How to visualise a Knowledge Graph
Science & Technology
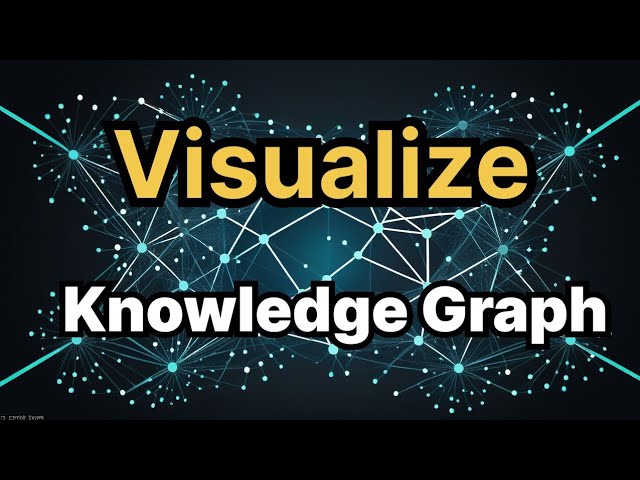
Introduction
Introduction
Hello everyone! Today's focus is on demonstrating how to visualize a knowledge graph that you've created using a large language model (LLM), which you might be using for Graph Retrieval-Augmented Generation (Graph RAG). Let's dive in!
Prerequisites
Before jumping into the visualization part, I assume you are already familiar with creating knowledge graphs using LangChain
. If not, make sure to check out my previous video on knowledge graph creation using LangChain.
First, install the necessary libraries via pip:
pip install langchain google-generativeai langchain-core JsonRepair networkx
Libraries and Versions
- LangChain: Specific version
- Google Generative AI: Specific version if using the free API key
- LangChain Core
- JsonRepair
- NetworkX: This will be particularly important for visualization.
Creating the Knowledge Graph
We will be using the Google Generative AI and LangChain libraries to set up the text for our knowledge graph.
Here is an example text about Marie Curie:
Marie Curie, born in 1867, was a physicist and chemist who was awarded the Nobel Prize.
We will transform this text into a knowledge graph using the convert_to_graph_documents
function from the LangChain
library.
Saving Relationships to a DataFrame
Save the relationships formed from the graph document into a DataFrame with columns: node1
, node2
, and relationship
. Here's a basic skeleton of what the DataFrame might look like:
node1 | node2 | relationship |
---|---|---|
Marie Curie | 1867 | born in |
Marie Curie | physicist | occupation |
Marie Curie | Nobel Prize | awarded Nobel Prize |
Visualizing the Knowledge Graph
Using NetworkX
We'll be utilizing the NetworkX
library for graph visualization. Follow these steps:
Create a Directed Graph Object:
import networkx as nx G = nx.DiGraph()
Add Nodes and Edges to the Graph:
Read each row in the DataFrame and add the nodes (
node1
,node2
) and the relationship as the edge.for index, row in df.iterrows(): G.add_edge(row['node1'], row['node2'], relationship=row['relationship'])
Set Up Layout for Visualization:
import matplotlib.pyplot as plt pos = nx.spring_layout(G)
Drawing the Graph:
edge_labels = nx.get_edge_attributes(G, 'relationship') plt.figure(figsize=(12, 8)) nx.draw(G, pos, with_labels=True, node_color='skyblue', node_size=2000, edge_color='gray', font_size=15, width=2, arrows=True) nx.draw_networkx_edge_labels(G, pos, edge_labels=edge_labels, font_color='red') plt.title("Graph Visualization") plt.show()
Conclusion
And that's how you can visualize a knowledge graph created using an LLM! We’ve covered the setup, conversion into a DataFrame, and visualization using NetworkX. I hope this tutorial helps you extend your work to build a Graph RAG and more.
Thank you for your time. The Graph RAG crash course is out now, which includes all the codes, a theory eBook, and links to video tutorials. Check out the link for the course in the description below.
Keywords
- Knowledge Graph
- LangChain
- NetworkX
- Large Language Model (LLM)
- Text Visualization
- Graph RAG
- Google Generative AI
- DataFrame
- Directed Graph
- Graph Visualization
- Python
FAQ
1. What libraries do I need to install for visualizing a knowledge graph?
You need to install langchain
, google-generativeai
, langchain-core
, JsonRepair
, and networkx
.
2. How do I create a knowledge graph using LangChain?
You can create a knowledge graph by using Google Generative AI and LangChain libraries, and the convert_to_graph_documents
function.
3. How can I save the relationships in the knowledge graph?
Save the relationships into a DataFrame with columns node1
, node2
, and relationship
.
4. What library is used for visualizing the graph?
We use NetworkX
for visualizing the graph.
5. What is an edge in the context of NetworkX?
An edge in NetworkX has three components: the two nodes it connects and the relationship, which serves as the edge label or name.