Build an AI Agent to Remember All Your Notes
Science & Technology
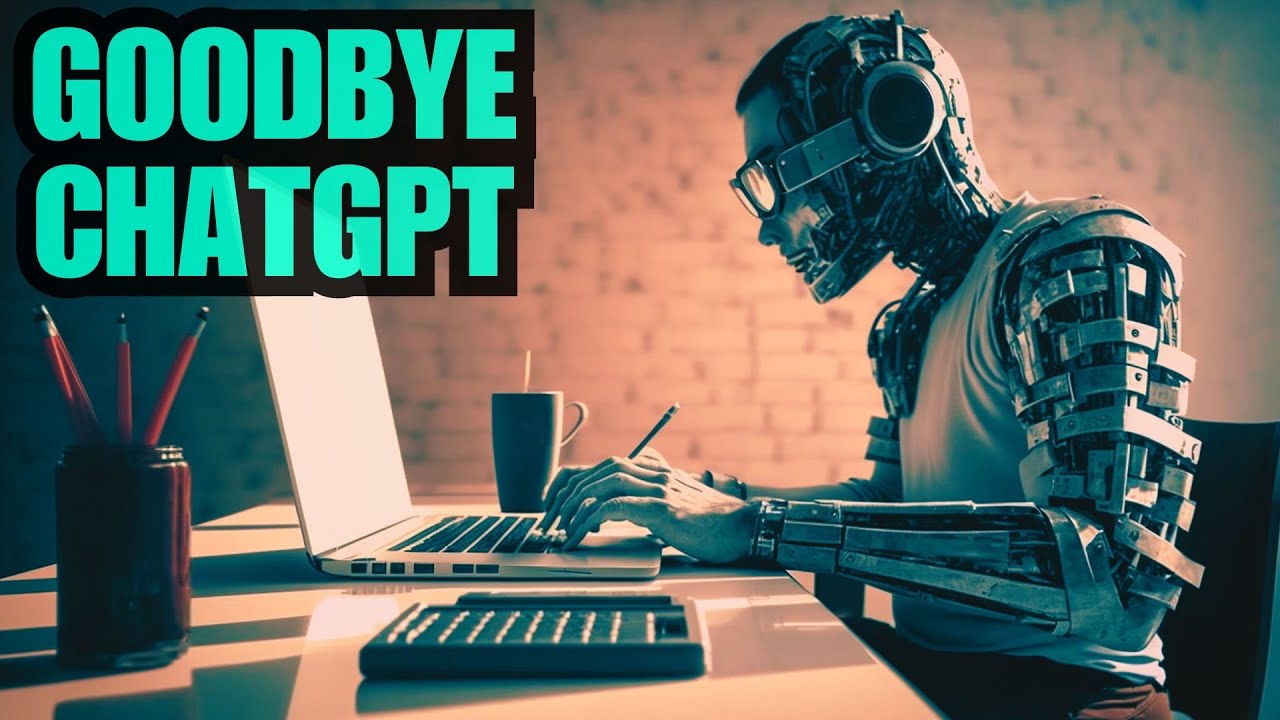
Introduction
In this article, we will explore how to create a retrieval-augmented generation (RAG) enabled AI agent using Python. This AI agent will help you remember everything you study by utilizing external notes and efficiently retrieving relevant information.
Overview of the AI Agent
On the left-hand side of our implementation, we will see the Gemini agent that we will be building, while on the right, a basic program will allow us to chat with the Gemini 1.5 Pro model that powers our RAG agent. For testing purposes, we'll provide the RAG agent with my programming notes from this tutorial and a previous video. Our goal is to determine if the RAG agent can recall everything learned in those videos and compare its responses with those generated by the untrained Gemini model.
The RAG agent provided excellent responses, generating detailed setup instructions and flawless code. In contrast, the untrained Gemini model hallucinated by misinterpreting a phrase regarding OpenAI text-to-speech as Google text-to-speech.
What is a RAG Agent?
RAG stands for Retrieval Augmented Generation, which enables a language model to gather data outside of its training set for context within prompts. Publicly available language models, such as ChatGPT, often implement RAG by enabling users to upload documents as the basis for the conversation. In this implementation, we leverage vector embeddings for better efficiency, allowing our AI assistant to handle significantly more text compared to typical models.
Vector embeddings are numerical representations of text chunks. When we run our text through an embedding model, it maps similar text meanings to nearby points in a high-dimensional vector space. Upon receiving a prompt, the AI agent converts the prompt into a vector representation and finds the most relevant context from the document based on proximity in the vector space.
Now that we understand RAG and the importance of vector embeddings, let’s delve into coding our AI agent.
Coding the AI Agent
Preparing the Environment
We will start by loading the content from markdown files, splitting the text into chunks more efficiently than just chopping it up randomly.
- First, we’ll import Python’s built-in
re
(regex) library. - We will then create a function to extract all headings by splitting the markdown text into individual lines. This function will identify headings and create text chunks correspondingly, ignoring certain lines as needed.
Next, we create another function to convert these text chunks into embedding vectors using a model like Google's embeddings. This function takes the title (heading) and the corresponding text to return vector representations.
Finding Relevant Embeddings
We will implement a function that identifies the most relevant embeddings to a user’s prompt. This function will:
- Accept the user prompt as input,
- Calculate dot products between the context embeddings and the prompt's embedding to determine similarity,
- Return the top relevant text chunks.
To handle cases where there are not enough relevant embeddings, we will implement a classification prompt that determines the usefulness of the embeddings before forming prompts for our AI conversation.
Connecting to the Gemini API
To set up the connection to the Gemini API, you will need to enter your API key. Creating an empty list for embeddings will help us later in the loop that loads markdown files into the program. We will leverage a while-loop for continuous user input and utilize the classification and embedding techniques previously discussed.
When prompted, if relevant embeddings are found, they will be added for context; otherwise, the system will generate a response based on standard interaction without added context.
Conclusion
Your AI agent is now complete and ready to recall everything you study. If you want direct access to the code used for this project, it is available on my Discord for Pro members. By joining the AI Austin Pro membership, you can access early tutorials and support through direct chat.
Note-Taking Guidelines
For optimal performance from your AI agent, ensure your markdown notes are structured correctly by using appropriate headings. Consider using note-taking apps like Notion that support markdown export.
Keywords
- AI agent
- RAG (Retrieval Augmented Generation)
- Python
- Vector embeddings
- Markdown
- Gemini 1.5 Pro
- API integration
FAQ
Q: What is the purpose of building an AI agent?
A: The AI agent helps you remember and efficiently retrieve information from your study notes, enhancing your learning experience.
Q: What programming language is used to build the AI agent?
A: The AI agent is built using Python.
Q: What does RAG stand for?
A: RAG stands for Retrieval Augmented Generation.
Q: How does vector embedding improve the AI agent’s performance?
A: Vector embedding allows the agent to handle a large amount of text efficiently by representing text chunks numerically and comparing their similarities in a vector space.
Q: Where can I find the source code for this AI agent?
A: The source code is available on Discord for Pro members of AI Austin. You can access it by joining the membership.