How to build AI Travel Planner (Next.js, Drizzle, Shadcn, OpenAI, Cursor)
Science & Technology
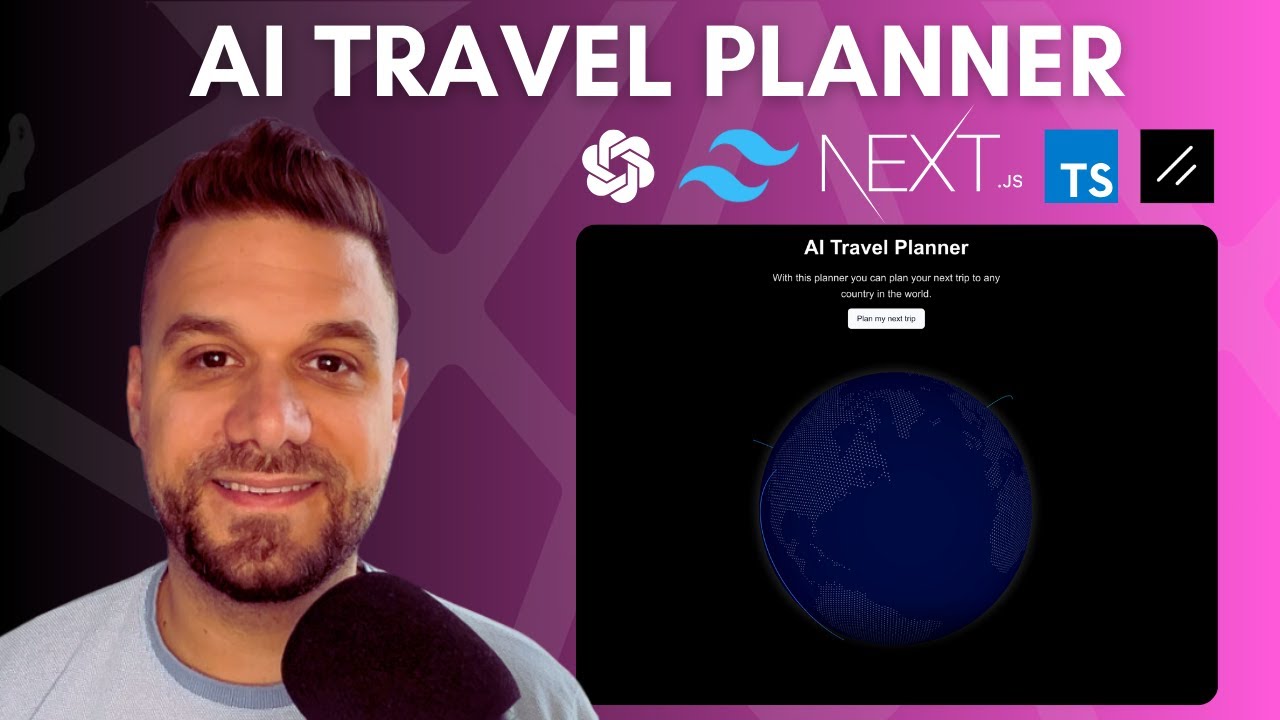
Introduction
In this article, we will walk through the process of creating an AI Travel Planner using Next.js, Drizzle ORM, Shadcn UI, and the OpenAI API. This planner will allow users to create personalized travel itineraries based on their preferences. The application includes user authentication, an interactive form for gathering travel details, and dynamic rendering of travel plans as HTML.
Table of Contents
- Setting Up the Project
- Creating the Landing Page
- Integrating Clerk for Authentication
- Building the Travel Planner Form
- Connecting to OpenAI API
- Saving Plans in Drizzle ORM
- Displaying the Travel Plans
- Setting Up the My Plans Page
- Conclusion
Setting Up the Project
To get started, create a new Next.js application with TypeScript and install necessary dependencies. Run:
npx create-next-app@latest AI-travel-planner --ts
After the setup is complete, navigate to the project directory and run it:
cd AI-travel-planner
pnpm dev
Adding Shadcn UI
Initialize Shadcn UI by running:
npx shadcn@latest init
Select the New York style and Slate color, then install additional dependencies including those required for 3D graphics.
Creating the Landing Page
Utilize the Exertility UI library for an appealing landing page. Begin by installing dependencies for Framer Motion and related libraries. Create a Globe
component to act as the main feature of the landing page, allowing users to navigate the globe.
// In components/UI/Globe.tsx
// Add code for the globe component here
After successfully setting up the globe, style it for a dark mode experience.
Integrating Clerk for Authentication
Use Clerk for user management. Install the Clerk Next.js package:
pnpm add @clerk/nextjs
Set up environment variables in a .env.local
file for secure API key management. Implement middleware for protected routes, ensuring users must log in to create travel plans.
Setting Up Authentication UI
Create custom sign-in and sign-up pages to enhance user experience. Make sure that users are redirected back to their planned trips upon successful login.
Building the Travel Planner Form
Create a TravelPlannerForm
component. Use Shadcn's form components to gather inputs such as:
- Start Date
- End Date
- Budget
- Activities
- Destination (optional)
Utilize the Calendar component for date selection, ensuring that users cannot select past dates and that the end date is after the start date.
Connecting to OpenAI API
Configure the OpenAI API for generating travel details. Create a mutation to process the user's travel data into a structured prompt. Make use of Zod for validation, ensuring data entered by users complies with the expected format.
Here’s an example prompt structure:
const prompt = `I'm planning a trip to $(destination) from $(startDate) to $(endDate) with a budget of $(budget) and I want to do the following activities: $(activities.join(', ')).`;
Saving Plans in Drizzle ORM
Set up Drizzle ORM for database interactions. Define a plans
table for storing users' travel itineraries. Save the data submitted through the form, including user details for easy retrieval later.
Use the following structure in your database schema:
// In your schema file
export const Plans = (
id: uuid(),
userId: string(),
text: string(),
budget: number(),
createdAt: timestamp(),
startDate: date(),
endDate: date(),
);
Displaying the Travel Plans
Create a dedicated page to reveal users’ plans. Utilize a Plan
component to display information fetched based on the selected plan's ID. Make use of dangerouslySetInnerHTML
to render HTML content dynamically.
Setting Up the My Plans Page
Create a My Plans page where users can view all their created travel itineraries. Fetch plans from the database, filter them based on the logged-in user, and display them neatly with options to view or edit.
Conclusion
With the above steps, you will have a fully functional AI Travel Planner that offers personalized experiences based on user input. Users can easily create, view, and manage their travel plans, ensuring a seamless travel planning experience.
Keywords:
Next.js, Drizzle ORM, Shadcn UI, OpenAI, Clerk, travel planner, authentication, travel itinerary, AI, reactive forms.
FAQ:
What technologies are used in the AI Travel Planner?
The project uses Next.js, Drizzle ORM for database management, Shadcn UI for user interface components, Clerk for authentication, and OpenAI for generating travel itineraries.How does user authentication work?
User authentication is managed through Clerk, which allows users to securely sign up and log in. The middleware ensures that only authenticated users can access travel planning features.Can users customize their travel plans?
Yes, users can specify their travel dates, budget, activities, and desired destinations when creating plans. The AI generates a tailored itinerary based on this input.Is it possible to see previously created plans?
Absolutely! Users can navigate to the "My Plans" page to view all their previous travel itineraries, which are stored in the database.How are travel plans stored?
Travel plans are stored in a PostgreSQL database using Drizzle ORM, allowing for effective CRUD operations and data management.