Create a Large Language Model from Scratch with Python – Tutorial
Education
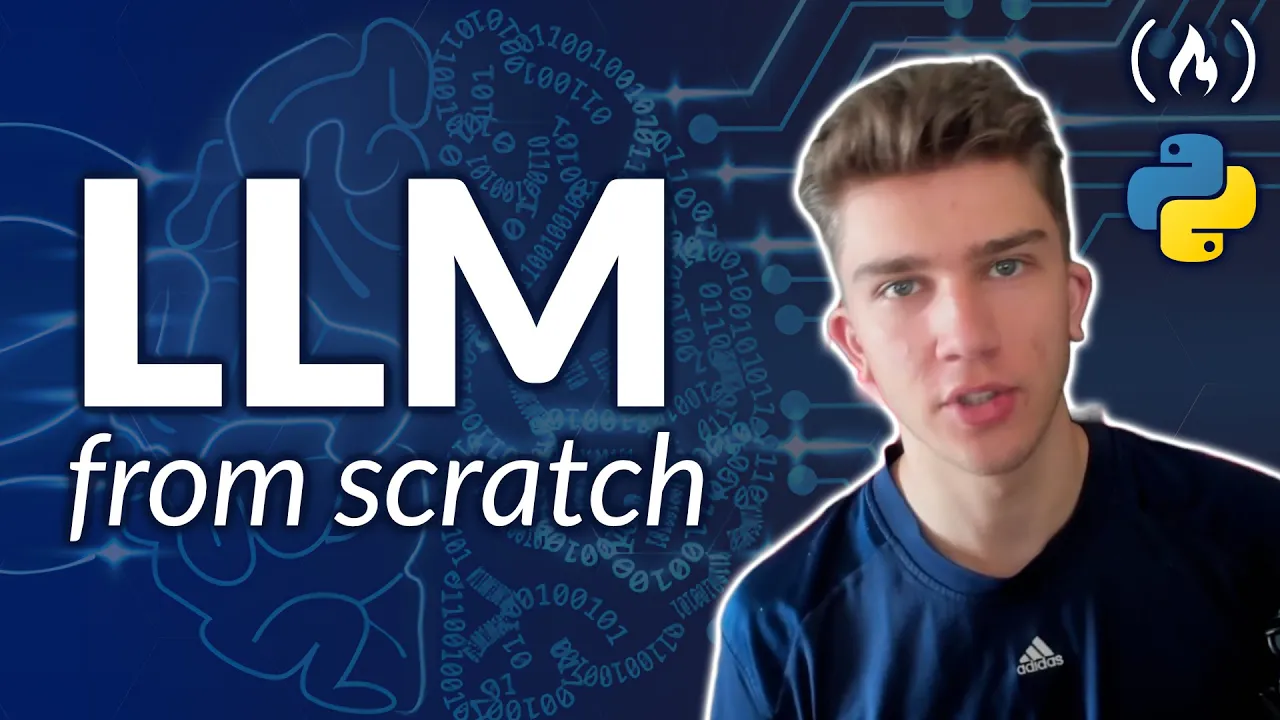
Introduction
In this comprehensive tutorial, we'll explore how to build a large language model from scratch using Python. The course is designed for beginners and advanced users alike, providing insights into data handling, mathematics, and the Transformer architecture that powers state-of-the-art language models like GPT-4. Elia Arledge, the course creator, guides us through the intricacies of language model construction, without assuming any prior experience in advanced mathematics or machine learning concepts.
Introduction to Language Modeling
Welcome to the course on creating large language models (LLMs). This tutorial will go into depth about the fundamental concepts of LLMs, including data handling, mathematical principles, and the Transformers architecture behind models like GPT. We will approach learning in baby steps, gradually building up to more complex concepts.
Course Agenda
- Data handling and preprocessing
- Basic Python and numpy operations
- Building and understanding the architecture of Transformer models
- Training and fine-tuning a language model on large text corpora
- Using tools like SSH for remote computation
Getting Started
To get started, you'll need three months' experience in Python programming. You'll require local computation resources, preferably with cloud computing for more intensive tasks. Ensure that you have around 90GB of storage space for the dataset, and install tools like Anaconda and Jupyter Notebooks for a smooth development environment.
Setting Up the Environment
We'll start by setting up the environment using Anaconda and Jupyter Notebooks. Here's a step-by-step guide:
- Install Anaconda: Follow the installation guide linked in the resources to set up Anaconda.
- Create Virtual Environment: Create a virtual environment to keep your project dependencies isolated.
- Installing Libraries: Install essential libraries like matplotlib, numpy, pylzma, ipykernel, and Jupyter Notebooks.
- Installing PyTorch with CUDA: Utilize PyTorch for GPU acceleration.
Understanding Tensors
In PyTorch, tensors are the primary data structures used for computation. They are analogous to numpy arrays but optimized for GPUs.
- Creating Tensors: Demonstrate how to create tensors and perform basic operations.
- Matrix Multiplication: Explain how to perform matrix multiplication using PyTorch.
Key Concepts and Components
Tokenizers and Encoders
A tokenizer converts text into numerical data by mapping each unique character or word to an integer. We’ll discuss character-level tokenizers, word-level tokenizers, and subword tokenizers.
Train and Validate Split
Explain why training and validation data splits are critical for preventing overfitting and ensuring the model generalizes well to new data.
Transformer Architecture
Multi-Head Attention Models: Explain how multi-head attention works, breaking down the terms keys, queries, and values, and the significance of scaled dot product attention.