Building an AI-powered Audiobook Generator (AI Product-a-thon)
Education
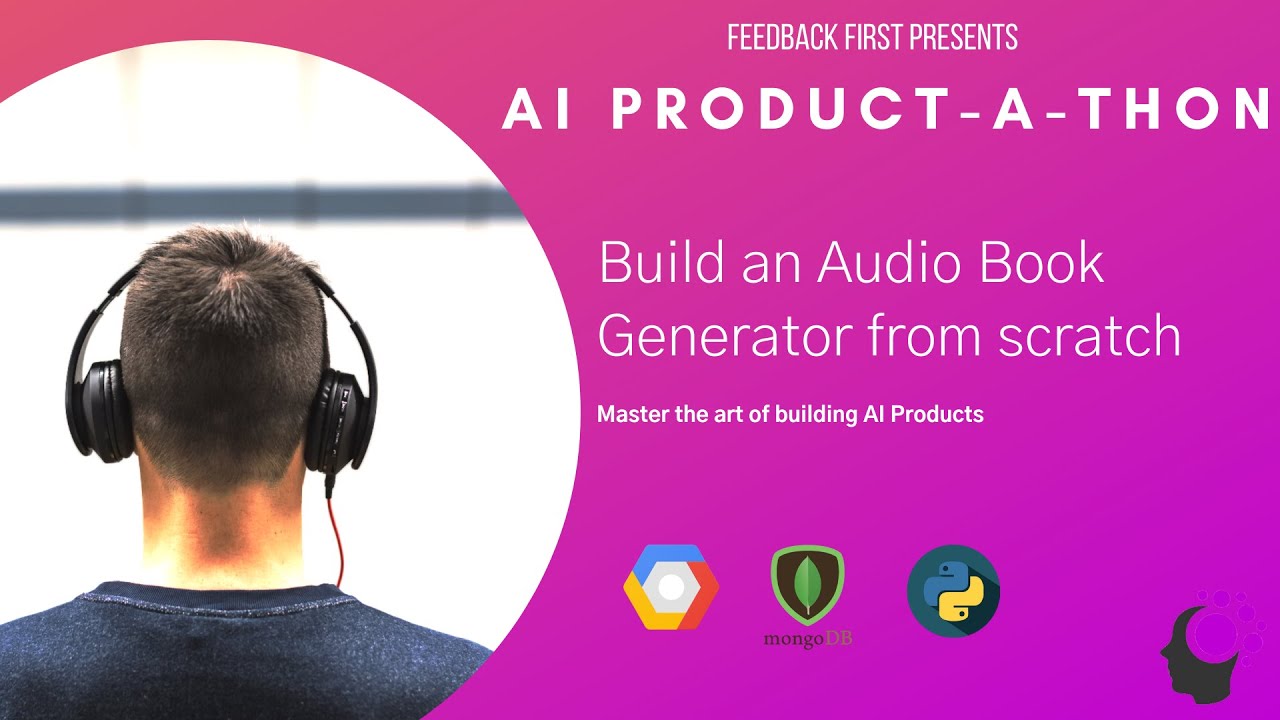
Building an AI-powered Audiobook Generator (AI Product-a-thon)
Introduction
Hello everyone, welcome to this product line session about building an audiobook generator from scratch. In previous sessions, we explored how to build an article recommendation engine, an AI-powered learning management system (LMS), and a video search engine. Today, we'll dive into how you can generate an audiobook from your PDFs using machine learning, particularly AI-powered APIs. We will utilize tools like Google Cloud, technologies like MongoDB, Python, and see how all of these come together to build this AI product.
The Problem Statement
Going through a PDF, scrolling from top to bottom and navigating through all those pages, can be a tiresome experience. Imagine you have a PDF and wish to consume its content while on a walk—how do you do that? Currently, the only way to read a PDF is by being in front of a laptop or mobile device, which isn’t always convenient. Wouldn't it be fantastic if an assistant could read out the PDF for you, summarizing the key points?
The Solution
Our solution is simple: create an audiobook out of a PDF. Here's a breakdown of how the process works:
- Upload a PDF.
