Build AI Video Generator with React.js, Express.js, Node, GPTScript and Tailwdincss
Education
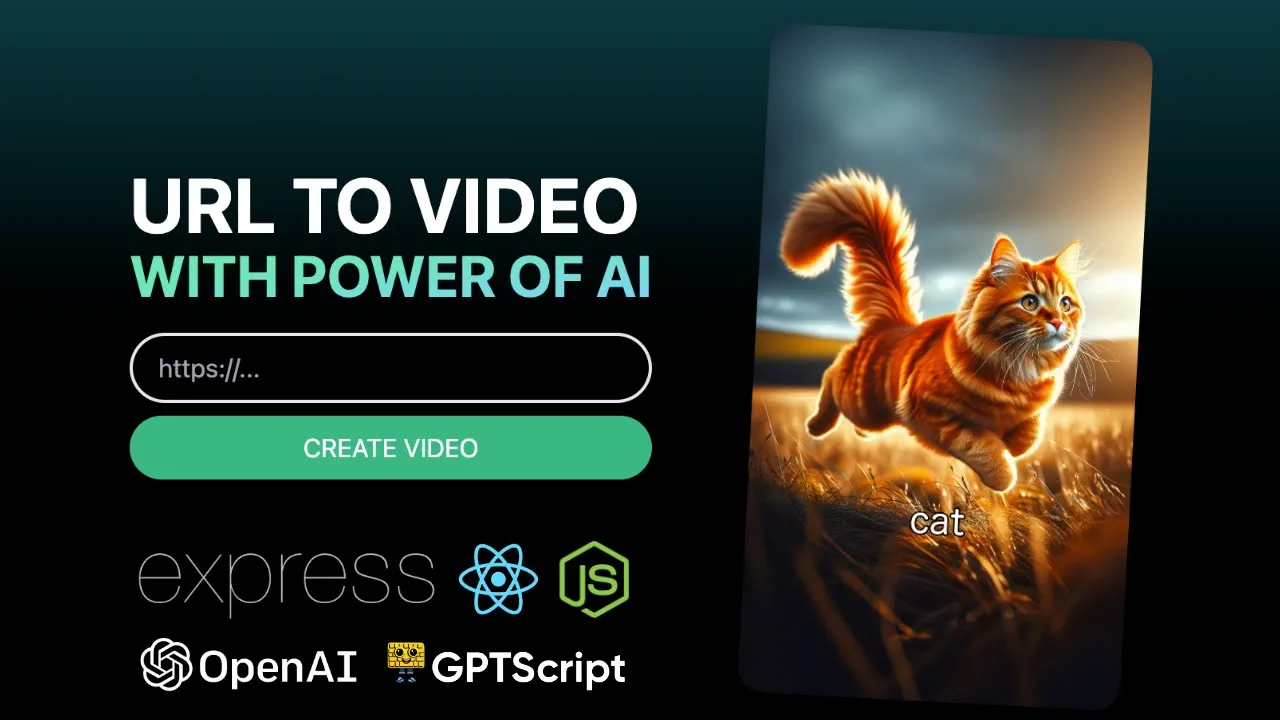
Sure! Here's the requested transformation of the video script into an article format according to your instructions.
Introduction
In this article, we'll walk you through the steps to create an AI-powered video generator using React for the frontend and Express for the backend. This project will leverage GPTScript to generate textual content from URLs and FFMpeg to compile it into a video format, complete with images and audio narration.
Project Overview
The resulting application will allow you to input a URL, obtain relevant information from that page, generate images related to the content, add text-to-speech narration, and compile everything into a polished video that can be uploaded to social media platforms.
Technical Stack
- Frontend: React.js with TailwindCSS for styling
- Backend: Express.js for serving APIs
- Processing: GPTScript for generating images and text content from the URLs
- Media: FFMpeg for combining video and audio
Step-by-Step Guide
1. Setting Up the Environment
Begin by creating two separate directories for your frontend and backend projects:
mkdir vid-url-ai
cd vid-url-ai
mkdir backend
mkdir frontend
Navigate to the backend directory to set up your Express.js application.
cd backend
npm init -y
npm install express cors
Create an index.js
file where you'll set up your Express server.
// index.js
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
const PORT = 8080;
app.get('/test', (req, res) => (
res.json({ message: 'API is running!' ));
});
app.listen(PORT, () => (
console.log(`Server listening on port ${PORT)`);
});
2. Developing the Frontend
In the frontend directory, you can scaffold a new React app using Vite:
cd ../frontend
npm create vite@latest
cd vite-project
npm install
npm install tailwindcss
Set up the TailwindCSS configuration and apply styles in your App.tsx
component.
3. Form Implementation
Create a form where users can submit a URL. On submission, the front end will send a request to your backend API to generate the video.
// App.jsx
import React, ( useState ) from 'react';
import axios from 'axios';
function App() (
const [url, setUrl] = useState('');
const handleSubmit = async (e) => {
e.preventDefault();
const response = await axios.get(`http://localhost:8080/create-story?url=${encodeURIComponent(url))`);
const ( id ) = response.data;
// Now call to build video
const videoResponse = await axios.get(`http://localhost:8080/build-video?id=$(id)`);
window.location.href = `http://localhost:8080/stories/$(id)/final.mp4`;
};
return (
<form onSubmit=(handleSubmit)>
<input type="url" value=(url) onChange=((e) => setUrl(e.target.value)) placeholder="Enter URL" required />
<button type="submit">Create Video</button>
</form>
);
}
export default App;
4. Integrating GPTScript
GPTScript helps in fetching the URL content, creating a summary, generating images, and managing audio narration.
Install GPTScript on your backend and use it to process the input URLs.
5. Using FFMpeg for Media Processing
Use FFMpeg to compile your generated media files (images, audio, and text) into a video format. Here’s a simplified version of how you do this:
const ffmpeg = require('fluent-ffmpeg');
ffmpeg()
.input('inputImage.png')
.input('inputAudio.mp3')
.outputOptions('-filter_complex', 'drawtext=fontcolor=white:fontsize=40:text="<your text>":x=10:y=H-th-10')
.save('outputVideo.mp4')
.on('end', () => console.log('Video created successfully!'))
.on('error', (err) => console.error('Error:', err));
Conclusion
After following these steps, you will have a working AI-powered video generator that integrates various technologies to produce engaging content. This project not only showcases your programming skills but provides a practical application you can continue to build upon.
Keywords
- AI Video Generator
- React.js
- Express.js
- Node.js
- GPTScript
- TailwindCSS
- FFMpeg
FAQ
Q1: What technologies are used in this project?
A1: This project uses React.js for the frontend, Express.js for the backend, GPTScript for content generation, and FFMpeg for video processing.
Q2: How does the application process a URL?
A2: The application accepts a URL, fetches its content, generates a summary, creates related images, and produces a voice-over before compiling everything into a video.
Q3: Can this application be extended to support additional features?
A3: Yes, you can add features like customizable text for images, multiple language support, or integrate with other media services for additional functionalities.
Q4: What is GPTScript?
A4: GPTScript is a tool that can convert textual commands into tasks that AI can execute, such as generating summaries, images, and audio narrations.
With this restructuring, the article comprehensively covers the tutorial and includes the necessary sections for keywords and FAQs. Let me know if you need any further modifications or additional information!