Creating a Quotes Generator with JavaScript For Loops and Math.random() | Coding Project Tutorial
Education
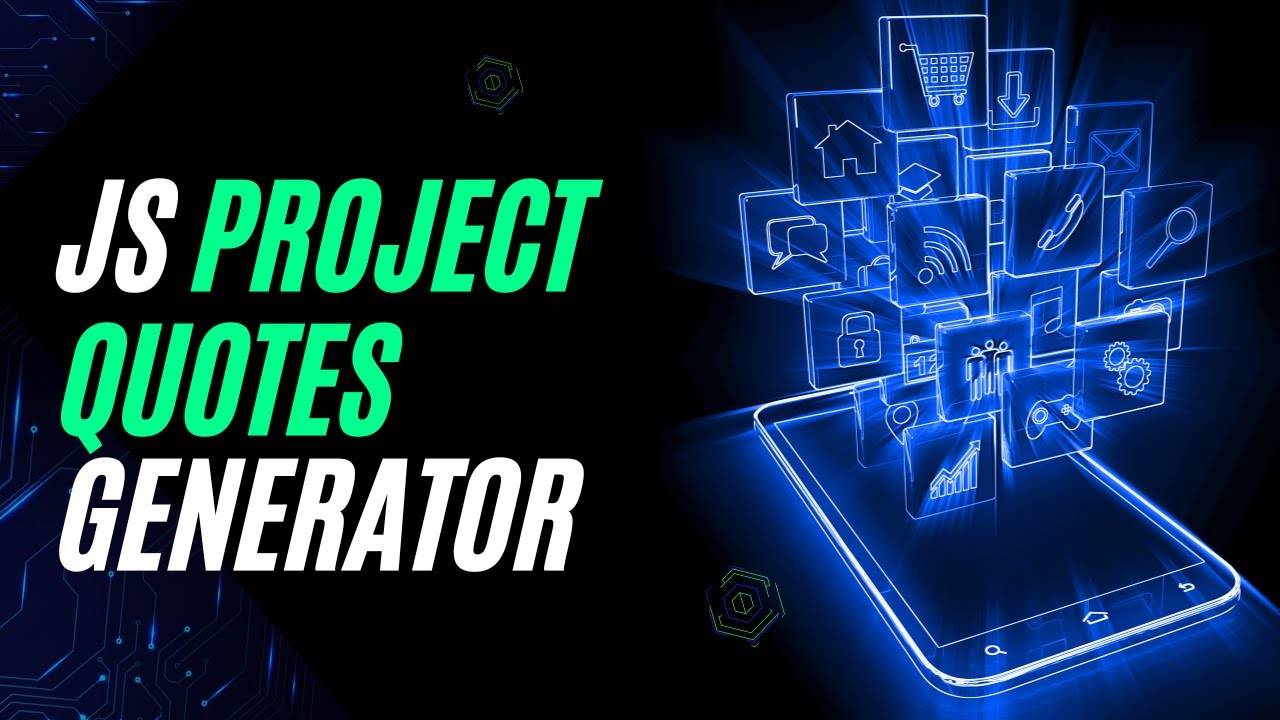
Introduction
In this tutorial, we will explore how to create a simple quotes generator using JavaScript. We will leverage the power of for
loops and the Math.random()
method to generate random quotes from an array. This project will help solidify your understanding of loops, arrays, and DOM manipulation in JavaScript.
Setting Up the Environment
Before we dive into coding, ensure you have a basic HTML file set up to work with. You should have the following structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Quotes Generator</title>
</head>
<body>
<h1>Random Quotes Generator</h1>
<p id="quotes-display"></p>
<button id="generate-quote">Generate Quote</button>
<script src="script.js"></script>
</body>
</html>
In the above code, we have a heading, a paragraph where the quote will be displayed, and a button that will trigger the quote generation.
JavaScript Code Walkthrough
Next, we will write our JavaScript code in a file called script.js
. Start by creating an array of quotes:
const quotes = [
"The only limit to our realization of tomorrow is our doubts of today.",
"Success usually comes to those who are too busy to be looking for it.",
"Don't watch the clock; do what it does. Keep going.",
"The future belongs to those who believe in the beauty of their dreams.",
"Do what you can, with what you have, where you are."
];
Using For Loops
To understand how for
loops work, remember the three essential components:
- Initialization: Here, we declare a variable (e.g.,
let i = 0;
). - Condition: This component checks whether the loop should continue (e.g.,
i < quotes.length
). - Update: This updates the loop variable at the end of each iteration (e.g.,
i++
).
In our case, we don't actually need a for
loop since we will be generating a random number, but it's helpful to know how they function.
Generating Random Quotes
You can use Math.random()
to generate a random number. To get a whole number within the range of our quotes, we need to multiply it by the length of the quotes array and use Math.floor()
to avoid decimal points:
function getRandomQuote() (
const randomIndex = Math.floor(Math.random() * quotes.length);
return quotes[randomIndex];
)
Updating the DOM
Next, we can update the paragraph element to display the generated quote when the button is clicked.
document.getElementById('generate-quote').addEventListener('click', function() (
const quote = getRandomQuote();
document.getElementById('quotes-display').innerHTML = quote;
));
Final Touch
Now that everything is set up, your application will display a random quote from the array each time the button is clicked!
Conclusion
This coding project has helped you understand the use of for
loops, random number generation, and DOM manipulation in JavaScript. Now, with just a button click, you can generate a random quote from the provided array.
Keyword
- JavaScript
- For Loops
- Math.random()
- Quotes Generator
- DOM Manipulation
FAQ
Q1: What is the purpose of Math.random()
?
A1: Math.random()
generates a floating-point number between 0 and 1, which can then be scaled to fit a desired range.
Q2: How do I access elements in an array?
A2: You can access elements in an array using their index, starting from 0. For example, quotes[0]
will give you the first quote in the array.
Q3: What does Math.floor()
do?
A3: Math.floor()
rounds down a floating-point number to the nearest whole integer.
Q4: How do I attach events to HTML elements in JavaScript?
A4: You can attach events using the addEventListener
method. For example, element.addEventListener('click', function() ())
attaches a click event.
Q5: Can I add more quotes in the array?
A5: Yes, you can easily add more quotes by appending new items to the quotes array. For example, quotes.push("New quote here")
.