Create Beautiful AI Images w/ Dalle-3 API | OpenAI
Education
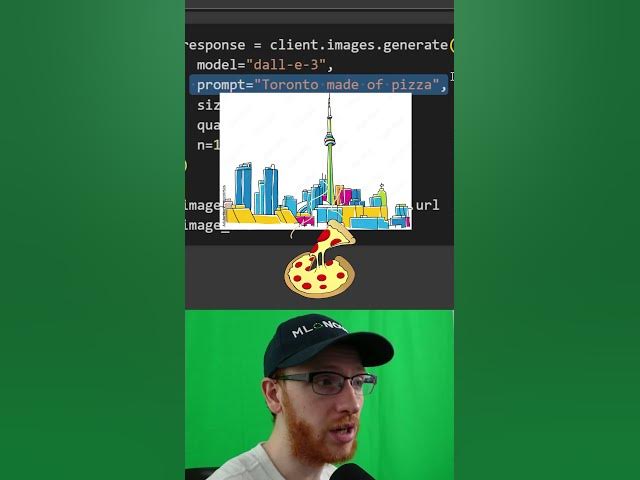
Create Beautiful AI Images w/ Dalle-3 API | OpenAI
Introduction
In this article, I will walk you through the process of creating beautiful AI-generated images using OpenAI's DALL·E 3 API. This guide will cover everything from installing the necessary libraries to generating your custom images.
Step 1: Installing the OpenAI Library
First, you need to install the OpenAI library. Open your terminal and run the following command:
pip install openai
Step 2: Setting Up Your OpenAI Account
To access the OpenAI API, you need to create an account on OpenAI's website. Once registered, navigate to the API section in your account settings and generate a secret key. This key will be essential for authentication purposes.
Step 3: Importing the Library and Initializing the Client
With your secret key in hand, you will now import the OpenAI library and initialize the client. This process allows you to authenticate your requests to the API.
Here's a code snippet to get you started:
import openai
openai.api_key = 'YOUR_SECRET_KEY_HERE'
client = openai.Client(api_key=openai.api_key)
Step 4: Generating an Image
Now that your client is set up, you can generate an image. For this example, I'll prompt the DALL·E 3 API to create an image of Toronto made out of pizza.
response = client.images.generate(
prompt="Toronto made out of pizza"
)
image_url = response['data'][0]['url']
print(image_url)
Step 5: Extracting and Displaying the Image URL
The response will include a URL for the generated image. You can print this URL to see your creation.
print(f'Image URL: (image_url)')
When you open the URL in your browser, you will see your unique image – in this case, a depiction of the CN Tower made out of pizza.
Conclusion
Congratulations! You've successfully created an AI-generated image using the DALL·E 3 API. This method can be tailored to create a wide variety of imaginative and unique images based on your prompts.
Keywords
- OpenAI
- DALL·E 3
- API
- Image Generation
- Python
- Machine Learning
- AI Artwork
FAQ
Q1: How do I install the OpenAI library?
A1: You can install the OpenAI library using pip. Run the command pip install openai
in your terminal.
Q2: Where do I get my OpenAI secret key?
A2: After creating an account on OpenAI's website, go to the API section in your account settings, and generate a secret key.
Q3: How do I initiate a client for the OpenAI API?
A3: After importing the OpenAI library, set your API key with openai.api_key = 'YOUR_SECRET_KEY_HERE'
and then initialize the client using client = openai.Client(api_key=openai.api_key)
.
Q4: What prompt did you use to generate the image of Toronto made out of pizza?
A4: The prompt used was "Toronto made out of pizza"
.
Q5: How can I display the generated image?
A5: Extract the image URL from the API response and open it in your web browser. The URL will be printed when you run the code.