Build a Real-Time Audio to Text App with Whisper AI & Groq API in Next.js | AI Projects
Education
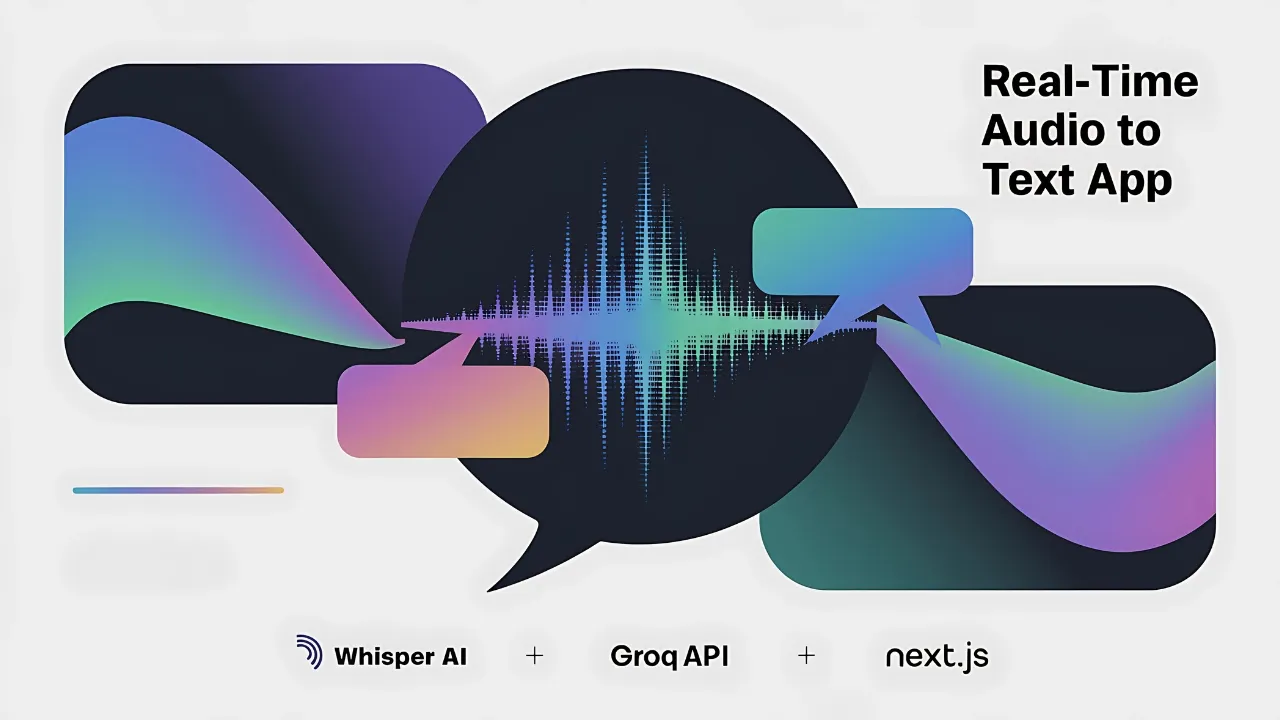
Introduction
Welcome to this tutorial where we will be building a real-time audio-to-text application using Whisper AI and the Groq API, all powered by Next.js. In this article, we’ll walk through the key steps to set up the application where users can upload audio files, visualize audio in real-time, and receive text transcriptions. Let's dive in!
Project Overview
Our app will feature an interface that allows users to upload audio files or record directly. After uploading or recording, users can visualize the audio, and with a click of the button, the transcription will appear on the screen. We’ll employ Whisper AI for transcription and Groq API for interaction with audio data.
Tools & Technologies
We will be using the following technologies:
- Next.js: A React framework for building server-rendered applications.
- TypeScript: A superset of JavaScript that provides type safety.
- Tailwind CSS: A utility-first CSS framework for styling.
- Whisper AI: OpenAI's model for accurate audio transcription.
- Groq API: For processing and handling audio data.
Setting Up Your Next.js Project
Start by setting up your Next.js project:
Initialize your project in the terminal:
npx create-next-app@latest transcript-text cd transcript-text
Install necessary packages:
npm install @groq/sdk @lucid/react
Create a
.env.local
file in your project’s root directory to securely store your API key.
Configuring the API
- Generate your API key from the Groq website and paste it into the
.env.local
file. - In your project, create a new folder named
API
, and inside it create another folder calledtranscript
. Here you’ll manage the transcription of audio files in a file calledroute.ts
.
Building the Audio Context
To handle audio functionalities, create an audioContext.ts
file. This will manage the audio context that helps in visualizing audio waves and processing live audio streams.
Recording Audio
For live recording, create a custom hook in useHasBrowser.ts
. This ensures that audio functionalities are only executed in the browser environment.
Audio Visualization Component
Create an AudioVisualizer.tsx
component to take audio data and render it on the screen with animated bars. This provides a real-time visual feedback of the audio being played.
Handling Transcription Results
Create a TranscriptionResult.tsx
component to display the transcription text clearly. It includes functionalities for copying the text to the clipboard.
Creating the Audio Uploader Component
The AudioUploader.tsx
component will allow users to upload files or record audio. It features:
- File validation and state management.
- Loading indicators while transcription processes.
- Sections to display the uploaded audio, visualizations, and the transcription results.
User Interface
Design the user interface using Tailwind CSS ensuring it’s responsive across devices, presenting a modern look and feel.
Testing The Application
After building the components, test out the application by uploading an audio file. You’ll see the real-time visualization, and upon clicking the transcript button, you’ll receive the written text
Everything should work smoothly, and users can now effortlessly upload audio files, visualize them in real-time, and receive accurate transcriptions.
Conclusion
Congratulations on building your real-time audio-to-text application! You now have a fully functional app that combines audio processing, visualization, and transcription.
Keywords
- Next.js
- Whisper AI
- Groq API
- Audio Transcription
- Real-Time Visualization
- TypeScript
- Tailwind CSS
FAQ
Q1: What is the purpose of Whisper AI in this project?
A1: Whisper AI is utilized for accurate audio-to-text transcription in the application.
Q2: How does the audio visualizer work?
A2: The audio visualizer component renders animated bars that reflect the frequency data of the audio being played in real-time.
Q3: Can users record audio live in this app?
A3: Yes, users have the option to record audio live and visualize it directly.
Q4: What technologies are used in this project?
A4: This project uses Next.js, TypeScript, Tailwind CSS, Whisper AI, and Groq API.
Q5: Is this application responsive for mobile devices?
A5: Yes, the UI is designed to be responsive and works smoothly on both desktop and mobile devices.