- Choose an accent and voice (male/female and various accents).
- Generate the audiobook using Google's Text-to-Speech API.
Implementation Steps
Uploading PDF and Selecting Preferences:
- We will implement the feature where a user can upload a PDF and choose their preferred accent and gender for the voice. We’ll use Google Cloud’s wide range of accents like American, British, and Indian.
Cloud Storage and Vision API:
- First, upload the PDF to Google Cloud Storage, which is cheaper and supports both input and output storage of file data. Then, use the Computer Vision API for Optical Character Recognition (OCR) to extract text content from the PDF.
Text-to-Speech API:
- Use the extracted text to generate an audiobook via Google's Text-to-Speech API. The API takes the text input along with the chosen accent and voice and synthesizes it into speech.
Search Engine and Notifications:
- Store the audiobook in Google Cloud Storage and notify the user via email about the audiobook URL once processing is complete. Additionally, build a search engine to index the audiobook content.
Tech Stack
- Cloud Storage & Hosting: Google Cloud Storage, Google Cloud App Engine
- Database: MongoDB, MySQL
- Programming Language: Python
- Frontend: Bootstrap, HTML, CSS, JavaScript, jQuery
Detailed Implementation
Import Necessary Libraries:
from google.cloud import storage, vision, texttospeech import flask
Setting Up Google Cloud Credentials:
- Create a service account in Google Cloud IAM with necessary permissions, and download the credentials in a JSON file.
os.environ['GOOGLE_APPLICATION_CREDENTIALS'] = 'path/to/credentials.json'
Enable APIs:
- Enable both Vision and Text-to-Speech APIs in the Google Cloud Console.
Upload PDF and Process:
- Create a Flask application for uploading PDFs and capturing user preferences.
@app.route('/upload', methods=['POST']) def upload_pdf(): # handle file upload # store metadata in MongoDB # upload to Google Cloud Storage # extract text using Vision API
Text Extraction using Vision API:
def extract_text_from_pdf(pdf_path): client = vision.ImageAnnotatorClient() with io.open(pdf_path, 'rb') as f: content = f.read() image = vision.Image(content=content) response = client.document_text_detection(image=image) return response.full_text_annotation.text
Generate Audiobook using Text-to-Speech API:
def generate_audio(text, language_code, gender): client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text=text) voice = texttospeech.VoiceSelectionParams( language_code=language_code, ssml_gender=gender ) audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) response = client.synthesize_speech( input=synthesis_input, voice=voice, audio_config=audio_config ) with open('output.mp3', 'wb') as out: out.write(response.audio_content)
Notify User and Provide Audiobook URL:
- Once the audiobook is generated and stored, notify the user via email with the URL for accessing the audiobook.
Conclusion
By combining various Google Cloud services and Python libraries, we've created an efficient and user-friendly audiobook generator. This robust solution is versatile and can be adapted with more features or scaled based on user requirements.
Keywords
- Google Cloud
- Audiobook Generator
- Text-to-Speech API
- Vision API
- Machine Learning
- Python
- MongoDB
- Flask
- OCR
- AI-Powered APIs
FAQ
Q: What libraries are used for this implementation? A: The libraries include Google Cloud's storage, vision, and text-to-speech libraries, as well as Flask for web handling and MongoDB for database.
Q: Why use Google Cloud Storage for storing PDFs and audiobooks? A: Google Cloud Storage is cost-effective and suitable for handling large, unstructured data such as PDFs and audio files. Moreover, Google’s APIs require data to be stored in their cloud storage.
Q: How does the Vision API work for text extraction? A: The Vision API uses Optical Character Recognition (OCR) to process the PDF, extracting text and storing it in a structured format, like a dictionary.
Q: What are the steps to enable Google APIs for this project? A: Navigate to the Google Cloud Console, search for the APIs (Vision and Text-to-Speech), and enable them. Also, set up a service account with the necessary permissions.
Q: Can I choose different accents and voices? A: Yes, Google's Text-to-Speech API supports various accents and genders, allowing you to customize the audiobook’s voice according to your preferences.