Fully Local RAG for Your PDF Docs (Private ChatGPT Tutorial with LangChain, Ollama, Chroma)
Science & Technology
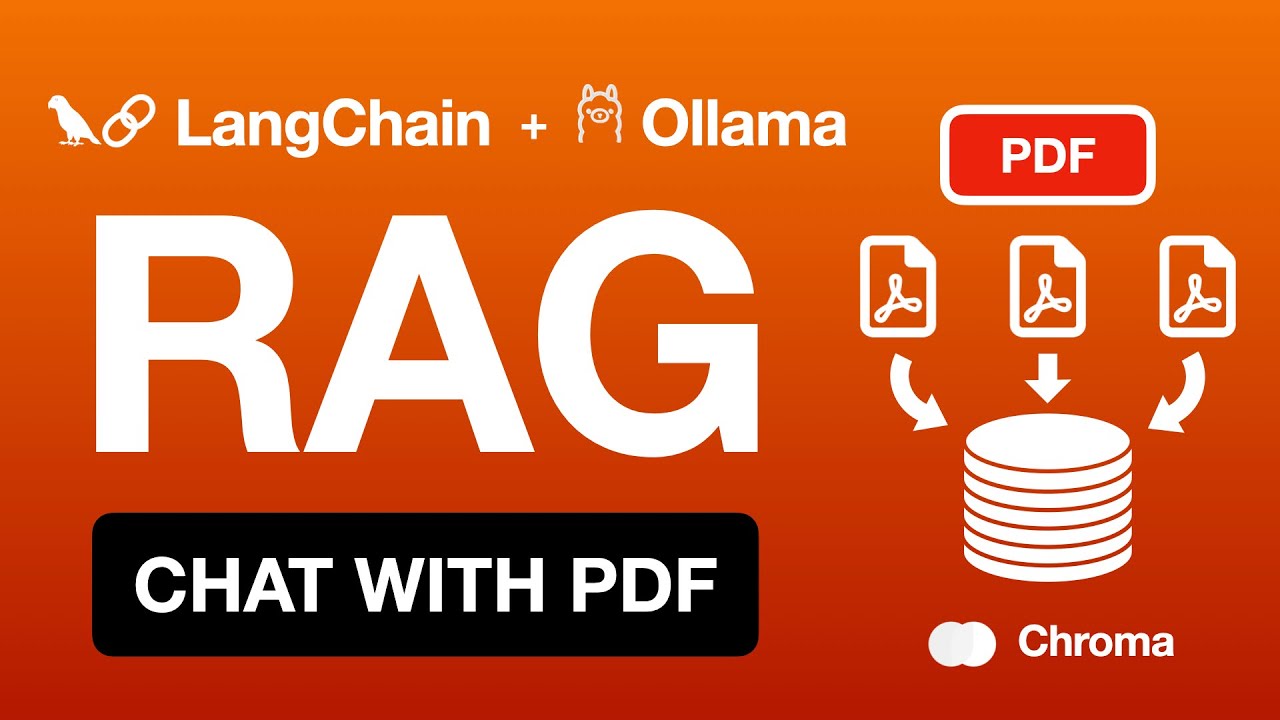
Introduction
In today's tutorial, we aim to create a completely local Retrieval-Augmented Generation (RAG) system that allows you to chat with your PDF files. PDFs have been a staple format for documentation, yet often users do not have the time to read through all that material. With advancements in parsing and RAG technology, we can transform the contents of these PDF files into a format suitable for querying via a local language model (LLM). This guide will take you through the process of building a chatbot that can interact with both single and multiple PDFs using LangChain, Ollama, and ChromaDB.
Why Use RAG with PDFs?
PDF files are ubiquitous, containing valuable information across various domains. By integrating a RAG system, we can effectively extract information from these documents without the need for tedious reading. This has practical applications in accessing large volumes of data quickly and efficiently.
Getting Started
Before diving into the coding aspect, make sure to check out the code repository at: Aid dev9 slts where you'll find the LangChain RAG PDF tutorial.
Inside the repository, you'll notice two folders, data
and DB
, along with several necessary files:
- Data Folder: Place PDFs here for ingestion.
- DB Folder: ChromaDB will store its database files here.
- requirements.txt: Contains required third-party libraries.
- models.py: Define your LLM and embeddings models here.
- ingest.py: Logic for ingesting PDFs into the system.
- chat.py: Script to run the chatbot.
How to Set Up
Here’s a quick run-down on how to set everything up:
- Clone the repository and navigate to it.
- Install dependencies by running:
pip install -r requirements.txt
- Install Ollama using:
- For Mac:
brew install llama
- For Windows, refer to the official website's instructions.
- For Mac:
- Download the LLaMA 3.2 model running:
You can change the model inama pull llama 3.2
models.py
if desired. - Drop your PDF files into the
data
folder. - Run the ingestion script:
python ingest.py
- In a second terminal, start the chatbot:
python chat.py
Tweaking Your Setup
Consider adjusting the chunk_size
and overlap_size
settings in ingest.py
for optimal performance based on your PDF contents. Explore different models by adding them in models.py
if you prefer.
You can also scrape content from websites and convert them to PDFs using a sample scraper provided in the repository. This feature is particularly useful for creating databases like OAS secure coding practices.
Key Components of the RAG System
- Document Ingestion: PDF files are divided into chunks and embedded into a vector database using Chroma DB.
- Querying: The user issues queries that the system processes, returning relevant chunks that enhance the context of the response generated by the LLM.
Coding the Models
Begin by defining your models in a new models.py
file:
- Import necessary libraries for embeddings and models.
- Define your variable for embeddings using
ama embed large
from the LLaMA website. - Set up models for both LLaMA 3.2 and Azure OpenAI as you see fit.
Next, create the ingest.py
file to handle file ingestion:
- Use
PyPDFLoader
to load and split the contents of PDFs. - Create unique IDs for documents and add them to the Chroma vector store.
For the chatbot logic, create chat.py
to interact with users:
- Initialize the models and vector store.
- Set up a main loop to accept user inputs, process them through the retrieval chain, and return responses based on the ingested documents.
Testing the Chatbot
Prepare sample PDF files and run your ingestion script. Once ingested, you can chat with your PDF contents, asking questions related to the document. The model should return accurate responses based on the PDF’s content.
Extending to Multiple Files
The tutorial also covers how to address larger datasets. By scraping documents from websites and converting them to PDFs, you can ingest multiple files at once, further demonstrating the system's capabilities.
As you experiment with various models and parameters, you might find different results in accuracy and comprehensiveness of responses. This flexibility allows you to tailor the system to your specific needs.
Keywords
- Local RAG
- PDF Chatbot
- LangChain
- Ollama
- ChromaDB
- Ingestion Script
- Embeddings
- Query Processing
FAQ
Q1: What is a RAG system?
A: A Retrieval-Augmented Generation system combines a retrieval model with a generative language model to provide more accurate responses based on context.
Q2: How can I modify my PDF ingestion settings?
A: You can alter the chunk_size
and overlap_size
parameters in the ingest.py
file based on the contents of your PDFs for better performance.
Q3: Is it possible to use other models in this setup?
A: Yes, you can include other models such as Azure OpenAI in the models.py
file and modify your scripts accordingly.
Q4: Can this system handle multiple PDF documents?
A: Absolutely! You can ingest multiple documents by placing them in the data
folder or even scrape web content and convert it to PDFs for ingestion.
Q5: How accurate are the replies from the chatbot?
A: Accuracy can vary depending on the size of chunks and the models used, but typically, you can expect 70-80% accuracy with proper configurations.