How To Build A Trading Bot In Python
Education
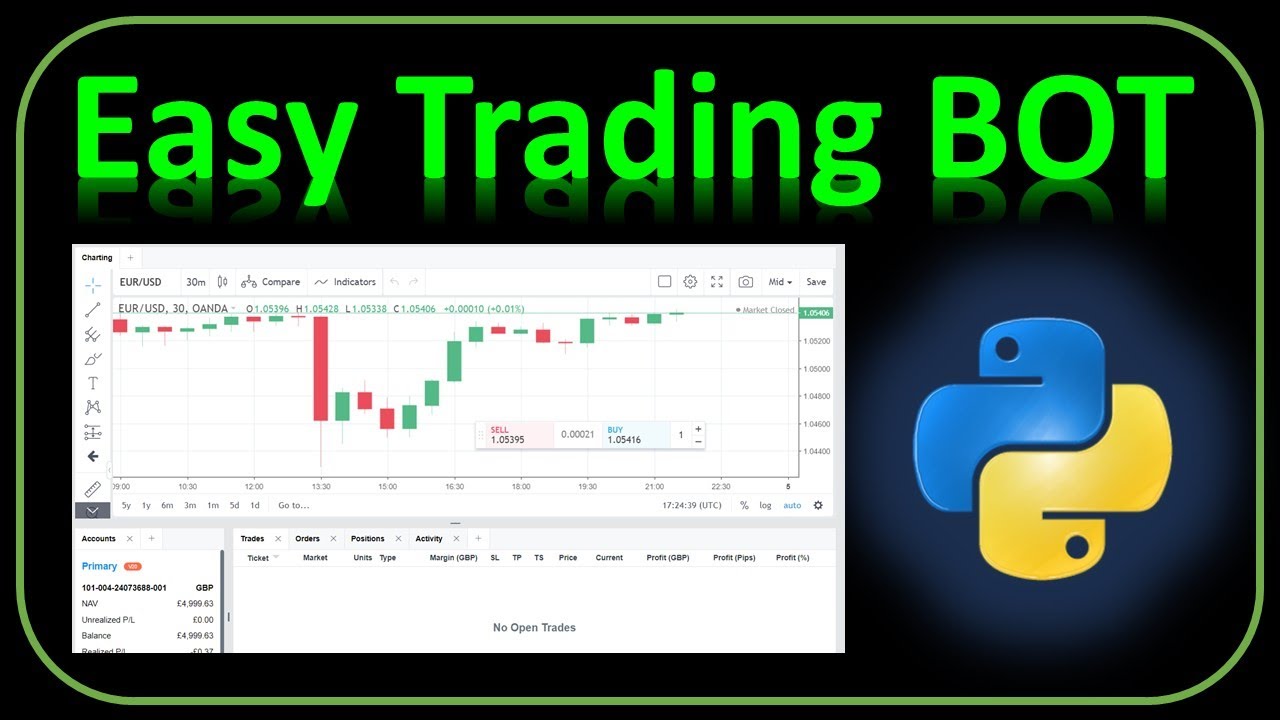
Introduction
Automating your trading strategy using Python can enhance your trading efficiency and take emotion out of the equation. In this article, we will guide you through building a simple trading bot that automatically executes trades based on a specific strategy. The code used in this guide is available for download, and we will leverage it with the help of the OANDA broker API.
Steps to Build a Trading Bot
1. Set Up Your Python Environment
Start by ensuring you have Python installed on your machine, along with the required libraries such as Pandas, NumPy, and a library for accessing market data like yfinance
.
2. Download Historical Data
First, we will need historical exchange data. For this tutorial, we will utilize the yfinance
library to download Euro to US Dollar (EUR/USD) data at a 15-minute interval. Note that for low time frames like 15 minutes, Yahoo Finance only allows data for the last 60 days.
import yfinance as yf
## Introduction
data = yf.download('EURUSD=X', start='YYYY-MM-DD', end='YYYY-MM-DD', interval='15m')
3. Create a Signal Generator
Next, we define a signal generator function. This function analyzes historical candlestick patterns to identify engulfing candle patterns which indicate potential buy or sell signals.
def signal_generator(df):
if df['Close'].iloc[-1] > df['Open'].iloc[-1] and df['Open'].iloc[-1] < df['Close'].iloc[-2]:
return 2 # Buy signal
elif df['Close'].iloc[-1] < df['Open'].iloc[-1] and df['Open'].iloc[-1] > df['Close'].iloc[-2]:
return 1 # Sell signal
else:
return 0 # No signal
4. Test the Signal Generator
We can test our signal generator with our downloaded data to see how many signals it generates.
def test_signal_generator(data):
signals = []
for i in range(1, len(data)):
signals.append(signal_generator(data[i-1:i+1]))
return signals
data['Signals'] = test_signal_generator(data)
5. Connect to OANDA API
Before executing trades, you need to have an account with a broker that supports API connections. OANDA is used as an example here. You will need your API access token and account ID.
from oanda_api import Client
client = Client(access_token='your_access_token', account_id='your_account_id')
6. Define Functions for Trading
Now we’ll define functions to acquire live candle data, execute trades, and set take-profit and stop-loss parameters.
def get_candles():
return client.get_latest_candles('EUR/USD', count=3)
def trading_job():
candles = get_candles()
signal = signal_generator(candles)
if signal == 1:
# Execute sell order
client.create_order(instrument='EUR/USD', units=-1000) # Selling 1000 units
elif signal == 2:
# Execute buy order
client.create_order(instrument='EUR/USD', units=1000) # Buying 1000 units
7. Schedule the Trading Job
Finally, we set a scheduler to execute the trading job at specified intervals (e.g., every 15 minutes).
from apscheduler.schedulers.background import BackgroundScheduler
scheduler = BackgroundScheduler()
scheduler.add_job(trading_job, 'interval', minutes=15)
scheduler.start()
Conclusion
Now you have set up a simple trading bot that connects to the OANDA API and executes trades based on engulfing patterns. This approach can be adapted and expanded with more sophisticated strategies and risk management techniques.
Feel free to modify the signal functions and trading parameters to suit your unique trading strategy. Always remember to test your bot in a demo account before deploying it with real money.
Keywords
trading bot, Python, automate trading, financial trading, signal generator, OANDA API, historical data, market order, backtesting, scheduling
FAQ
Q: What is a trading bot?
A: A trading bot is a software program that interacts directly with financial exchanges to place buy or sell orders based on predefined parameters, which can improve efficiency and remove emotional decision-making from trading.
Q: Which libraries do I need for building a trading bot in Python?
A: You'll typically need libraries like Pandas for data manipulation, NumPy for numerical calculations, and libraries for market data access and API interactions, such as yfinance
and requests for HTTP requests.
Q: How can I backtest my trading strategy before going live?
A: You can use historical data to simulate your trading strategy over past market conditions. There are many backtesting libraries available in Python, such as Backtrader or Zipline, that can help with this process.
Q: What should I do if my trading bot doesn't execute trades as expected?
A: Ensure your API connection is correctly set up, check the trading strategy for errors, and verify that your signals are being calculated accurately. Testing your bot in a sandbox or demo environment can help troubleshoot issues before going live.
Q: Can I manually intervene in trades executed by the bot?
A: Yes, you can manually modify or close trades from your broker's platform even if they were placed by the bot. However, this should be done cautiously as it may affect the strategy's intended performance.