Convert Youtube video into Neo4j graph using LangChain|Tutorial:120
Education
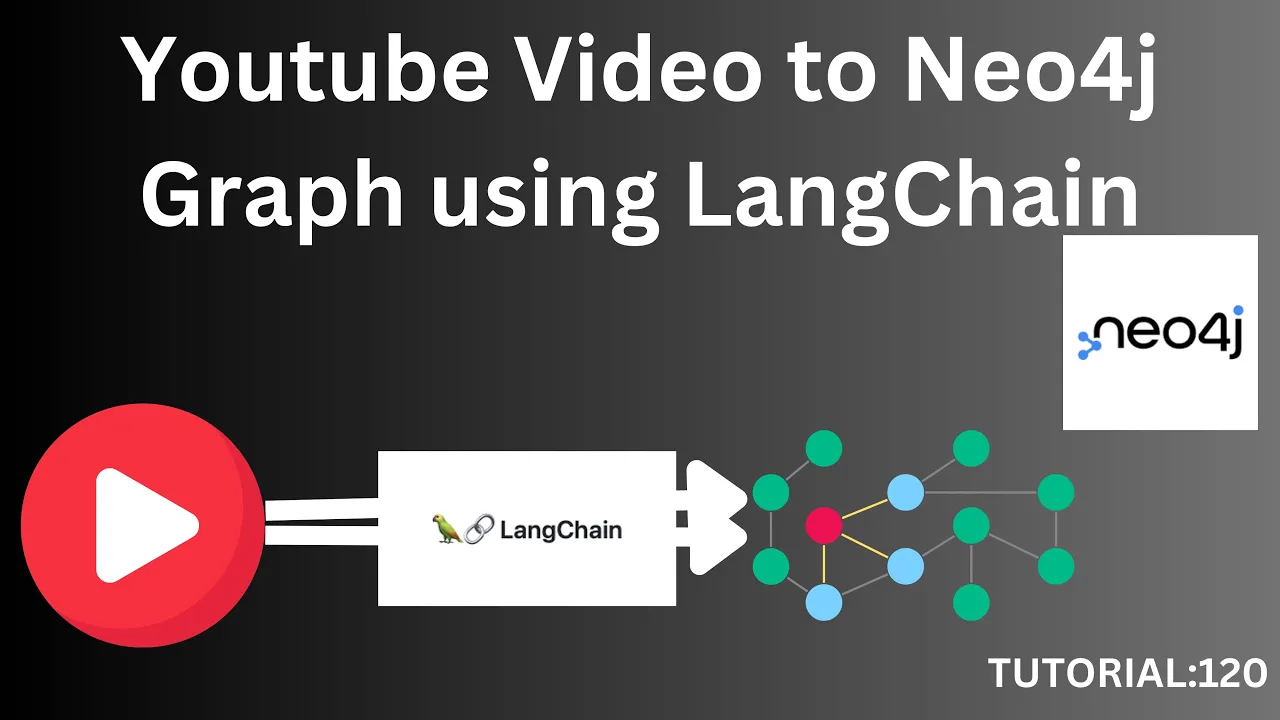
Introduction
In this tutorial, we will explore how to convert a YouTube video into a graph document using LangChain. This involves retrieving the audio transcript from the video, transforming it into a graph document, and then loading it into a Neo4j graph database.
Introduction to Graph Documents
A graph document stores information as nodes and the relationships between these nodes. This method of data storage is valuable for applications involving connection and relationships, particularly in the domain of generative AI and large language models (LLMs). If you're unfamiliar with Neo4j, it's recommended to check out our Neo4j playlist available on the channel for a deeper understanding.
Prerequisites
Before diving into the coding part, it’s essential to ensure you have the necessary tools and libraries installed. The following libraries must be imported in your Python environment:
from langchain.document_loaders import YouTubeLoader
from langchain.experimental.graph import GraphTransformer
from langchain.openai import ChatOpenAI
from langchain.community.neo4j import Neo4j
Step 1: Extract Audio Transcript from YouTube Video
The first step is to extract the transcript from a selected YouTube video. Let’s begin by defining a function that takes a YouTube URL and returns the audio transcript.
def get_youtube_transcript(url):
loader = YouTubeLoader.from_youtube_url(url)
docs = loader.load()
return docs
The YouTubeLoader
allows us to pull audio from the video efficiently. After retrieving the transcript, we can print or return it for further processing.
Step 2: Transform Transcript into Graph Document
Next, we need to convert the extracted transcript into a graph document using the GraphTransformer
. This is where LangChain’s capabilities come into play as it provides a seamless way to transform text documents into graph structures.
def convert_to_graph(docs):
llm_transformer = GraphTransformer()
graph_documents = llm_transformer.convert_to_graph_documents(docs)
return graph_documents
Step 3: Loading to Neo4j Graph Database
To load the graph document into a Neo4j graph database, set up your connection details and create the graph. You need the NE4 URI, username, and password, which can be obtained from the Neo4j website.
import os
from dotenv import load_dotenv
load_dotenv()
neo4j_uri = os.getenv("NEO4J_URI")
neo4j_username = os.getenv("NEO4J_USERNAME")
neo4j_password = os.getenv("NEO4J_PASSWORD")
graph = Neo4j(uri=neo4j_uri, username=neo4j_username, password=neo4j_password)
graph.add_graph_documents(graph_documents)
After executing this code, your YouTube video content will be successfully transformed and loaded into the Neo4j graph environment.
Conclusion
By following these steps, we can effectively convert YouTube video content into nodes and relationships within a Neo4j graph. This approach provides valuable insights into the connectivity within the video's transcript. Make sure to like, comment, and subscribe to help support the channel and stay updated with future tutorials!
Keywords
- YouTube video
- Neo4j
- LangChain
- Graph document
- Transcript
- Audio loader
- Large Language Models
FAQ
Q1: What is LangChain?
A1: LangChain is a powerful library used for processing and transforming language data, primarily leveraging large language models (LLMs).
Q2: How can I access the Neo4j database?
A2: You can create a free account at the Neo4j website and set up a sample database. Connection details are provided, including URI, username, and password.
Q3: Can this method handle lengthy videos?
A3: Yes, LangChain can extract audio transcripts from longer videos as well, making it suitable for various types of content.
Q4: Do I need to understand graph databases to use this tutorial?
A4: While having a basic understanding of graph databases like Neo4j is helpful, the tutorial provides the necessary steps for conversion and loading data.
Q5: Is there any additional setup required for using LangChain?
A5: Ensure you have Python and the necessary libraries installed in your environment, including the environment variable management library for handling your Neo4j credentials.