Employee Management System Angular 18 | Angular 18 Project
Science & Technology
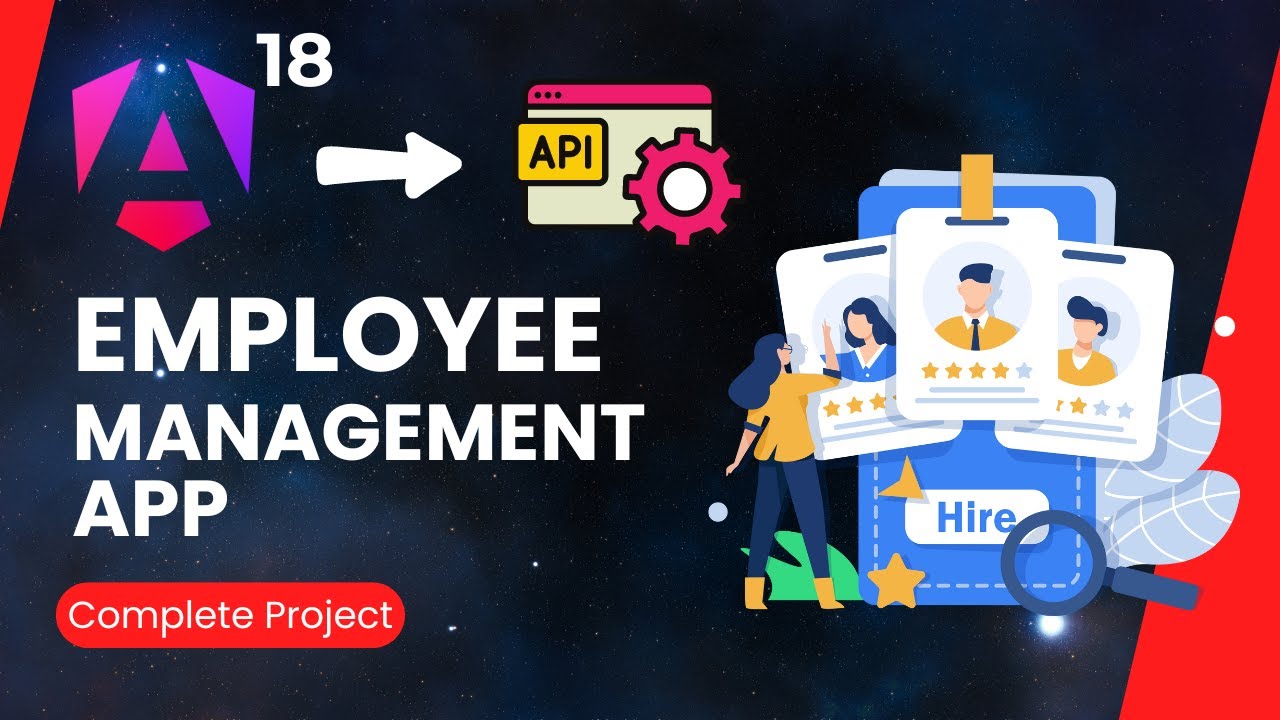
Introduction
Welcome back to Learning Partner! If you're new here, please consider subscribing. In today's walkthrough, we'll be building an Employee Management Application using Angular 18. This project is a common task encountered during technical interviews, and completing it will improve your understanding of CRUD operations, as well as API integrations.
Project Overview
We will develop an Employee Management System, which caters to frequent CRUD operations in Angular applications. This application will primarily consist of four main modules:
- Login Module: A static login page where users can enter their credentials.
- Employee Module: A portal for managing employee CRUD operations. Users can create, edit, list, and delete employees.
- Project Module: This will support the creation and management of various projects within the organization.
- Project Employee Module: This module will link employees to respective projects.
Lastly, we will have a Dashboard that summarizes key statistics like total employees, total projects, recently hired employees, and the latest projects.
Step-by-Step Development Process
Initial Setup
First, we need to create a new Angular project and set up the necessary packages and configurations. Use the following command to create a new Angular project:
ng new employee-management
Once the project is created, install Bootstrap and Font Awesome for styling:
npm install bootstrap font-awesome
Make sure to include them in your angular.json
file under styles.
Creating Components
Next, we'll create several components for each module:
- Login Component: Handles user authentication.
- Employee Component: For managing employee records.
- Project Component: For managing project information.
- Project Employee Component: To manage associations between projects and employees.
- Dashboard Component: Displays all the summary information.
You can generate components using the Angular CLI command:
ng generate component <component-name>
Routing Configuration
Setting up routing is crucial for navigation in our application. We'll configure routes to redirect users to various components. Make sure your main routing module (app-routing.module.ts
) includes paths for login, employee, project, project employee, and dashboard.
Creating Services and API Integration
To interact with our backend APIs, we will define a service (let's call it MasterService
). This service will contain all API calls necessary to interact with employee data, project data, and dashboard data.
Use Angular's HttpClient module to perform GET, POST, PUT, and DELETE operations.
Implementing CRUD Operations
We'll implement the standard CRUD operations for employees, projects, and project employees:
- Create: Users can add a new record.
- Read: Users can view a list of all records.
- Update: Users can edit existing records.
- Delete: Users can remove records as necessary.
We’ll also provide visual feedback and validation during these operations.
Building the Dashboard
In the dashboard component, we will summarize the statistics using a separate endpoint. We will show the total count of employees, projects, and recently added information, enhancing the user experience with quick stats.
Final Thoughts
This Employee Management System is an effective way to showcase your Angular skills. As a hands-on project, it reinforces learning key concepts, such as routing, service use, and dynamic data binding. Once you're comfortable with the system, you can extend its functionality by adding advanced features, improving the UI, or even integrating more complex state management systems.
Keyword
Keywords: Employee Management System, Angular 18, CRUD Operations, API Integration, Login Module, Employee Module, Project Module, Project Employee Module, Dashboard, Reactive Forms, Observables.
FAQ
Q1: What is the primary purpose of the Employee Management System?
A1: The primary purpose is to manage employee records, projects, and their associations effectively using an Angular application.
Q2: What technologies are used in this project?
A2: This project uses Angular 18, Bootstrap for styling, and Font Awesome for icons.
Q3: How are CRUD operations implemented?
A3: CRUD operations are implemented using Angular services for API calls, with forms used for input and display.
Q4: What features does the dashboard include?
A4: The dashboard displays total employee count, total projects, recently hired employees, and the latest project summaries.
Q5: Can I extend the functionality of this project?
A5: Yes, you can extend this project by adding more features such as advanced search, filtering, or more complex business logic.