Building a Speech-Driven NPC with GPT/LLM: A Step-by-Step Tutorial"
Entertainment
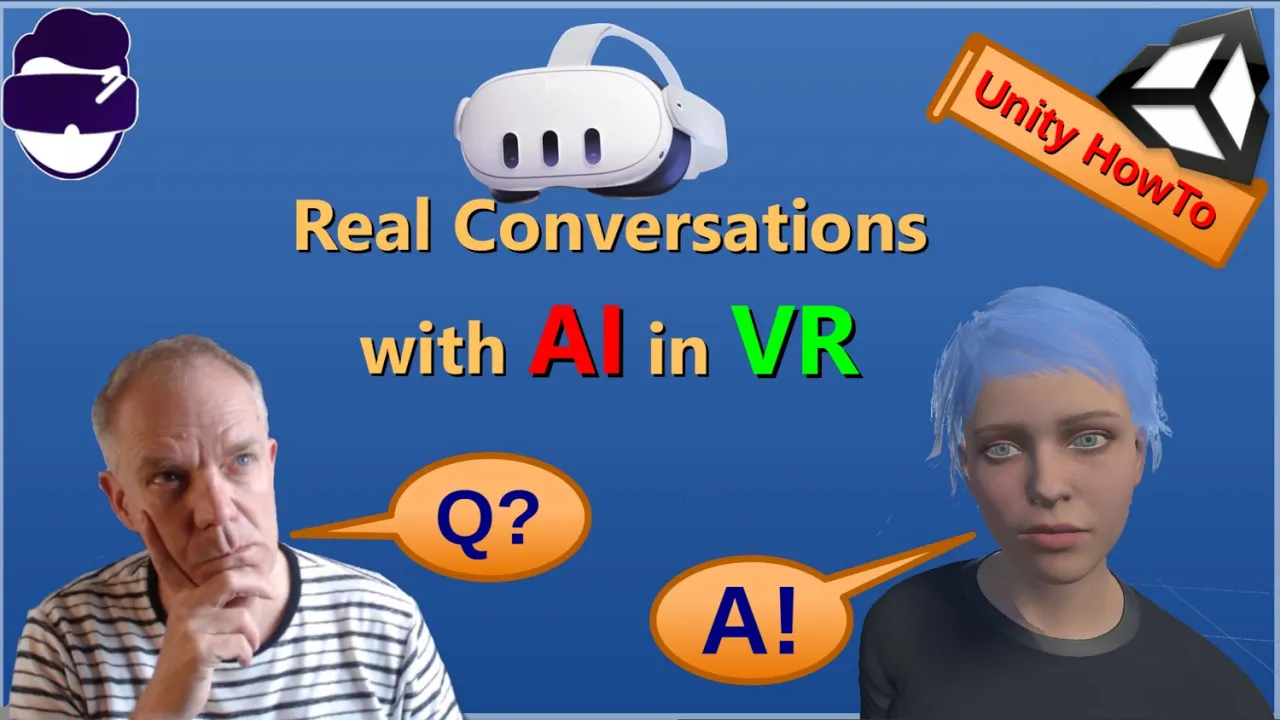
Introduction
Welcome to this extensive guide where we'll cover how to build an intelligent NPC (Non-Player Character) leveraging Large Language Models (LLMs), Generative Pre-trained Transformers (GPTs), and speech interfaces with Unity. This tutorial will step you through the process, including speech-to-text (STT), text-to-speech (TTS), and setting up an LLM. The project will be available on my GitHub repository, github.com/sdigitalplusplus
.
Introduction
In this tutorial, we'll explore creating an interactive NPC using Unity and modern AI technologies. We will-
- Implement a speech interface using LLMs and GPTs.
- Build from scratch within Unity.
- Provide code, assets, and more details available for download on GitHub.
So, grab your favorite drink and join me on this exciting coding journey!
Supporting Tools and Services
Speechify
Speechify has generously supported this project by offering free credits. With over 40 English-speaking voices, we can use their API within our text-to-speech module. Learn how Speechify simplifies reading and enhances API-driven projects.
