[HINDI] #08 Dart -Functions | Complete Flutter + AI Course for Beginners
Education
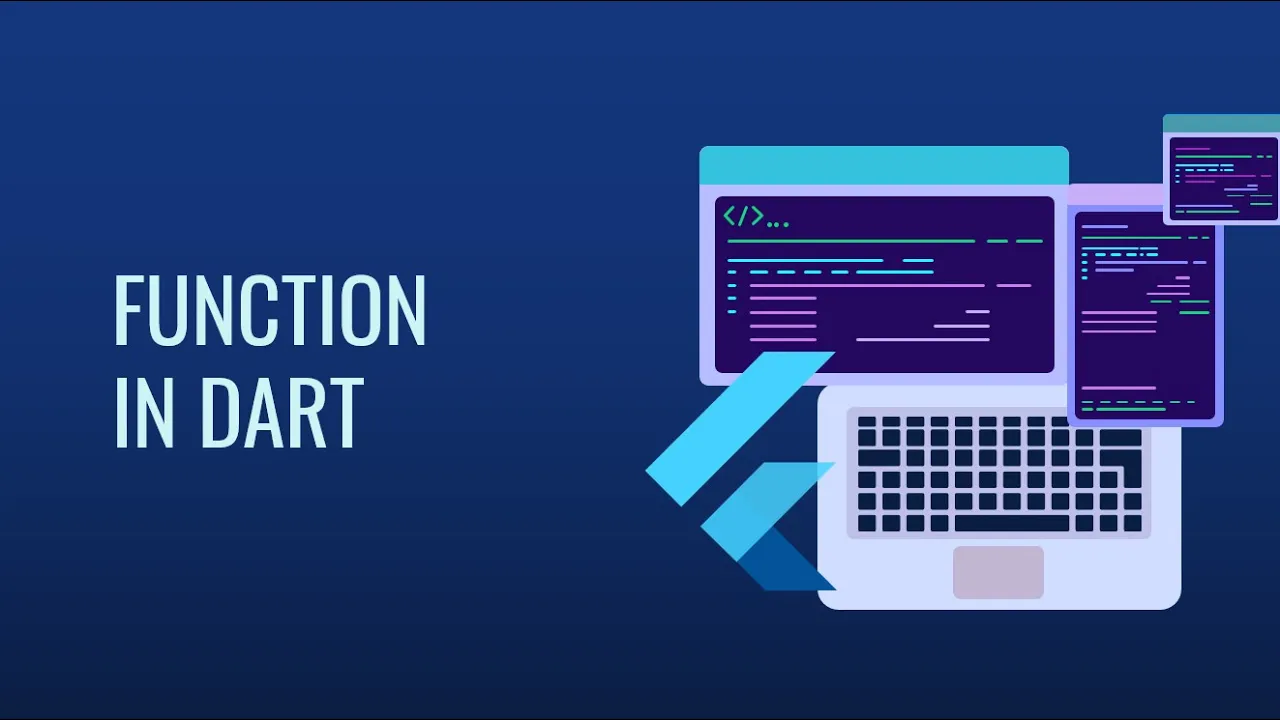
Introduction
In today's video of our Flutter Application Development series, we dive deep into the essential concept of functions in Dart. Functions are a vital aspect of every programming language, and understanding them is crucial for any developer.
What are Functions?
Functions in programming are akin to functions in mathematics. Recall from your math classes how you would have a function, say f(x)
, that took a value as input and returned a calculated output based on a defined equation. For example, if f(x) = 3x + 5
, when you input x
, the function would replace x
with the value you passed and perform the calculations defined in it.
However, functions in programming are far simpler and much more practical. They allow developers to encapsulate blocks of code so that they can be executed whenever needed, which significantly enhances code reusability and efficiency.
Real-life Example of Functions
To illustrate the practical use of functions, let’s say you have a scenario where you need to generate bills for numerous products in a store. In this scenario, each bill might require a batch ID, MRP, manufacturing date, and expiry date. If there are 200,000 products, creating individual bills manually would be a nightmare. Instead, we can create a function that automates the bill creation process. When called, it would execute the necessary steps to print a bill without the need to rewrite code for each product.
Creating Functions in Dart
Let’s explore how to define and implement functions in Dart.
Defining a Function: When defining a function, you specify the return type, the function name, and if necessary, parameters that the function will accept. For instance:
void performAction() ( // Your code here )
Calling a Function: To execute the function, you simply call it by its name:
performAction();
Parameters and Return Types: Functions can accept multiple types of parameters, and you can specify what type of value the function will return. You can have functions return integers, strings, lists, maps, or any other data type.
Example of Adding Two Numbers:
int add(int a, int b) ( return a + b; )
Using Functions with Arguments: When you call a function, you can pass specific values (arguments) that correspond to the parameters defined.
int result = add(2, 3); // result will be 5
Conclusion
Functions significantly simplify coding by allowing you to reuse code efficiently. They can be organized in such a way that any part of your program can call them at any time, leading to cleaner and more manageable code.
In the next video, we'll explore more advanced topics like lists, maps, and how to manipulate them, as well as taking user input and other essential functions.
Keyword
- Dart
- Functions
- Flutter
- Programming
- Encapsulation
- Code Reusability
- Parameters
- Return Types
- Example
- List
- Map
FAQ
Q1: What are functions in Dart? A1: Functions in Dart are blocks of code that perform specific tasks and can be called whenever needed, making code more reusable and manageable.
Q2: How do I define a function in Dart?
A2: To define a function in Dart, you specify the return type, function name, and include parameters if needed. For example: void myFunction() ( //code )
.
Q3: Can functions return values in Dart? A3: Yes, functions in Dart can return values, and you specify the return type when defining the function.
Q4: What is a parameter in a function? A4: A parameter is a variable that you define in a function to accept input values when the function is called.
Q5: How do I call a function in Dart?
A5: You call a function by simply using its name followed by parentheses, like this: myFunction();
.