Build A Complete Fullstack banking app with Spring Boot, AI and Reactjs 1
Education
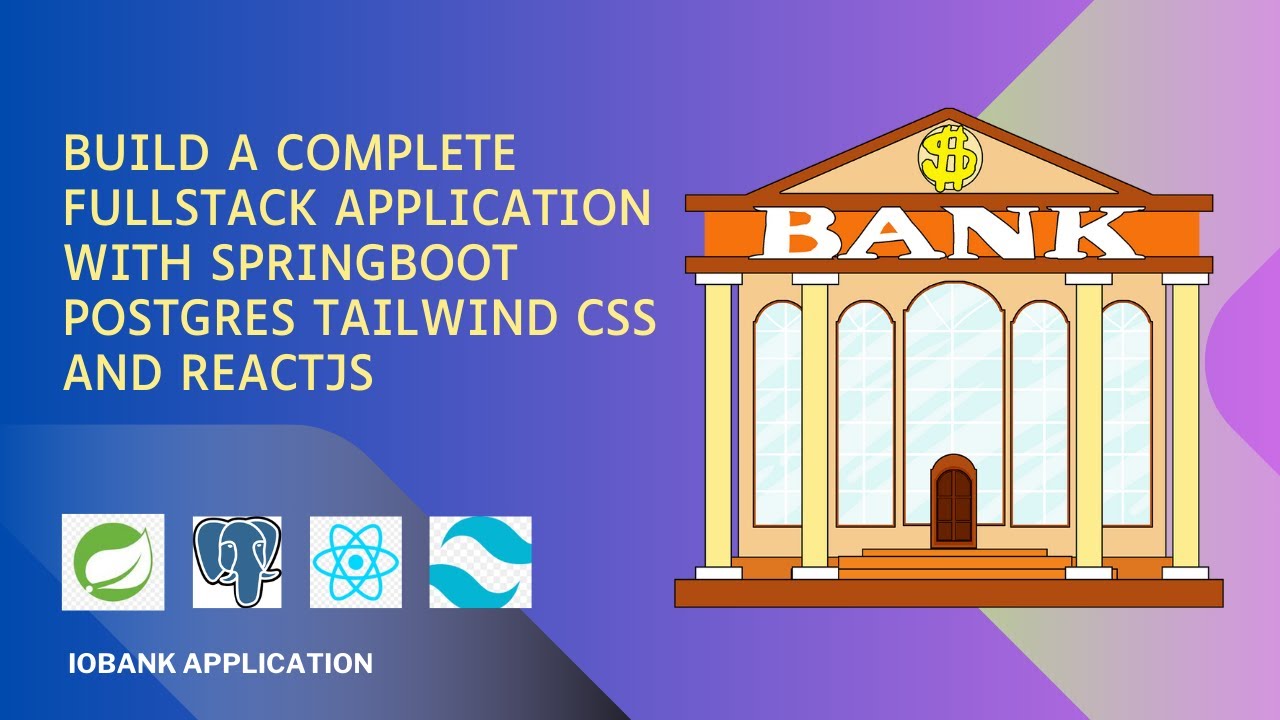
Introduction
In this tutorial, we will develop a full-stack banking application using Spring Boot for the backend and React.js for the client interface. The project will be divided into two main parts: the backend development and the frontend development. The backend will be implemented with Spring Boot and PostgreSQL, while the frontend will utilize React.js along with CSS for styling.
Until we get to the client application development, we will be utilizing Postman and TestF for testing all the functionalities we implement within our server application. For this backend development, we'll be using IntelliJ IDEA as our IDE of choice. Although I'm partial to IntelliJ, feel free to use any IDE you prefer. Additionally, we will install a couple of plugins, beginning with TabNine, an AI code completion tool that will help generate some of the code, while we'll hand-code other segments ourselves to avoid inefficiencies caused by what is known as "hallucinations" in AI—this is a term for inaccurate statements generated by AI models.
Along with the TabNine plugin, we'll also install JPA Buddy. This tool facilitates database management by suggesting repository methods that Hibernate can utilize to query our database.
Application Overview
To give you an idea of what we will be developing, let’s take a brief look at the finished application. The dashboard of a logged-in user displays a list of their accounts. For instance, if we consider a user named Maxwell, he might have both USD and NGN accounts, with each account corresponding to its own account number. Users can switch between currency types, create new accounts, view their transactions, and see card details associated with their accounts.
A notable feature will include real-time currency conversion between different pairs, such as USD and NGN, utilizing a public currency API to fetch accurate exchange rates.
Getting Started with Spring Boot
To kick off our application, we'll initiate a Spring Boot project using the Spring Initializr website. We will fill in essential details such as Group ID, Artifact ID, and select various dependencies including Spring Web, Spring Data JPA, PostgreSQL, and Spring Security for authentication.
Once the project is set up, we will define our entities, relationships, and repositories. Our application will consist of several entities including User, Account, Card, and Transaction models, and we will establish relationships between them.
Implementing Business Logic
After defining our models and repositories, we will begin implementing the business logic. We will build functionalities like user registration, authentication, creating accounts, and conducting transactions. We will also implement necessary validations to ensure the integrity of our data, such as ensuring that users cannot create multiple accounts of the same type or transferring amounts that exceed their balances.
Future Steps: Security and Data Handling
In regard to security, we will implement Spring Security to manage user authentication effectively. Each user will have a unique token that they will use to authenticate their requests.
To ensure accurate data fetching and minimizing unauthorized access, we will configure secure endpoints appropriately. We also plan to utilize real-time exchange rate services, fetching data periodically from the currency API, allowing conversions between various currencies in real time.
Conclusion
By the end of this implementation, you will have a robust backend application that not only manages banking functionalities but is also secured and efficient. The final part of this series will focus on building the client application using React.js, which will interact with our Spring Boot backend seamlessly.
With that, let’s get started!
Keywords
Spring Boot, PostgreSQL, React.js, Banking Application, Backend Development, Frontend Development, TabNine, JPA Buddy, REST API, JWT, Currency Conversion, Security, User Authentication.
FAQ
Q1: What technologies will be used in this banking application?
A1: The application will use Spring Boot for the backend, PostgreSQL for the database, and React.js for the frontend.
Q2: How will data be tested during development?
A2: We will utilize Postman and TestF to test various functionalities implemented in the backend before completing the frontend.
Q3: Why should I use TabNine and JPA Buddy?
A3: TabNine helps with code completions and suggestions using AI, while JPA Buddy streamlines database interaction by generating appropriate repository methods.
Q4: What security measures will be in place for the application?
A4: Spring Security will be implemented to manage user authentication and secure various endpoints.
Q5: Can I customize account types in the application?
A5: Yes, the application allows users to create different accounts with unique currency types.