How to use the ChatGPT API with Python!!
Science & Technology
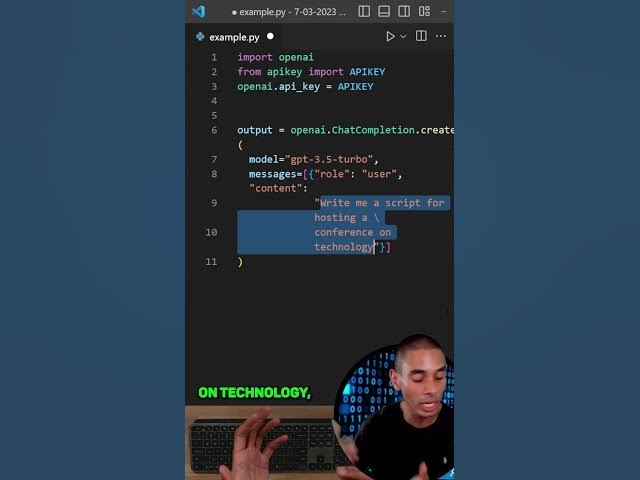
How to Use the ChatGPT API with Python
The recent release of the ChatGPT API has created a buzz in the tech community. Let’s delve into how you can utilize this API using Python. This guide will walk you through the process step-by-step.
Step 1: Importing the OpenAI Python Library and Setting Your API Key
First, you need to import the OpenAI Python library and set up your API key. You can find the API key directly in your OpenAI account.
Step 2: Using the openai.ChatCompletion.create
Method
To specify the model and pass through a prompt, use the openai.ChatCompletion.create
method. For instance, if you want to generate a script for hosting a technology conference, set the prompt accordingly.
import openai
openai.api_key = 'your-api-key-here'
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[
("role": "system", "content": "You are a helpful assistant."),
("role": "user", "content": "Write me a script for hosting a conference on technology.")
]
)
print(response.choices[0].message['content'])
Step 3: Print and Run the Output
Once the script is executed, the API provides a generated script for your specified task. This can significantly save you time and effort.
By running the script, you receive the generated output, which in this case, is the entire script for hosting a technology conference.
Conclusion
By following these steps, you can effectively leverage the ChatGPT API for various tasks. For example, generating content such as scripts can be automated, saving you precious time for other important duties. If you want to dive deeper, the code is available for you to try.
Keywords
- OpenAI
- ChatGPT API
- Python
openai.ChatCompletion.create
- API Key
- Script Generation
FAQ
Q1: How do I obtain my OpenAI API key?
A1: You can find your API key directly in your OpenAI account under the API section.
Q2: What is the purpose of the openai.ChatCompletion.create
method?
A2: The openai.ChatCompletion.create
method is used to specify the model and pass prompts for generating content or responses using the ChatGPT API.
Q3: Can I use this API for tasks other than generating scripts?
A3: Yes, the ChatGPT API can be used for a wide range of tasks including but not limited to generating articles, answering questions, and building conversational agents.
Q4: Is the output customizable based on different prompts?
A4: Absolutely, the output can be tailored based on the prompts you provide, allowing for versatile applications.
Q5: Where can I find more examples and documentation?
A5: More examples and comprehensive documentation can be found on the OpenAI API documentation.