Image Classification Project in Python | Deep Learning Neural Network Model Project in Python
Education
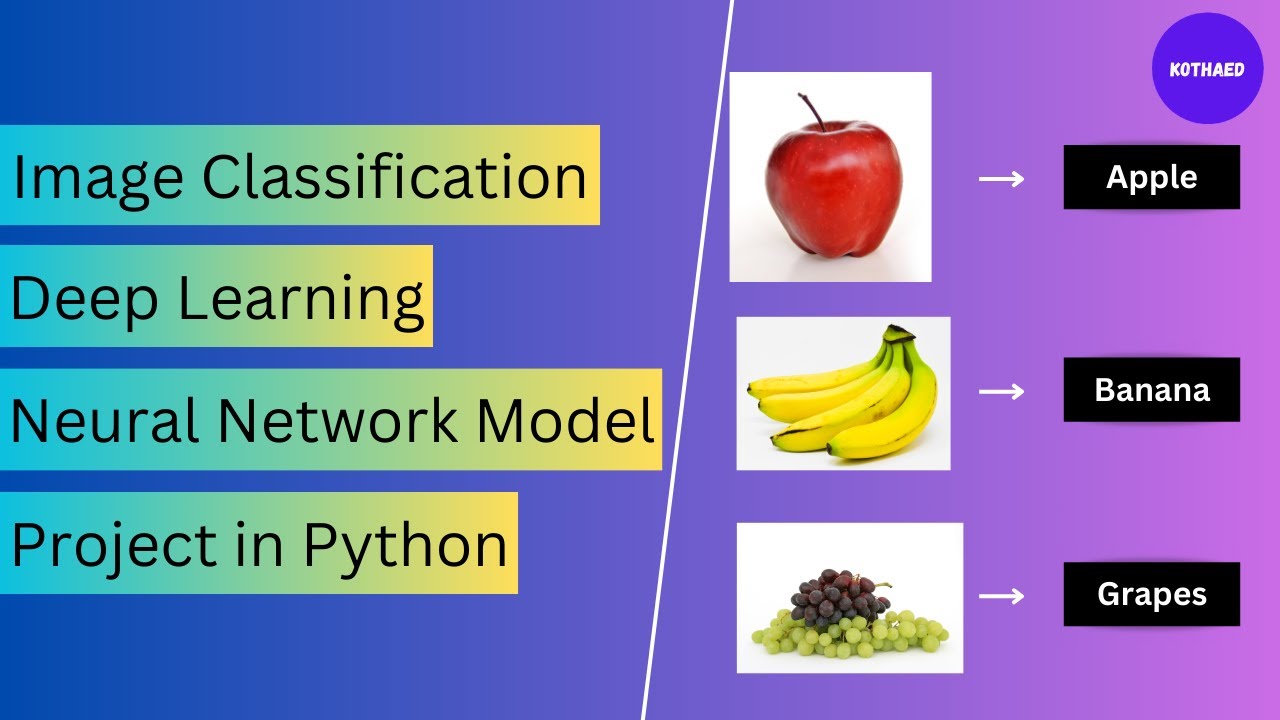
Introduction
Introduction
In this article, we will take a closer look at building an image classification model from scratch using Python's TensorFlow library. The goal of this project is to create a model that can distinguish between fruits and vegetables by analyzing image data. After successfully training our model, we'll go through the steps of testing it with new images and even deploying it as a web application.
Project Overview
Our final image classification model will take an image name as input and identify whether the displayed image is a fruit or a vegetable. For example, given the image of an apple, the model could output “The image is apple with an accuracy of 99.95%”. Similarly, other images can be tested using the same methodology.
Setting Up the Environment
To build our deep learning model, we will use the TensorFlow and Keras libraries. TensorFlow is developed by Google and provides an efficient interface for creating machine learning models with ease.
Required Libraries
We first need to install a few essential Python libraries via the command line or terminal:
pip install tensorflow streamlit matplotlib pandas
Data Preparation
Our images of fruits and vegetables are organized into folders within three datasets: training, test, and validation. Each subfolder contains relevant images in separate categories corresponding to their respective fruit or vegetable.
- Training Dataset: Contains images used to train the model.
- Validation Dataset: Contains images used to validate the model's performance during training.
- Test Dataset: Contains completely new images used to evaluate the model after training.
Loading the Data
We will utilize the ImageDataGenerator
class from TensorFlow to help preprocess the images. Images will be resized to 180x180 pixels, and we will use one-hot encoding for our labels.
Data Augmentation and Rescaling
As part of preprocessing, we will rescale pixel values from a range of 0-255 to 0-1, which helps improve model accuracy:
image_datagen = ImageDataGenerator(rescale=1./255)
Model Development
Creating the Neural Network
We will utilize a sequential model in Keras. The model will consist of multiple layers, including convolutional, pooling, and dropout layers to help with learning feature representations from the images. The key components include:
- Convolutional Layers: Apply filters to the input data to capture patterns.
- Pooling Layers: Reduce dimensionality, while retaining important features.
- Dense Layers: Fully connected layers for classification tasks.
Compiling the Model
After building the model architecture, we will compile it using an optimizer such as Adam, a loss function like categorical crossentropy, and a metric to evaluate accuracy.
Training the Model
We will then fit our model to the training data for a specified number of epochs while validating it against our validation dataset. By monitoring the training process, we can ensure that the model learns effectively without overfitting.
Evaluating the Model
After training is complete, we can visualize the accuracy and loss over the epochs to understand how the model performed.
Testing the Model
With the model trained, we can evaluate its performance on unseen data by measuring its accuracy against test images. For instance, running the model on an image of a banana may yield an output of "The image is banana with an accuracy of 46.91%."
Deploying the Model
Finally, we will deploy our model into a streamlit web application. This allows users to upload images and receive predictions in real time. After saving our trained model, we will import it into our streamlit app and set up a simple interface for image classification.
Conclusion
By following the steps outlined, we have successfully created a Python image classification model using TensorFlow. This project provides a solid foundation for anyone interested in exploring deeper into the world of machine learning and image analysis.
Keywords
- Image classification
- TensorFlow
- Keras
- Neural networks
- Fruits and vegetables
- Model training
- Data augmentation
- Streamlit
FAQ
Q1: What is image classification?
A1: Image classification is the process of assigning a label to an image based on its content. In this project, we classify images as either fruits or vegetables.
Q2: Why do we use data validation?
A2: Data validation ensures that our model remains generalizable and performs well on new, unseen data rather than just fitting to the training data.
Q3: What is TensorFlow?
A3: TensorFlow is an open-source machine learning library developed by Google that enables easy development and training of models for various machine learning tasks.
Q4: How can we evaluate the performance of the model?
A4: The model's performance can be evaluated using metrics like accuracy over a validation dataset and visualizing training and validation loss/accuracy graphs.
Q5: Can this model be deployed?
A5: Yes, the trained model can be deployed as a web application using tools like Streamlit, which provides a simple interface for image classification.