AI-Powered Refactoring: Elevating Code Quality
Science & Technology
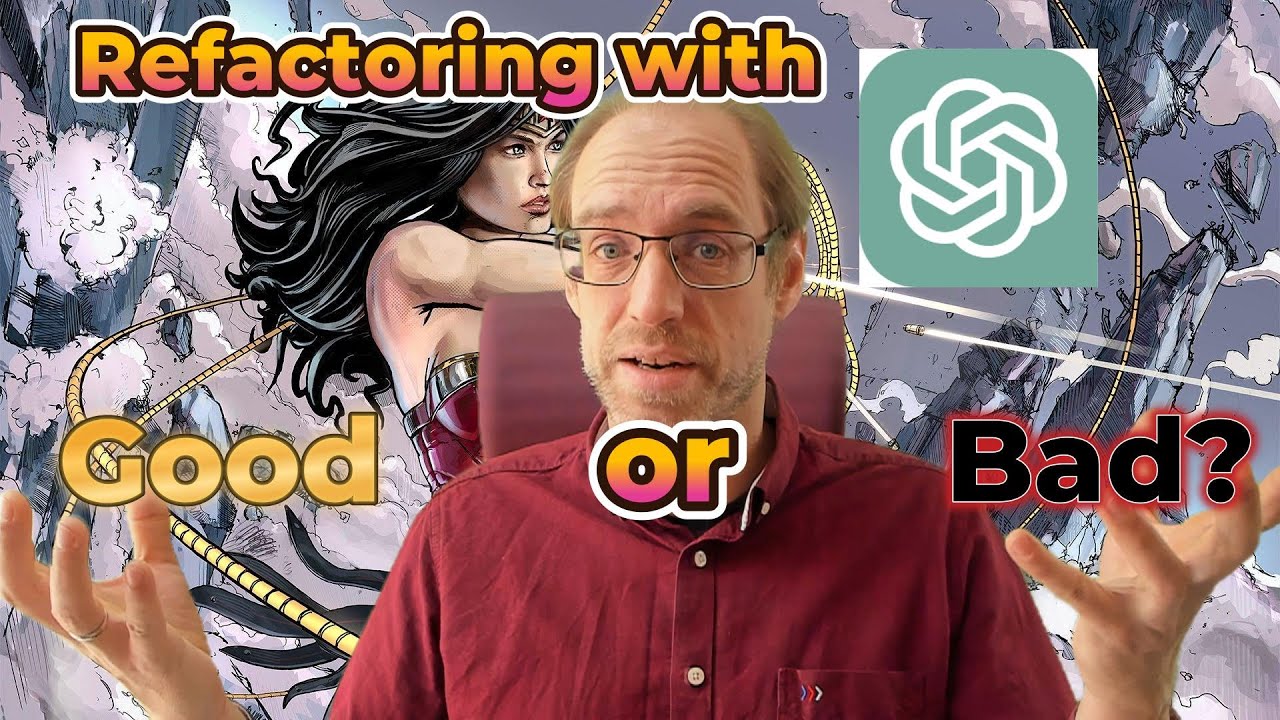
AI-Powered Refactoring: Elevating Code Quality
In our previous adventure into the realm of competitive programming, we witnessed the raw potential and undeniable challenges of melding AI with code strategy. A promising journey met with an unexpected stumble against a seemingly simple adversary. However, every developer knows that true growth lies in iteration—refining not just the code we write but the very way we approach its crafting.
Welcome back to Frankly Developing. I'm your host, Frank, and today, we’re not just going to fix a bug; we’re embarking on a journey to transform our code using AI as our guide and mentor. This article will delve into the art of AI-assisted code improvement, showing how it helps us to refactor our code and enhance overall quality. Together, we’ll dissect the reasoning behind each line of code, understand the principles of clean coding, and witness firsthand the impact of AI in improving code quality.
This isn’t just about beating a game boss; it’s about mastering the craft, setting new standards, and pushing the boundaries of what our code can achieve. Whether you're here to sharpen your programming skills, are curious about AI’s role in code refactoring, or simply love a good coding challenge, you're in the right place. Join me as we redefine what it means to code with quality.
