How to Create Jarvis AI Assistant | Like Iron Man
People & Blogs
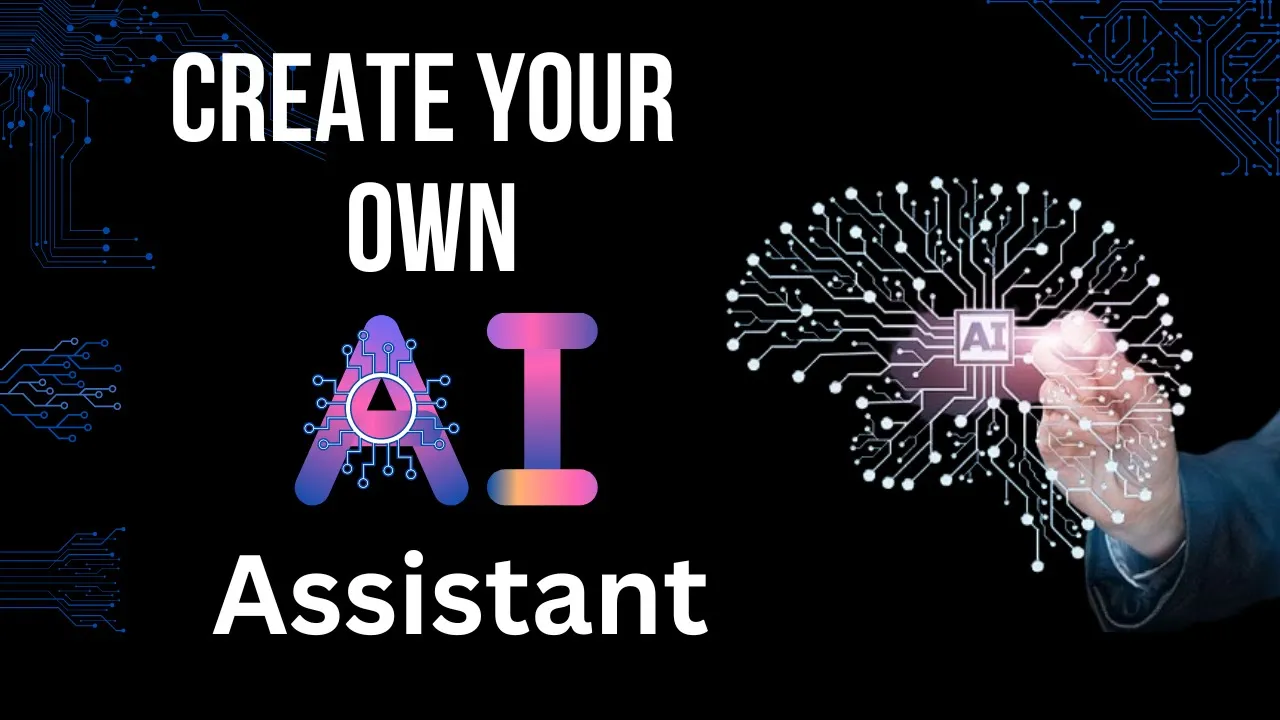
Introduction
Are you interested in creating your own AI assistant that goes beyond the capabilities of Siri or Alexa? Using OpenAI, you can create an interactive AI assistant in just about 25 lines of code. This guide will walk you through the process, explaining all the necessary steps.
Step 1: Setting Up Your Project
First, download PyCharm, an integrated development environment (IDE) that supports several programming languages. You can find the PyCharm download link in the description of the video, and follow the instructions to install it on your system. Once PyCharm is installed, create a new project.
Step 2: Getting an OpenAI API Key
To use OpenAI, you'll need an API key. Head to the OpenAI Platform and sign up for an account if you haven't already. Once logged in, navigate to 'API Keys' and create a new secret key. Make sure to copy and save this key, as you won't be able to view it again once you close the prompt.
Step 3: Setting Up Your Environment
Open your PyCharm project and set up your environment by importing the following modules:
import os
import time
import pyaudio
from playsound import playsound
from gtts import gTTS
import openai
import speech_recognition as sr
Step 4: Installing Dependencies
You'll need to install several packages. Use pip to install these:
pip install pyaudio playsound gtts openai speechrecognition
For macOS users, you might need to install PortAudio and Homebrew first. Use the following commands:
brew install portaudio
brew install pyaudio
pip install playsound gtts openai speechrecognition
Step 5: Creating the Python Script
Now, let's write the script. Start by saving your OpenAI API key in a variable:
API_KEY = "your_api_key_here"
Next, import the necessary modules and set up OpenAI:
openai.api_key = API_KEY
Define a function to get audio input:
def get_audio():
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Listening...")
audio = recognizer.listen(source)
said = ""
try:
said = recognizer.recognize_google(audio)
print(f"You said: (said)")
except Exception as e:
print("Exception: " + str(e))
return said
Define another function to get a response from OpenAI:
def get_response(text):
response = openai.ChatCompletion.create(
model="text-davinci-003",
messages=[("role": "user", "content": text)]
)
return response["choices"][0]["message"]["content"]
Finally, write the main logic:
while True:
text = get_audio()
if "Friday" in text:
response = get_response(text)
print(response)
speech = gTTS(text=response, lang='en')
speech.save("response.mp3")
playsound("response.mp3")
os.remove("response.mp3")
Step 6: Running the Script
Run your script, and your AI assistant named "Friday" will be ready to interact with you. You can ask it anything, like writing a haiku about programmers.
Here’s an example interaction:
You: "Friday, write a haiku about programmers." Friday: "Lines and code encode, Crafting genius with each byte, Programmers create."
Summary
By following the steps outlined in this guide, you’ll be able to build your very own voice-activated AI assistant using OpenAI’s API. This assistant can recognize your voice commands, generate responses using GPT-3.5, and even speak back to you using Google Text-to-Speech (gTTS).
Keywords
- AI Assistant
- OpenAI
- PyCharm
- Python
- Speech Recognition
- Google Text-to-Speech
- OpenAI API
- Pyaudio
FAQ
Q: What is OpenAI? A: OpenAI is an AI research and deployment company. Their mission is to ensure that artificial general intelligence (AGI) benefits all of humanity.
Q: How do I obtain an OpenAI API key? A: You can obtain an API key by signing up on the OpenAI Platform and navigating to 'API Keys.'
Q: What packages do I need to install for this project?
A: You need to install the following packages: pyaudio
, playsound
, gtts
, openai
, and speechrecognition
.
Q: Can this script run on macOS? A: Yes, though macOS users may need to install additional dependencies such as PortAudio and Homebrew.
Q: How do I interact with the AI assistant? A: You interact with the AI assistant by speaking into your microphone. The assistant will listen for the keyword "Friday," and then respond to your command or question.