Build and Deploy a Full Stack AI SaaS | Next JS 13, DrizzleORM, OpenAI, Stripe, TypeScript, Tailwind
People & Blogs
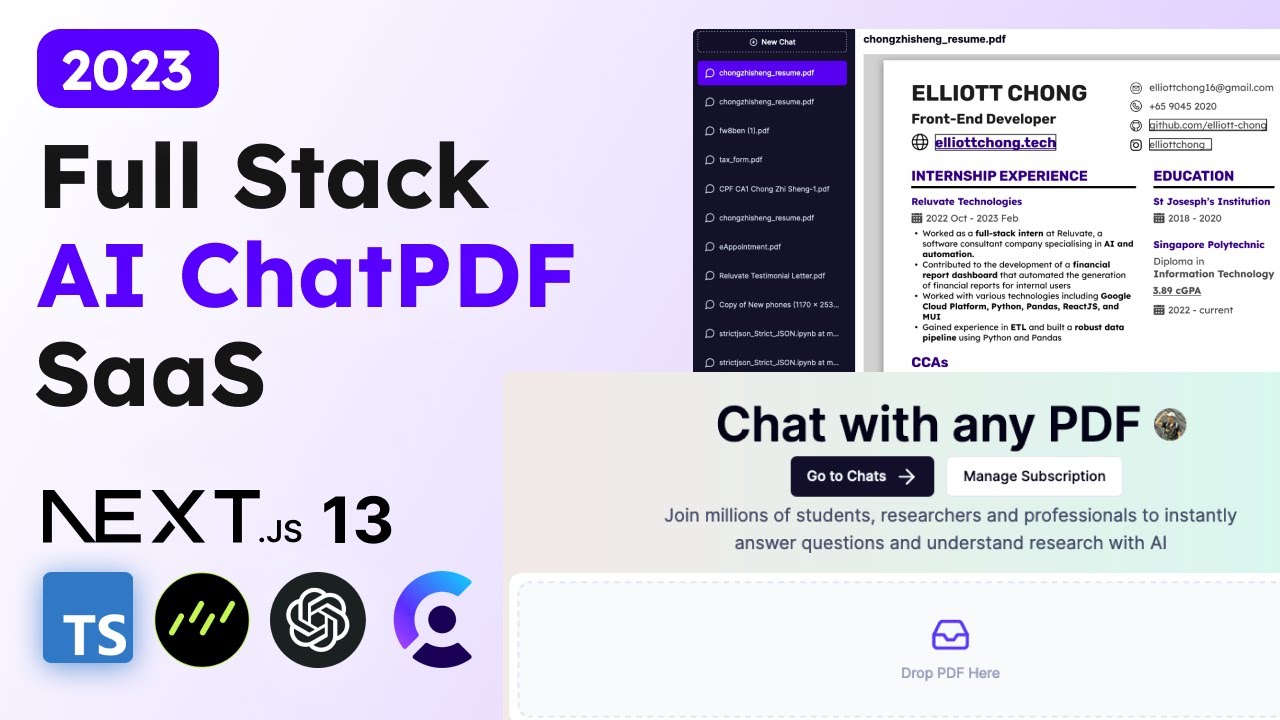
Introduction
In this article, we'll walk through the process of building a full stack AI Software as a Service (SaaS) application that allows users to interact with PDF documents using OpenAI's GPT model. We will utilize Next.js 13, DrizzleORM for database management, Stripe for payment processing, and Tailwind CSS for styling. By the end of this tutorial, you will be able to deploy your own SaaS product that can handle PDF interactions!
Step 1: Project Overview
We will build a chat application where users can upload PDF files and interact with them using AI-powered chatbots. The application architecture is as follows:
- Frontend: Built with Next.js, using TypeScript for type safety and Tailwind CSS for styling.
- Backend: Using DrizzleORM to manage database operations and OpenAI's API to power the chat features.
- Payments: Integrating Stripe for subscription management.
Step 2: Setting Up the Project
Initialize a Next.js Project:
npx create-next-app@latest --typescript chat-pdf
This command initializes a new Next.js project with TypeScript.
Install Required Dependencies: Use npm or yarn to install the required packages:
npm install drizzle-orm typeorm stripe lucid-react @vercel/ai npm install tailwindcss postcss autoprefixer
Set Up Tailwind CSS: Follow the Tailwind CSS installation guide to integrate it into your Next.js project.
Step 3: Building the Chat Application
3.1 User Authentication
Set up Clerk for user authentication. This process involves:
- Creating a Clerk account.
- Integrating Clerk's authentication methods in our application.
3.2 User Subscription
Integrate Stripe for subscription management. This allows users to upgrade to a pro plan.
- Set up Stripe and initialize API keys in your environment variables.
- Create models in DrizzleORM for user subscriptions and implement the subscription logic.
3.3 Drag-and-Drop PDF Uploading
Implement a drag-and-drop interface for users to upload PDFs.
- Use the React Dropzone library to handle file inputs.
- The uploaded PDF is stored in AWS S3, where it can be processed.
3.4 PDF Interaction using OpenAI
Once a PDF is uploaded, users can interact with it through chat with the AI.
- The PDF content is extracted and split into smaller chunks.
- OpenAI's GPT model is queried with the relevant PDF context.
3.5 Chat Component
- Create a chat component that listens for user input and sends messages to the AI.
- Display chat history with the AI responses.
3.6 Deployment
Finally, deploy your application using Vercel.
- Create a Vercel account and link it to your GitHub repository where the code is stored.
- Configure environment variables in Vercel for production.
By following these steps, you’ll have your very own AI-enabled PDF chatting application deployed and ready for users!
Keywords
- Full Stack Development
- Next.js
- DrizzleORM
- OpenAI GPT
- Stripe Subscription
- TypeScript
- Tailwind CSS
- AWS S3
- SaaS Application
FAQ
1. What technologies are used to build this application?
The application uses Next.js for the frontend, DrizzleORM for database management, OpenAI for AI functionalities, Stripe for user payments, and Tailwind CSS for styling.
2. How do users interact with PDF files?
Users can upload PDFs via a drag-and-drop interface. The content of the PDF is then extracted, and users can ask questions about the document using the chat interface.
3. How is user authentication handled?
User authentication is managed using Clerk, which provides a simple integration for handling user login and registration.
4. How are payments processed?
Payments are handled by Stripe, allowing users to upgrade to a pro subscription for access to premium features.
5. How can I deploy the application?
The application can be deployed on Vercel by connecting your GitHub repository and setting up necessary environment variables.