Architectural Components
Large Language Models (LLMs)
LLMs like GPTs are used for generating text responses based on input. We will chain STT, LLM, and TTS to facilitate AI-driven conversations.
AI Workflow
- Speech to Text (STT) – Converts voice input to text.
- LLM Processing – LLM generates text responses based on STT output.
- Text to Speech (TTS) – Converts LLM text response back to voice.
REST API Integration
We’ll utilize REST APIs to keep our project flexible and vendor-agnostic. This approach will allow us to quickly adapt to any new developments in AI technologies.
Cloud vs. Local AI
Running AI models locally is impractical for high-quality outputs due to large memory and power requirements. We’ll leverage cloud services to handle AI processing efficiently.
API Products
Various vendors provide APIs for LLMs, STT, and TTS:
LLM API Providers
- OpenAI
- Anthropic
- Microsoft
- Google Cloud
- Meta (LLaMA)
Speech to Text API Providers
- Hugging Face
- RapidAPI
- AWS Transcribe
- Microsoft Azure
- Google Cloud
Text to Speech API Providers
- Speechify
- Hugging Face
- RapidAPI
Animating the NPC
To enhance the experience, we will animate the NPC using blend shapes and the Unity Animator. Specifically, we will use the free UL Lip Sync
Unity package to animate real-time lip-sync.
Building the Project
Setting up Unity
- Create a new Unity project and set up basic scenes.
- Import necessary SDKs and packages for VR and networking (if applicable).
Scripting AI Components
Text to Speech (TTS)
- Integrate Speechify's TTS API in Unity.
- Serialize fields for API keys and voice selection.
- Define a public function to convert text to speech.
public class TTSSimba : MonoBehaviour (
// Code to integrate Speechify's TTS API
)
Large Language Model (LLM)
- Use Grok Cloud’s REST API for LLM integration.
- Serialize fields for API keys and model selection.
- Create a public function to process text input using LLM and return text through TTS.
public class LLMGrok : MonoBehaviour (
// Code to integrate Grok Cloud's LLM API
)
Speech to Text (STT)
- Utilize Hugging Face’s API for the STT module.
- Serialize fields for API keys.
- Create functions to record audio, convert it to a stream, and process it via STT API.
public class STTHuggingFace : MonoBehaviour (
// Code to integrate Hugging Face's STT API
)
NPC Animation
- Import 3D NPC models (with blend shapes for lip movements).
- Add components for lip sync.
- Utilize Unity’s Animator for idle and talking animations.
Combining Components
- Ensure smooth integration between STT, LLM, and TTS.
- Implement event-driven architecture for click-to-talk mechanism in VR.
- Optimize lip sync animations for realistic interactions.
Testing & Finalization
- Once scripts and animations are set, test the interaction flow.
- Debug and resolve any issues in audio, animations, or API calls.
Wrapping Up
Deploy and commit the project to GitHub to share and collaborate. Stay updated with AI and Unity developments to continuously enhance the NPC capabilities.
Keywords
Here are some keywords summarizing the article:
- LLM (Large Language Model)
- GPT (Generative Pre-trained Transformer)
- Unity
- Speech to Text (STT)
- Text to Speech (TTS)
- NPC (Non-Player Character)
- Blend Shapes
- Lip Sync
- Animator
- REST API
FAQ
Q1: What is an LLM (Large Language Model)? A: LLMs are AI models trained to understand and generate human-like text based on input data.
Q2: Why use REST APIs for AI integration? A: REST APIs keep the project flexible and vendor-agnostic, allowing easy adaptation to new AI developments.
Q3: Why not run AI models locally? A: Running AI models locally is impractical due to high power and memory requirements; cloud services provide efficient processing.
Q4: How do you animate the NPC? A: We use blend shapes for lip-sync and Unity's Animator for idle and talking animations.
Q5: What tools and services were used? A: We used Speechify for Text to Speech, Grok Cloud for the LLM, and Hugging Face for Speech to Text.
Q6: How can I access the project?
A: You can access the project on the GitHub repository: github.com/sdigitalplusplus
.
I hope you enjoyed this detailed guide on building an intelligent NPC, and I look forward to seeing you next time!