Employee shift scheduling AI using Python
Science & Technology
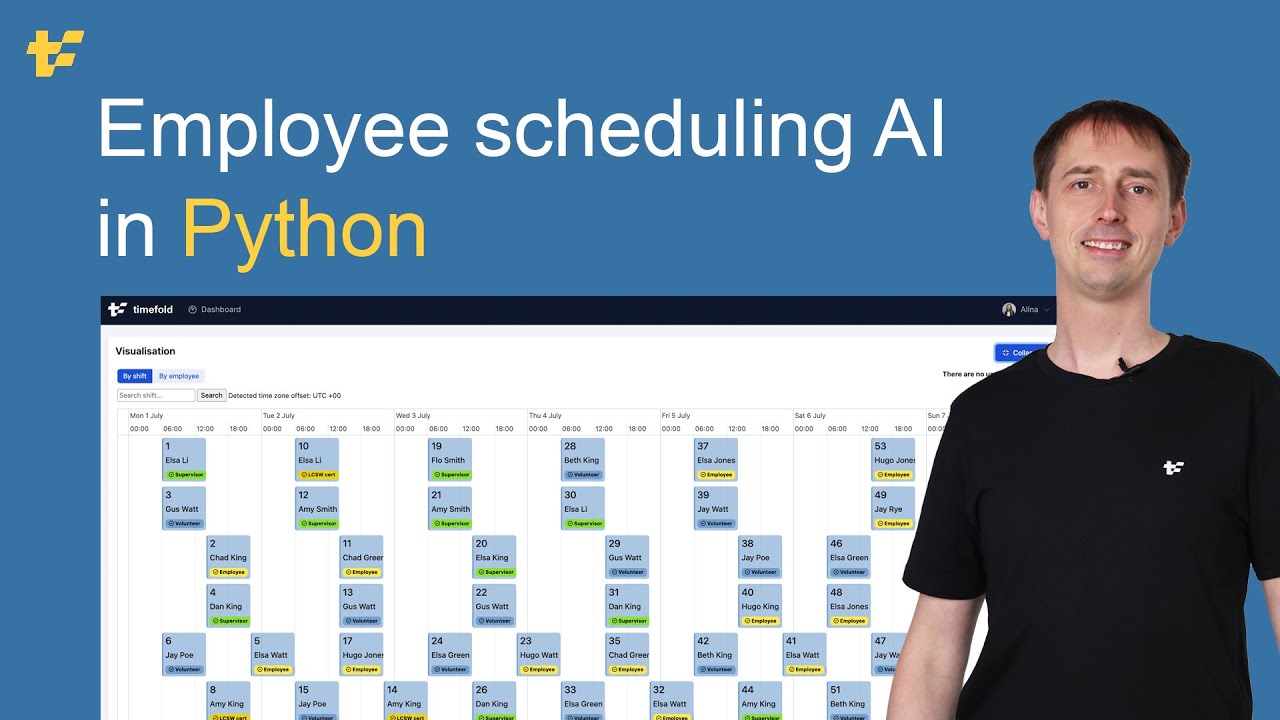
Introduction
In today’s fast-paced work environment, effective employee shift scheduling can significantly affect operational efficiency, employee satisfaction, and overall service quality. In this article, we explore how to utilize Python along with the Timefold open-source solver to automate the assignment of employees to shifts. We will discuss setting up the environment, developing the model, constraints, and optimization techniques.
Setting Up Timefold
To start creating an automated employee scheduling system, we need to set up the Timefold quick start repository. Follow these steps to initialize your project:
- Clone the Repository: Use Git to clone the Timefold quick start repository.
- Navigate to the Directory: Move into the
python/sl_employee_scheduling
directory. - Install Timefold: Execute the command
pip install timefold
to install Timefold from PyPI. - Run the Quick Start: Follow the instructions in the repository's README file to launch the demo application.
Once the application is up and running, you can access it via your web browser. Here, you will see a visual representation of shifts and employees, which allows you to proceed with the scheduling.
Understanding the Application
Within the demo application, you can see various shifts that are initially unassigned to employees. Each employee is associated with a set of skills, and preferences regarding when they are willing to work. For example:
- Availability: Chad is unavailable on July 8, while Elsa dislikes working on July 11.
- Preferences: Bley prefers to work on July 12.
Clicking the "Solve" button invokes your Python code, which assigns available shifts to employees while taking into account their availability and preferences.
Exploring the Code
The core logic for the application can be found in several Python files organized for clarity. Key files include:
- Domain File: This file contains domain classes which include Employee and Shift definitions. Each employee has a name, a set of skills, and lists for unavailable dates and preferred working days. Shifts have start and end times, locations, and required skills.
class Employee:
def __init__(self, name: str, skills: set, unavailable_dates: list, desired_dates: list):
#... initialize attributes
- Shift File: Represents shifts that are assigned to employees.
After defining the domain classes, a demo data file is included to automatically generate sample data for employees and shifts.
Implementing Constraints
Timefold allows the inclusion of various hard and soft constraints within the scheduling model:
- Hard Constraints:
- No Overlapping Shifts: Ensures that no employee can have two shifts at the same time.
- Required Skills: Checks that assignments only occur if an employee possesses the required skills for the shift.
if shift1.employee == shift2.employee and shifts_overlap(shift1, shift2):
penalize("Overlapping shifts detected.")
- Soft Constraints: These are preferences that can be maximized to improve employee happiness and service quality. For instance, rewarding employees for being assigned to a preferred shift.
if shift.start_date in employee.desired_dates:
reward("Preferred shift assigned.")
Testing Constraints
To ensure that our constraints are functioning correctly, unit tests are implemented. For example, testing a required skill constraint verifies if the system correctly penalizes unqualified employees from receiving specific shifts.
Conclusion
Using Timefold in Python, organizations can efficiently automate the process of scheduling shifts. By clearly defining the constraints and using optimization techniques, businesses can balance operational needs with employee preferences and skills.
Keyword
- Employee Scheduling
- Python
- Timefold
- Shift Assignment
- Constraints
- Hard Constraints
- Soft Constraints
- Optimization
- Demo Application
- Skills Requirement
FAQ
What is Timefold?
Timefold is an open-source solver for planning and scheduling problems in Python.
How do I install Timefold?
Clone the Timefold quick start repository and run pip install timefold
in the python/sl_employee_scheduling
directory.
Can I customize the shift scheduling?
Yes, you can modify the demo data and constraints to suit your specific scheduling needs.
What kind of constraints can I implement?
You can add both hard constraints (e.g., no overlapping shifts) and soft constraints (e.g., rewarding preferred shifts) to cater to the needs of the scheduling process.
Does the application support skill matching?
Absolutely! The application checks for required skills before assigning shifts to ensure that employees are qualified for their roles.