How to create quote generator using python | Fun python projects for beginners | Cool projects ?
Education
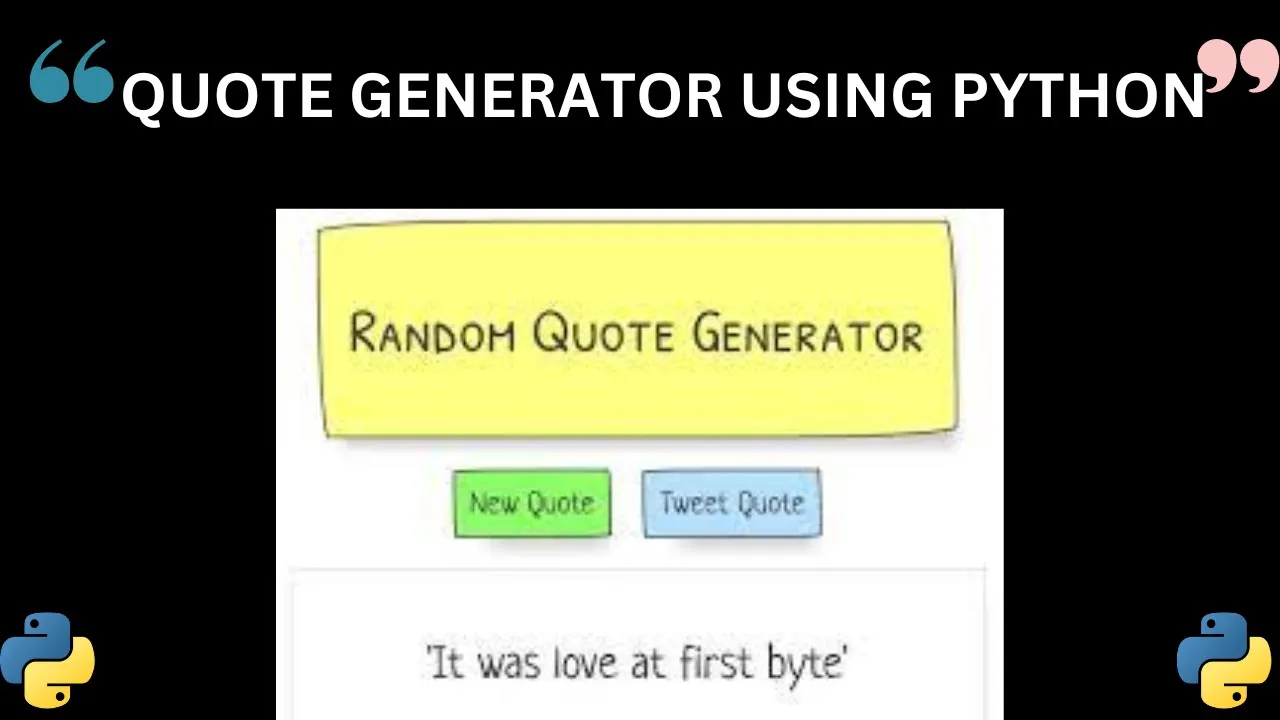
Introduction
Creating small projects in Python can be both fun and educational. In this article, we will walk you through how to develop a simple quote generator using the random
library. This project will help you enhance your Python skills and allow you to experiment with basic programming concepts.
Step-by-Step Guide to Creating a Quote Generator
Step 1: Importing the Random Library
First, to utilize randomness in your quote generator, you need to import the random
library. This library will allow us to randomly select quotes from a predefined list.
import random
Step 2: Defining a List of Quotes
Next, we will create a variable called codes
that stores a collection of inspirational quotes. You can include as many quotes as you want. For instance, here are a few motivational quotes:
codes = [
"Innovation distinguishes between a leader and a follower. - Steve Jobs",
"The future belongs to those who believe in the beauty of their dreams.",
"The only limit to the realization of tomorrow is our doubts of today.",
"In the end, we will remember not the words of our enemies, but the silence of our friends. - [Martin Luther King Jr](https://www.topview.ai/blog/detail/Martin-Luthor-King-Jr-).",
"The way to get started is to quit talking and begin doing. - Walt Disney"
]
Step 3: Randomly Selecting a Quote
After defining the list, we will create another variable called random_code
that will use the random.choice
method to select a quote from our list randomly. Finally, print out the selected quote.
random_code = random.choice(codes)
print(random_code)
Step 4: Running the Program
Now that your code is set up, you can run the program to see the output. Each time you execute it, you should get a different quote randomly selected from your list.
## Introduction
## Introduction
Step 5: Testing the Randomness
To ensure that your generator works as intended, run the program multiple times. You should observe a variety of quotes, verifying that the randomness is functioning properly.
Conclusion
Congratulations! You have successfully built a simple quote generator using Python. Feel free to expand the project by adding more quotes or even creating a graphical user interface (GUI) to improve user interactivity.
Keyword
- Python
- random library
- quote generator
- inspirational quotes
- random selection
- programming project
- beginners
FAQ
Q: What is the purpose of the random library in Python?
A: The random library allows you to perform random operations, such as generating random numbers or selecting random items from a list.
Q: How do I add more quotes to the generator?
A: You can simply add more strings to the codes
list in the same format as the existing quotes.
Q: Can I modify the program to display quotes in a different format?
A: Yes, you can customize the output formatting in the print statement or add additional functionalities to enhance the user experience.
Q: What other projects can I create as a beginner in Python?
A: Some fun projects to consider include a to-do list app, a simple game, or a weather app that pulls data from an API.