Create a ChatGPT Voice Assistant in 8 Minutes (Python Tutorial)
Science & Technology
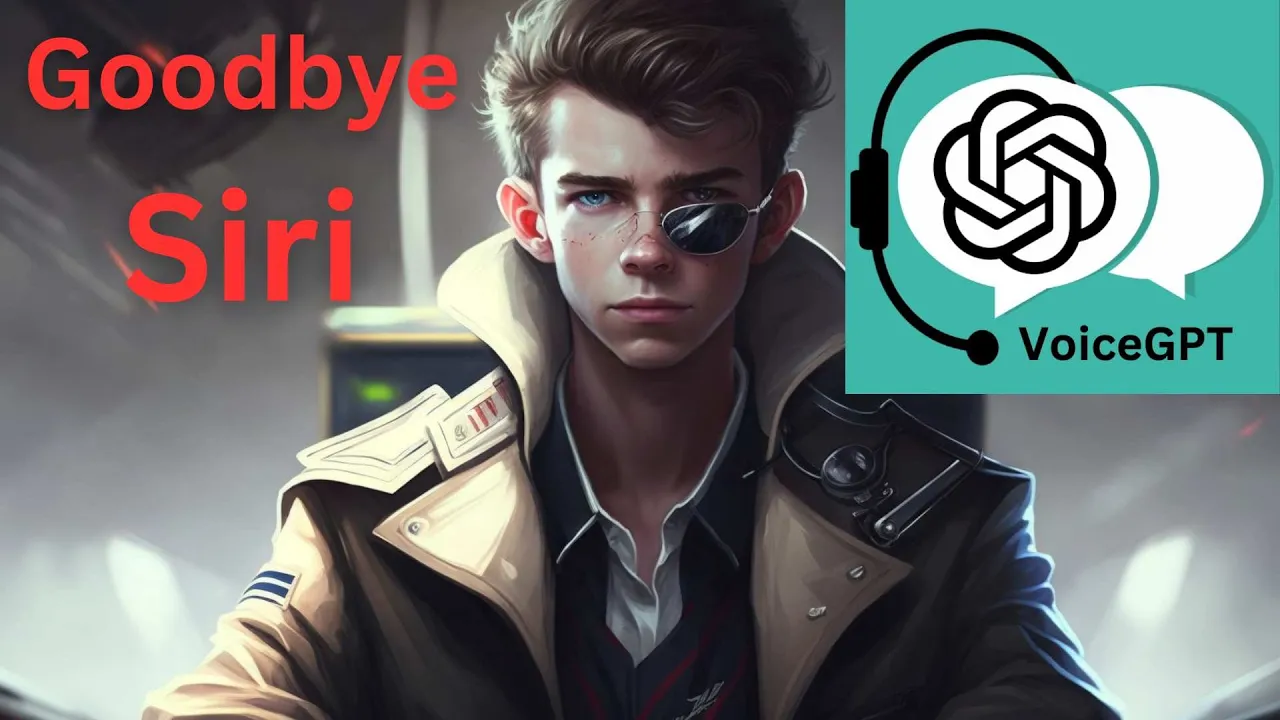
Introduction
Ever since ChatGPT was released, I’ve had the constant urge to ask Siri a question that only ChatGPT can answer. Instead, I decided to create a GPT-3 powered voice assistant with Python. In this tutorial, I'm going to show you how you can do the same. At the end, I will give some ideas on how to take this program and make it into a Software as a Service (SaaS) business.
We will be diving into the code step-by-step and explaining what each line of code is doing, so even if you're new to Python and AI, you’ll still be able to follow along.
Step-by-Step Guide
Step 1: Import Necessary Libraries
First, open your Python environment and create a new Python file. Begin by importing the openai
library, which will allow us to access the GPT-3 API. In addition, import the pyttsx3
library to convert text to speech, as well as the speech_recognition
library to transcribe audio to text.
import openai
import pyttsx3
import speech_recognition as sr
Step 2: Set Up OpenAI API Key
Next, set up your OpenAI API key. Replace the dummy API key with your own OpenAI API key, which you can get for free from the OpenAI website.
openai.api_key = 'your_openai_api_key'
Step 3: Set Up Text-to-Speech Engine
Create an instance of the text-to-speech engine and store it in a variable.
engine = pyttsx3.init()
Step 4: Transcribe Voice Commands
Define a function to transcribe voice commands into text using the speech_recognition
library.
def transcribe_audio_to_text(filename):
recognizer = sr.Recognizer()
with sr.AudioFile(filename) as source:
audio_data = recognizer.record(source)
try:
return recognizer.recognize_google(audio_data)
except sr.UnknownValueError:
return "Could not understand the audio"
Step 5: Generate GPT-3 Responses
Create a function to generate responses from the GPT-3 API.
def generate_response(prompt):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=4000,
temperature=0.5
)
return response.choices[0].text.strip()
Step 6: Text-to-Speech Function
Define a function to convert text to speech.
def speak_text(text):
engine.say(text)
engine.runAndWait()
Step 7: Main Function Logic
Structure the logic of the program within a main function, including an infinite loop to keep the assistant running.
def main():
while True:
print("Say 'genius' to ask your question.")
recognizer = sr.Recognizer()
with sr.Microphone() as source:
audio = recognizer.listen(source)
try:
if recognizer.recognize_google(audio).lower() == "genius":
print("Ask your question.")
with sr.Microphone() as source:
audio = recognizer.listen(source)
with open("input.wav", "wb") as f:
f.write(audio.get_wav_data())
text = transcribe_audio_to_text("input.wav")
print(f"You said: (text)")
response = generate_response(text)
print(f"Assistant: (response)")
speak_text(response)
except sr.UnknownValueError:
print("Listening again...")
if __name__ == "__main__":
main()
Conclusion
With these steps, you now have a basic Python-powered GPT-3 voice assistant. You can talk to it and get intelligent responses in real-time.
Keywords
- Python
- OpenAI
- GPT-3
- Voice Assistant
- pyttsx3
- speech_recognition
- Text-to-Speech
FAQ
Q: How do I get an OpenAI API key? A: You can get a free API key by signing up on the OpenAI website.
Q: Why is the pyttsx3 module not found?
A: Ensure that pyttsx3 is installed correctly and is compatible with your Python version. You can install it using pip install pyttsx3
.
Q: How do I deploy this assistant to a website? A: You can use web frameworks like Flask or Django to build a web interface for your assistant and host it on a server.
Q: What does the temperature parameter do in GPT-3? A: The temperature parameter controls the creativity or randomness of the generated text. A lower value makes the output more deterministic.
Q: What if my assistant doesn't recognize my speech?
A: The recognize_google
method may sometimes fail to understand the audio. Ensure your microphone is working and try speaking more clearly.