus';
const notifySuccess = (message) => (
ElNotification({
title: 'Success',
message,
type: 'success',
));
};
Conclusion
This part detailed how to extend a Laravel and Vue.js project to interact with Jiminy AI to generate blog content. It covered setting up routes, interacting with the AI API, and ensuring a seamless front-end experience with real-time updates and notifications.
Keywords
- Laravel
- Vue.js
- TypeScript
- Jiminy AI
- Content Generation
- API Integration
- User Interface (UI)
- Element Plus
- Tailwind CSS
- Notifications
FAQ
Q1: What is the purpose of using Jiminy AI in the project? A1: Jiminy AI is used for generating content, such as poems or other text, which can be integrated into our blog application, enhancing content creation efficiency.
Q2: How is the AI button integrated into the existing user interface? A2: The AI button is added next to a text area input using Tailwind CSS for styling. When clicked, it triggers an AI content generation process and displays the result in a drawer.
Q3: What packages are necessary for this AI integration? A3: Necessary packages include Jiminy AI and Element Plus for the drawer component and notifications.
Q4: How do you ensure the content is valid before sending it for AI processing?
A4: Validation is implemented server-side in the controller method using Laravel's validate
method.
Q5: How is user feedback managed after content generation? A5: User feedback is managed using the Element Plus notification component to inform users regarding the success or failure of the content generation.
Q6: Where do you need to configure the Jiminy AI API key?
A6: The Jiminy AI API key goes into the .env
configuration file of your Laravel project.