Domain Name Generator Using LangChain OpenAI Model and Output Parser
Education
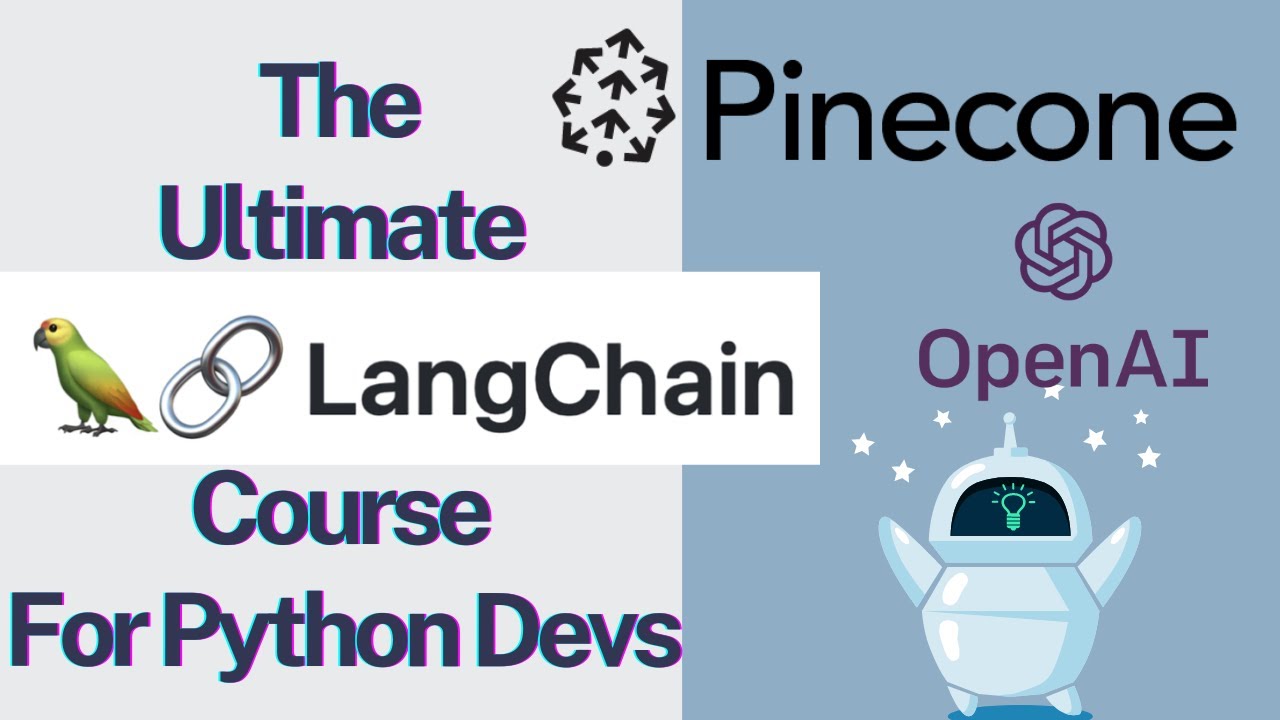
Introduction
In this article, we will walk through the creation of a simple web application that generates domain names based on a given niche using LangChain and OpenAI's models. The application will utilize Flask as a backend server to handle requests and responses, and we will implement an output parser to format the generated data appropriately.
Setting Up the Backend
Step 1: Create the Backend Code
To begin, we'll set up our backend by creating a new file named backend.py
. Here's how we will structure the backend code:
Import Required Libraries
from dotenv import load_dotenv from langchain.llms import OpenAI from langchain.prompts import PromptTemplate from langchain.output_parsers import CommaSeparatedListOutputParser
Load Environment Variables Make sure to load the necessary environment variables using
load_dotenv()
.Initialize the OpenAI Model We define our OpenAI model with specified temperature and model name.
openai_llm = OpenAI(temperature=1, model_name="gpt-3.5-turbo")
Create the Prompt Template We will set up a prompt that instructs the model to generate three
.com
domain names for a particular niche.prompt_template = PromptTemplate("Suggest three .com domain names for my blogging niche: (niche)")
Format the Template and Fetch Results We will implement a function to format the template and interact with the model:
def generate_domain_names(niche): formatted_prompt = prompt_template.format(niche=niche) result = openai_llm(formatted_prompt) return result
Output Parsing Additionally, we introduce an output parser to ensure our response is formatted correctly as a comma-separated list:
output_parser = CommaSeparatedListOutputParser() parsed_output = output_parser.parse(result)
Step 2: Create the Flask Server
Next, we will create another file called server.py
to set up the Flask server:
Import Flask and Initialize the App
from flask import Flask, request, jsonify, render_template app = Flask(__name__)
Create API Endpoints We will create an API route that handles GET requests and returns generated domain names based on user input:
@app.route('/api/chat', methods=['GET']) def chat(): niche = request.args.get('input') response = generate_domain_names(niche) return jsonify(response)
Home Route To display a user interface, we will define a home route that renders an HTML page.
@app.route('/') def home(): return render_template('home.html')
Step 3: Frontend Creation
Now we will create a frontend that allows users to input their niche and receive domain name suggestions.
1. Create HTML Template
In the templates
directory, create a file called home.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Domain Name Generator</title>
</head>
<body>
<div id="container">
<input type="text" id="input" placeholder="Enter your niche">
<button onclick="generateDomain()">Generate Domains</button>
<div id="output"></div>
</div>
<script>
function generateDomain() (
const niche = document.getElementById('input').value;
document.getElementById('output').innerHTML = 'Loading...';
fetch(`/api/chat?input=${encodeURIComponent(niche))`)
.then(response => response.json())
.then(data => (
document.getElementById('output').innerHTML = data.join('<br/>');
));
}
</script>
</body>
</html>
Conclusion
We have successfully built a full-stack web application that combines backend logic with an interactive frontend. By utilizing Flask for the server and LangChain for domain name generation, this application demonstrates how to integrate OpenAI models effectively.
Keywords
- Domain Name Generator
- LangChain
- OpenAI Model
- Flask
- Output Parser
- API
- Web Application
- Frontend
FAQ
Q1: What is the purpose of the domain name generator application?
A1: The application generates .com domain name suggestions based on a niche provided by the user.
Q2: What libraries are used in this project?
A2: The project utilizes Flask for the backend server, LangChain for interacting with OpenAI, and dotenv for managing environment variables.
Q3: How does the frontend communicate with the backend?
A3: The frontend uses the Fetch API to make GET requests to the Flask server, retrieving domain name suggestions based on user input.
Q4: Can I modify the model used for generating domain names?
A4: Yes, you can replace the OpenAI model in the backend with any compatible model from LangChain or modify the parameters as needed.
Q5: Is it necessary to install additional libraries?
A5: Yes, you will need to install libraries such as Flask, LangChain, and optionally dotenv for managing configuration.