Full-Stack Resume Builder | For Beginners | ReactJS, Firebase, React Query
Education
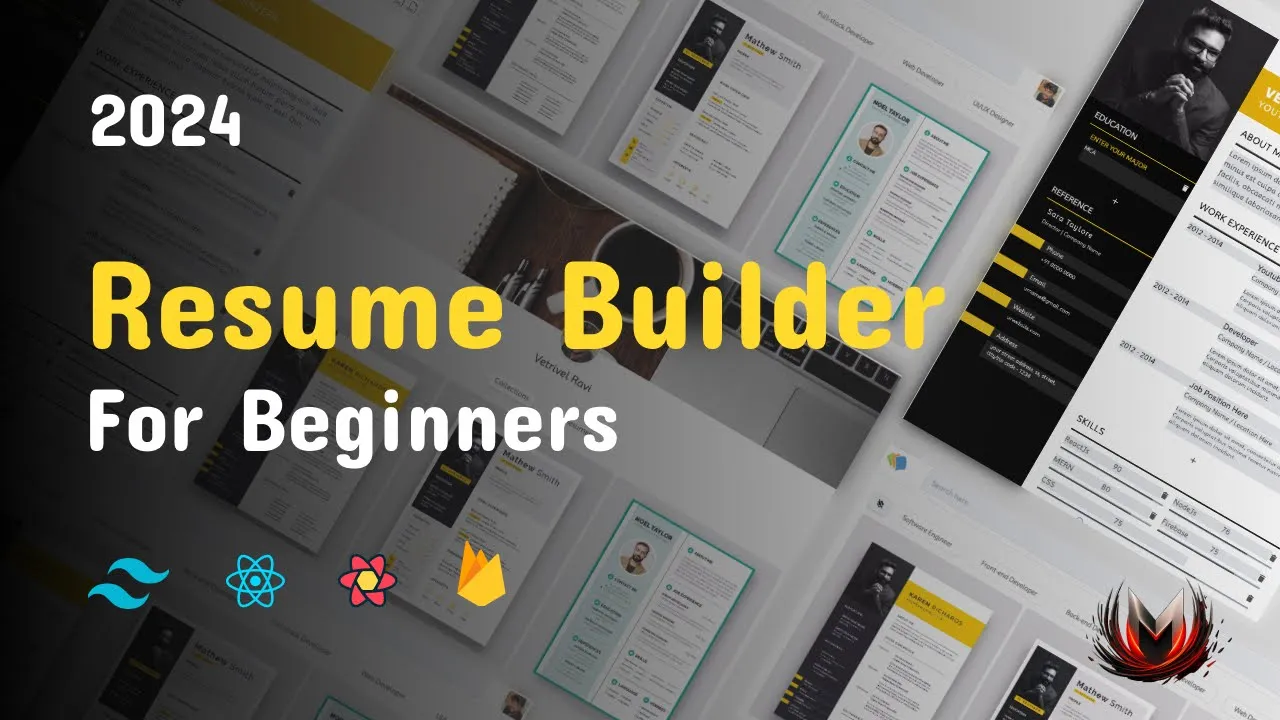
Introduction
Welcome back, everyone! In this tutorial, we'll learn how to build a fully functional resume builder web application using ReactJS, Firebase, React Query, and CSS. Even if you're a beginner, don't worry; this guide will walk you through each step in detail.
Table of Contents
- Introduction
- Setting Up Your Project
- Creating the Resume Builder UI
- Implementing Authentication
- Managing State with React Query
- Adding and Removing Templates
- Finalizing the Application
- FAQs
Introduction
The Resume Builder we are going to create features user authentication (with Google and GitHub), template management, and the ability to download resumes in various formats. We'll use Firebase for backend services and React Query for state management.
Setting Up Your Project
We start by creating a new project in Visual Studio Code. Use the command yarn create react-app resume-builder
to set it up. After that, we install the necessary packages, including Firebase, React Router, React Query, and Tailwind CSS.
yarn add firebase react-router-dom react-query tailwindcss
Next, we set up a Firebase project where we’ll manage templates and user data. Follow the instructions on Firebase to create a new project and enable Firestore and Firebase Authentication.
Creating the Resume Builder UI
We will design a clean user interface (UI) using Tailwind CSS that allows users to log in, create, and save resumes. The UI includes an authentication screen and a main screen containing the resume templates.
We'll create UI components like Header
, Filters
, Template Design Pin
, and Footer
. For styling, we will utilize Tailwind classes to create responsive designs that adapt to different screen sizes.
Implementing Authentication
The application uses Firebase Authentication to allow users to sign in via Google or GitHub. We'll set up the necessary Firebase configuration and implement authentication functionality in our application. After logging in, users are directed to the main screen where they can manage their resumes and templates.
const handleLogin = async () => (
const provider = new firebase.auth.GoogleAuthProvider();
try {
const result = await firebase.auth().signInWithRedirect(provider);
) catch (error) (
console.error('Error during login:', error);
)
};
Managing State with React Query
Instead of using local component state, we will utilize React Query to manage our data. This ensures that we have consistent and updated data throughout our application.
We create a custom hook, useTemplates
, which fetches template data from Firestore and handles errors or loading states efficiently.
const ( data: templates, isLoading, error ) = useTemplates();
Adding and Removing Templates
The application allows users to add new templates, which are stored in Firestore. By implementing appropriate UI components, we ensure a seamless user experience for adding and removing templates.
We can use the storage reference of Firebase to upload images used in templates and then display them appropriately in our UI.
const addTemplate = async (templateData) => (
// Code to add template in Firestore
);
Finalizing the Application
After implementing all functionalities, we ensure to test the application for any bugs or issues. We also set up deployment to Firebase Hosting so that our application can be accessed online by others.
To deploy, we build the app and then use Firebase commands to host the application.
yarn build
firebase deploy
Keywords
RSS, Firebase, React, React Query, UI components, Authentication, State Management, Document ID, Firestore, Web Development, Deployment, Tailwind CSS.
FAQ
What technologies are used in this Resume Builder app?
- The app uses ReactJS for the frontend, Firebase for backend services, and React Query for state management.
Can I use other authentication methods besides Google and GitHub?
- Yes, Firebase supports various authentication providers; you can add others like email/password or Facebook.
How are templates stored in the application?
- Templates are stored in Firestore using a document structure. Each user can have multiple templates associated with their account.
Is this application responsive?
- Yes, the application is designed to be responsive using Tailwind CSS, adapting to different screen sizes.
Can I modify the templates after they have been created?
- Yes, users can edit their templates and save changes, which will be reflected in real-time in the application.
How do I deploy this application?
- You can deploy the application using Firebase Hosting after building the project with the command
yarn build
and using thefirebase deploy
command.
- You can deploy the application using Firebase Hosting after building the project with the command
By following the steps in this tutorial, you should be able to create a fully functional resume builder application as a beginner with the knowledge of ReactJS and Firebase. Happy coding!