In our previous video, which I've linked here, we used AI to get code that performed the input parsing, parsed all data, provided the necessary information, and then selected actions that seemed best. We also evaluated scores to determine which actions were better than others. Now, let’s ask the AI to suggest some refactoring approaches.
Upon doing so, we received excellent suggestions from the AI:
- Separate Game Logic from Action Strategy: This makes perfect sense and is something we’ll pursue.
- Improve Decision-Making Using a Strategy Pattern: Introducing design patterns will enhance the code’s structure.
- Enhance Action Simulation: While not a true refactoring as it changes functionality, it’s something to consider separately.
- Use Enums and Structs for Directions and Actions: This addresses Primitive Obsession, which is prevalent in our current codebase.
- Implement More Sophisticated Action Selection: Again, not purely refactoring but relevant for future improvements.
- Optimize Data Structures and Algorithms for Performance: Currently unnecessary as performance isn’t an issue, but good timing in the future.
As you can see, AI-assisted refactoring comes with its good and bad suggestions. Some are strong and endorseable, while others don't fit the refactoring criteria as they modify functionality.
We’ll start by addressing Primitive Obsession and introduce enums and structs. I even have a detailed video on this topic. We’ll look at this based on the AI’s suggestion. If you like this sort of content, please like the video and subscribe to the channel.
Here’s what the AI generated to rid the code of Primitive Obsession:
// Enum for direction
enum Direction (
NORTH, SOUTH, EAST, WEST
)
// Struct for Action
struct Action (
string ActionType;
int UnitIndex;
Direction MoveDirection;
Direction BuildDirection;
public Action(string actionType, int unitIndex, Direction move, Direction build) {
ActionType = actionType;
UnitIndex = unitIndex;
MoveDirection = move;
BuildDirection = build;
)
public override string ToString() (
return $"{ActionType) (UnitIndex) (MoveDirection) (BuildDirection)";
}
}
// Extension to convert direction to coordinate
public static class DirectionExtensions (
public static Coordinate ToCoordinate(this Direction direction) {
switch(direction) {
// Map enum to coordinate
)
}
}
Firstly, there’s an enum for direction and a struct for Action. The direction enum is quite straightforward. Instead of a struct, a record could be used, which would simplify the code. Changes to the parsing process also reflect the new design.
The AI grapples a bit with null-checks and fallback actions, generating some unnecessary comments like:
// Return the first action
return actions.First();
This comment is superfluous and doesn’t add value.
When refactoring, ensure you check the outputs rigorously. Our goal was to maintain functionality, meaning the refactoring should not alter how the code behaves.
Conclusively, using AI for code refactoring:
- Can generate boilerplate code that might need manual tweaks.
- Often includes non-value-adding comments.
- Requires human oversight to adjust and ensure the generated code is fit for purpose.
Thank you for reading, and I hope you find joy in developing your skills!
Keywords
- AI-assisted code improvement
- Competitive programming
- Refactoring
- Primitive Obsession
- Clean coding
- Design patterns
- Strategy pattern
- Code quality
FAQ
What is AI-assisted code improvement?
AI-assisted code improvement is the use of artificial intelligence to help identify potential enhancements and refactorings in code, ultimately improving its quality and maintainability.
Why is separating game logic from action strategy important?
Separating game logic from action strategy helps in making the code more modular, easier to reason about, and more maintainable. It allows each part of the code to focus on a single responsibility.
What is a primitive obsession?
Primitive Obsession is a code smell where primitive data types (like strings and integers) are used to represent domain ideas instead of using classes or structs. This can lead to less readable and more error-prone code.
How does the strategy pattern help in decision-making?
The strategy pattern allows for defining a family of algorithms or strategies and makes them interchangeable. This pattern enables choosing an algorithm’s strategy at runtime, thereby improving flexibility and reuse of code.
Do AI-generated comments add value?
AI-generated comments often do not add significant value and can sometimes be superfluous. It is essential to review and refine comments to ensure they add meaningful insights.
Should all AI suggestions be followed?
Not necessarily. AI suggestions should be critically evaluated. While some can be highly beneficial, others might require adjustment or may not be relevant to the current context.