How to Create a Cloud-Based POS System Using ChatGPT AI | HTML, CSS, JS, PHP, MySQL | website
Science & Technology
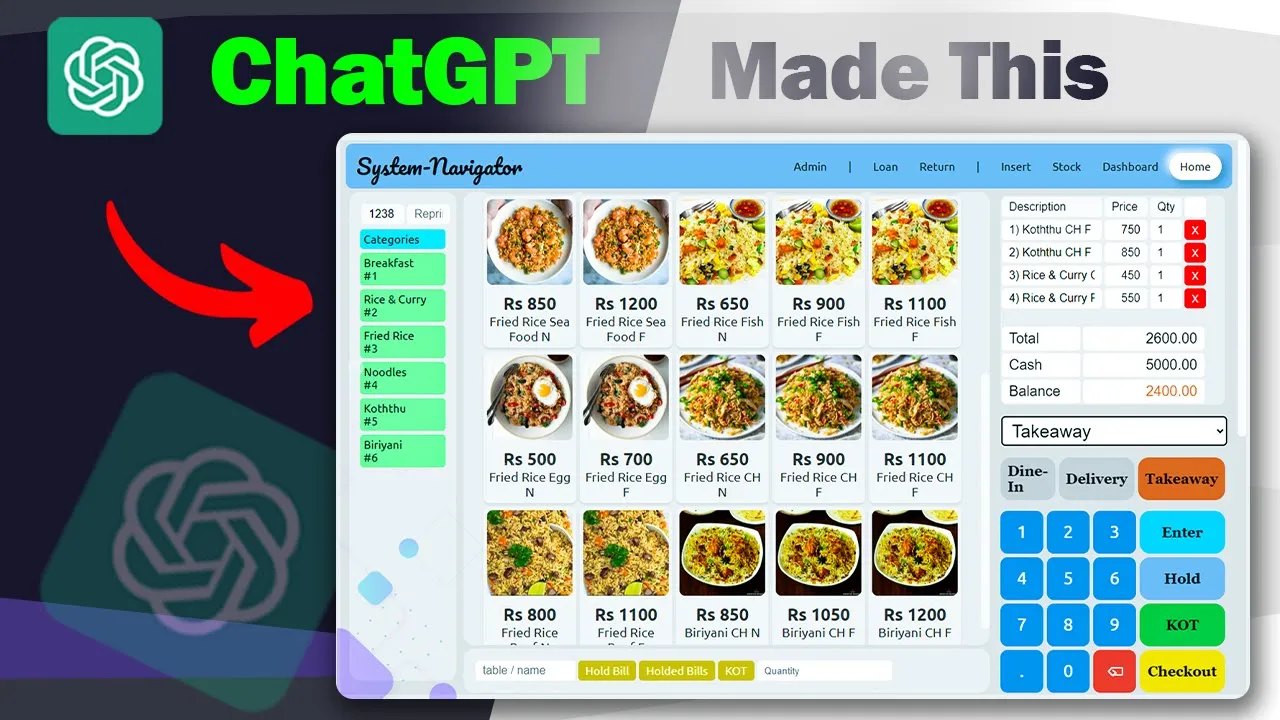
Introduction
Creating a Point of Sale (POS) system can seem daunting, but with tools like ChatGPT and a solid understanding of web technologies, you can develop a fully functional system without needing to master each programming language. In this guide, we'll walk through setting up a cloud-based POS system using HTML, CSS, JavaScript (JS), PHP, and MySQL. By the end, you will have the skills needed to offer billing system development services and potentially earn from it.
Project Overview
A basic POS system consists of at least two essential pages: a billing page and an insertion page for adding items. This article will guide you through the process of creating these pages, how to manage your backend and database, and how to implement user interactions.
Step 1: Setting Up the Environment
Install XAMPP: Start by downloading and installing XAMPP, which will allow you to run a local server for your project. Check previous tutorials on how to set up XAMPP if needed.
Folder Structure:
- Create a folder called
POS
in the root directory of your XAMPP server. - Inside this folder, create an
index.html
file for the insertion page and aninsert_stock.php
file for the backend logic.
- Create a folder called
Uploads Folder: Create a folder named
uploads
in yourPOS
directory to store uploaded images.
Step 2: Creating the Insert Page
HTML Code (index.html)
Include a form in your HTML for inputting item details such as category name, price, and an image.
<form id="insertForm">
<input type="text" name="category" placeholder="Category Name" required>
<input type="number" name="price" placeholder="Price" required>
<input type="file" name="image" accept="image/*" required>
<button type="submit">Add Item</button>
</form>
JavaScript Code (script.js)
Use JavaScript to handle form submissions via AJAX, sending the data to your PHP backend script.
document.getElementById('insertForm').addEventListener('submit', function(e) (
e.preventDefault();
// Implement AJAX call to insert_stock.php
));
PHP Code (insert_stock.php)
This script will process the AJAX request, handle file uploads, and insert the data into your MySQL database.
<?php
// Database connection and insert logic here
?>
SQL Code to Create Stock Table
You need a database to store your items. Use phpMyAdmin to create a database named POS
and then run the following SQL to create a stock
table:
CREATE TABLE stock (
id INT(11) AUTO_INCREMENT PRIMARY KEY,
category VARCHAR(255) NOT NULL,
price DECIMAL(10, 2) NOT NULL,
image_path VARCHAR(255) NOT NULL
);
Step 3: Testing the Insert Page
- Start your Apache and MySQL servers using XAMPP.
- Go to the localhost in your browser and test inserting items using your form.
After the items are added successfully, check the stock
table in your database and the uploads
folder for the images.
Step 4: Building the Billing Page
Next, create a billing.html
file and set up the main functionality of the POS system. This page should display:
- Categories: Fetch and display item categories that do not duplicate.
- Items: On selecting a category, display items as cards with images.
- Bill Summary: Allow users to add items to a bill and include a checkout button.
Billing Page Code Example
This includes HTML, CSS, JavaScript, and PHP code to handle fetching categories and items, updating the bill, and generating a receipt.
Step 5: Checkout Functionality
Add a checkout button at the end of the billing section. This button will print the bill summary. When implemented, this finalizes the transaction process for users.
Conclusion
With this guide, you are equipped to create a simple yet effective cloud-based POS system. Enhance its functionality over time by gradually adding more features and improvements. Remember, your implementation may vary, so focus on understanding each method rather than replicating results.
Keywords
- POS System
- Cloud-Based
- HTML
- CSS
- JavaScript
- PHP
- MySQL
- AJAX
- Database
- User Interface
FAQ
Q: What technologies do I need to create a POS system?
A: You will need knowledge of HTML, CSS, JavaScript, PHP, and MySQL.
Q: Can I create a POS system without programming knowledge?
A: This guide minimizes the need for extensive programming skills by leveraging AI tools like ChatGPT.
Q: What is the purpose of the uploads folder?
A: The uploads folder stores images of the items added to the POS system.
Q: How do I handle database operations in this system?
A: Use PHP scripts to interact with the MySQL database for operations like inserting and retrieving items.
Q: How can I enhance my POS system in the future?
A: You can expand your system by adding features like user authentication, reporting, and integration with payment gateways.