Generate AI Images with OpenAI DALL-E in Python
Science & Technology
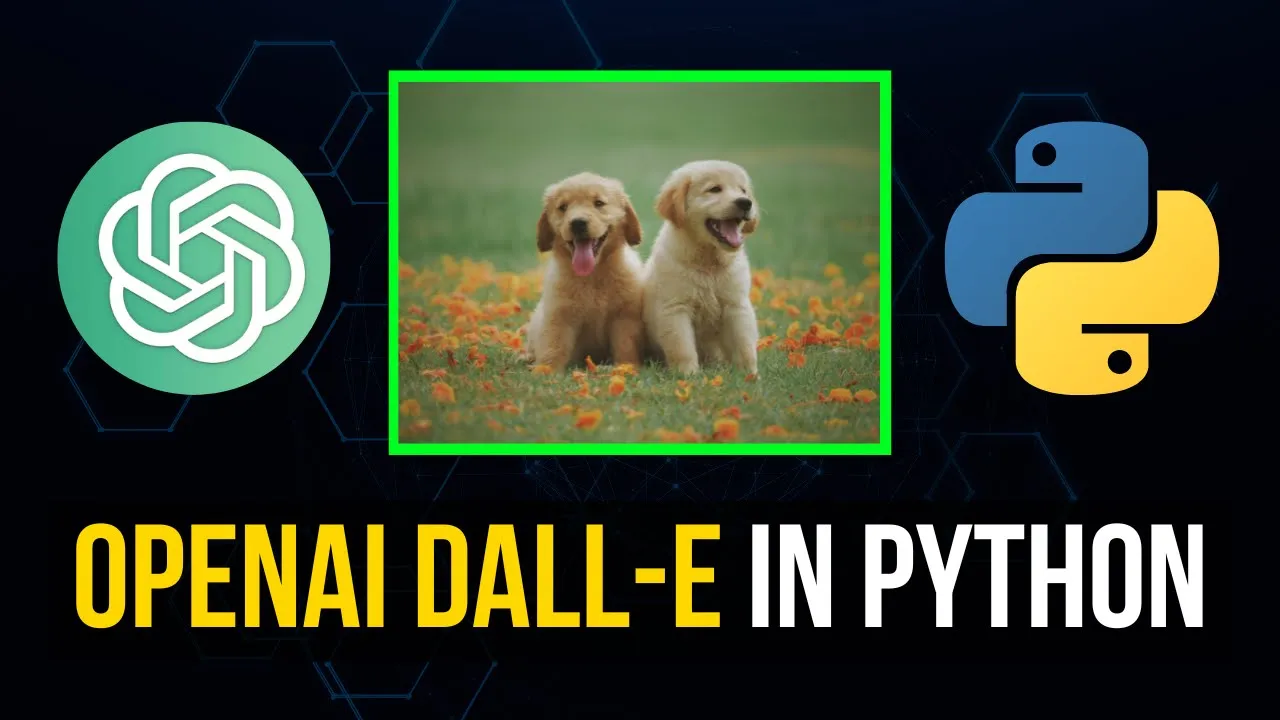
Introduction
Welcome back! In this guide, we will explore how to use OpenAI's DALL-E in Python to create AI-generated images as well as edit existing images. Let's dive right in!
Prerequisites
Before we start, you’ll need to have an OpenAI account. If you don’t have one yet, head over to platform.openai.com to create an account. If you already have a ChatGPT account, you can simply log in, as it uses the same credentials.
Obtaining the API Key
Once logged in, click on your account in the upper-right corner and choose "View API Keys." If you don’t see an API key, click on "Create New Secret Key" to generate one. Make sure to copy this key and keep it private, as anyone with access to it can authenticate as you and use your credits.
Installation
To use the DALL-E functionalities, you need to have the OpenAI package installed. Open your command line and run:
pip install openai
Authenticating with API Key
In your Python environment, you will need to authenticate with your API key:
import openai
## Introduction
with open("api_key.txt", "r") as file:
openai.api_key = file.read().strip()
Image Generation
You can request image generation with a simple prompt. For example, let’s generate an image of "five yellow dogs playing ball in the rain." Here is how you can do it:
response = openai.Image.create(
prompt="five yellow dogs playing ball in the rain",
n=1,
size="1024x1024"
)
image_url = response['data'][0]['url']
print(image_url)
When you run this code, a link to the generated image will be printed, which you can click to view the result. Keep in mind that results can vary, and sometimes the model may not perfectly fulfill your prompts.
Image Editing
DALL-E also allows you to edit images. To do this, you need a base image and a mask image (where a specific area has been removed to indicate what to change). Here is the basic workflow:
- Generate or find a base image.
- Create a mask image by removing the area you want to edit (use a transparent background).
- Run a similar code snippet as before to modify the base image. For example:
response = openai.Image.create_edit(
image=open("base_image.png", "rb"),
mask=open("mask_image.png", "rb"),
prompt="a sunlit indoor lounge area with a pool containing a flamingo",
)
edited_image_url = response['data'][0]['url']
print(edited_image_url)
Variations of Images
You can also generate image variations using an existing image. This involves uploading an existing image and requesting a similar version. Here’s how:
response = openai.Image.create_variation(
image=open("dog_image.png", "rb"),
n=1,
)
variation_image_url = response['data'][0]['url']
print(variation_image_url)
Conclusion
You can now generate, edit, and create variations of images using OpenAI’s DALL-E in Python! Experiment with different prompts and settings to see what results you can achieve. Thank you for following along; I hope you found this guide helpful. Remember to like, comment, and subscribe for more tutorials!
Keywords
OpenAI, DALL-E, Python, API Key, Image Generation, Image Editing, Variations, AI Images, Prompt
FAQ
1. Do I need an OpenAI account to use DALL-E?
Yes, an OpenAI account is required to access DALL-E API functionalities.
2. How do I get my OpenAI API key?
You can generate or view your API key from the OpenAI platform under your account settings.
3. What kind of prompts can I use?
You can use descriptive prompts for image generation, but keep in mind that the quality of results may vary depending on the complexity of the prompt.
4. What is the difference between image generation and image editing?
Image generation creates a new image based on a prompt, while image editing modifies an existing image using a base image and a mask.
5. How much does it cost to use the OpenAI DALL-E API?
Pricing varies, but generating and editing images typically starts at a lower amount (e.g., $ 0.02 per image). Check OpenAI's pricing page for details.