How We Built LlamaCoder (400k Users) – A Full-Stack Next.js AI App
Science & Technology
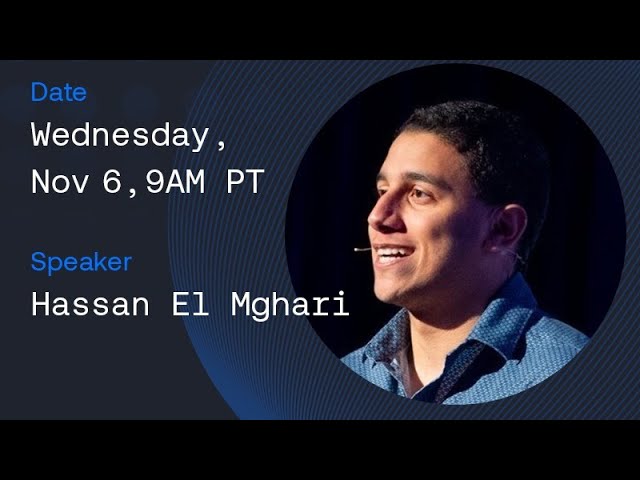
Introduction
Welcome to this article where we delve into the fascinating journey of building LlamaCoder, an innovative AI application that has attracted over 400,000 users. In this article, we will utilize a practical live coding approach to demonstrate how to create a Next.js full-stack application that leverages AI capabilities.
Introduction
LlamaCoder is a unique web application that turns user ideas into interactive applications. By simply prompting the system with an idea, users can generate functional apps. In this tutorial, we will take you through the process of building such an application from scratch, discussing both the front-end and back-end architecture along the way.
Setting Up the Environment
To get started, we’ll create a new Next.js app. Using the terminal, the following command sets the foundation for our application:
npx create-next-app@latest llama-coder-clone
During the setup process, we’ll opt for TypeScript and Tailwind CSS to streamline our development and styling.
App Structure Overview
Next.js has a wonderful structure to manage both front-end and back-end functionalities in one framework:
- The pages.tsx file in the
app
folder handles the front-end and is built using React-based syntax. - The
API
folder is where we define back-end API routes, similar to using serverless functions.
Building the Front-End
Starting with our front end, we established a basic layout featuring a header and an input field for users to type their prompts:
<div className="max-w-5xl mx-auto">
<h1 className="text-3xl font-bold">LlamaCoder Clone</h1>
<input type="text" placeholder="Put in your prompt" className="input-style"/>
</div>
This input field is bound to React state, allowing us to capture user input dynamically.
Implementing the Back-End API
For the back end, we create a new API route in our Next.js application under API/generateCode/route.ts
. This route will handle our application logic by receiving the user prompt and processing it:
export async function POST(request: Request) (
const data = await request.json();
const prompt = data.prompt;
console.log(prompt);
return new Response("Done");
)
Here, we’re simply logging the prompt to the console for now.
Integrating Together AI
Next, we integrate the Together AI SDK to convert user prompts into executable code. Setting up the SDK is as simple as running:
npm install together-ai
Following that, we instantiate a new client in our back-end function. The SDK allows us to generate code with Llama 3.1 405B, one of the top open-source coding models.
Streaming Code Response
A key feature of our application is the ability to stream back the generated code. We use the ReadableStream
to give immediate feedback as the AI processes the request, similar to how chatbots work.
const stream = together.chat.completions.stream();
return new Response(stream.toReadableStream());
This streaming mechanism greatly enhances user experience, providing near-instantaneous results.
Rendering the Generated Code
On the front-end, we utilize the Sandpack component to render the generated code. Sandpack serves as a mini code editor allowing users to view and interact with the output:
<Sandpack
template="react-ts"
options=({
editorHeight: '80vh',
externalResources: ['https://tailwindcss.com']
)}
/>
By conditionally rendering Sandpack only when there is generated code, we elegantly display the result of the user’s request.
Performance Statistics
As of recent reports, LlamaCoder has had roughly 436,000 visitors within a few months. This success has led to more than 1 million requests via our API, showcasing the application’s popularity and utility.
Conclusion
Creating LlamaCoder exemplifies how modern developers can leverage AI to build functional applications quickly. This project demonstrates the capability of Next.js to handle both front-end and back-end processes, combined with the power of Together AI to turn user prompts into immediate code solutions.
Encouragingly, many more developers can create innovative AI applications of their own.
Keyword
- LlamaCoder
- Next.js
- AI application
- Full-stack
- Together AI
- TypeScript
- Sandpack
- Real-time streaming
- Open-source models
FAQ
1. What is LlamaCoder?
LlamaCoder is an AI application that generates interactive apps based on user prompts.
2. What technology stack was used to build LlamaCoder?
The application was built using Next.js for the full-stack framework, TypeScript for type safety, and Tailwind CSS for styling.
3. How does the code generation process work?
When a user inputs their prompt, it is sent to Together AI's SDK, which leverages powerful open-source coding models to generate functional code as a response.
4. Can the generated code be tested in real-time?
Yes, the Sandpack component allows users to view and edit the generated code in a live code editor environment.
5. Where can I find the source code for LlamaCoder?
The LlamaCoder app is open-source, and the code can be found on GitHub. A link will be provided for further exploration.