How to Code a AI Trading bot (so you can make $$$)
Science & Technology
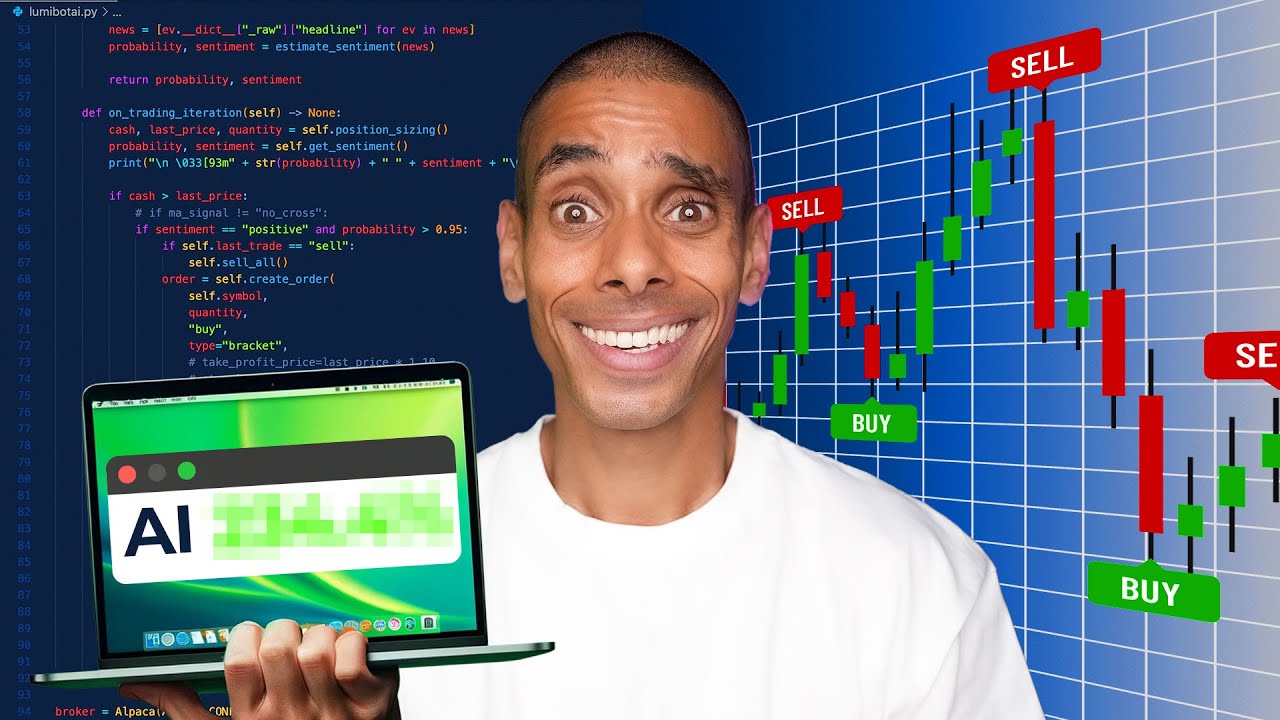
Introduction
Algorithmic trading has become a cornerstone for many hedge funds, allowing them to automate their trading strategies and capitalize on market opportunities. With firms like Renaissance Technologies and Two Sigma leading the charge, the appeal of an AI-powered trading bot is undeniable. But can anyone create such a bot? In this article, we will explore how to build your own fully automated AI trading bot through five essential steps.
Step 1: Building the Baseline Bot
To kick things off, we’ll create the foundational component of our trading bot. Start by creating a new Python file named trading_bot.py
. You’ll need to import several libraries, particularly from the lumot
library, which provides an excellent framework for trading.
from lumot.Brokers import Pacer
from lumot.dob.testing import YahooDataBacktesting
from lumot.strategies.strategy import Strategy
from lumot.Trader import Trader
from datetime import datetime
Next, you will need API keys to fetch market data. These keys can be generated through an Alpaca account. Once you've obtained your API keys, create a dictionary to hold them.
alpaca_creds = (
"API_key": "", # Add your API key here
"API_secret": "", # Add your API secret here
"base_URL": "" # Add your base API URL here
)
Now, you can establish the broker and set up your strategy by creating a new class inheriting from Strategy
. This will form the backbone of your bot, encapsulating all trading logic.
class MLTrader(Strategy):
def initialize(self):
# Initialization logic
pass
def on_trading_iteration(self):
# Trading logic executed on each tick
pass
Step 2: Implementing Position Sizing and Limits
With the baseline bot created, the next step is to implement position sizing and cash management strategies. Instead of making static trades, you will create dynamic positions based on your cash balance. The idea is to only risk a specified percentage of your capital on each trade, which is critical for sustainable trading.
You can calculate position size dynamically based on the cash at risk. Additionally, setting a take profit and stop-loss limit helps manage trades better.
def calculate_position_size(self):
cash = self.get_available_cash()
last_price = self.get_last_price(self.symbol)
cash_at_risk = 0.5 # 50% of cash
position_size = (cash * cash_at_risk) // last_price
return position_size
Step 3: Gathering News
Next, we need to integrate a component for gathering news to inform trading decisions. This can be accomplished through an API call to Alpaca to retrieve relevant news articles for a specified symbol.
def get_news(self):
news = self.api.get_news(symbol=self.symbol)
cleaned_news = [("headline": event['headline'], "date": event['date']) for event in news]
return cleaned_news
This will help the bot assess market sentiment based on recent developments.
Step 4: Integrating Machine Learning
At this stage, we can utilize machine learning models, such as FinBERT, to assess sentiment from the gathered news. Sentiment analysis helps dictate whether to take a long or short position based on whether the news is seen as positive or negative.
from finbert_utils import estimate_sentiment
sentiment, probability = estimate_sentiment(news)
if sentiment == "positive" and probability >= 0.99:
self.buy()
elif sentiment == "negative" and probability >= 0.99:
self.sell()
Step 5: Finalizing the Trading Logic
The last step is to integrate your sentiment analysis into your trading algorithm. Based on the trading signals derived from the sentiment, issue buy or sell orders and dynamically manage your existing positions.
def on_trading_iteration(self):
news = self.get_news()
sentiment, probability = self.get_sentiment(news)
if sentiment == "positive" and self.last_trade != "buy":
self.execute_trade("buy")
elif sentiment == "negative" and self.last_trade != "sell":
self.execute_trade("sell")
After all these integrations, you can run backtests to evaluate the performance of your trading strategy. Monitor how well your bot performs against market benchmarks.
Conclusion
Building an AI trading bot is an exciting journey that combines programming with finance. While the outlined bot shows promising results in backtests, remember that live trading involves different dynamics such as slippage, transaction costs, and market impact.
Keyword
- AI Trading Bot
- Algorithmic Trading
- Position Sizing
- Cash Management
- Machine Learning
- Sentiment Analysis
- Backtesting
- Trading Strategy
FAQ
1. Can anyone create an AI trading bot? Yes, with the right programming knowledge and resources, anyone can create an AI trading bot.
2. What programming language do I need to build a trading bot? Most trading bots are built using Python due to its rich ecosystem and libraries available for data science and trading.
3. How important is sentiment analysis in trading? Sentiment analysis can provide insights about market reactions and help in making informed trading decisions.
4. What is backtesting, and why is it important? Backtesting involves testing a trading strategy on historical data to evaluate its performance before deploying it in a live market.
5. How do I implement risk management strategies in my bot? Risk management can be implemented through position sizing, setting stop-loss limits, and determining how much capital to risk on each trade.