Intelligent AI Chatbot in Python
Science & Technology
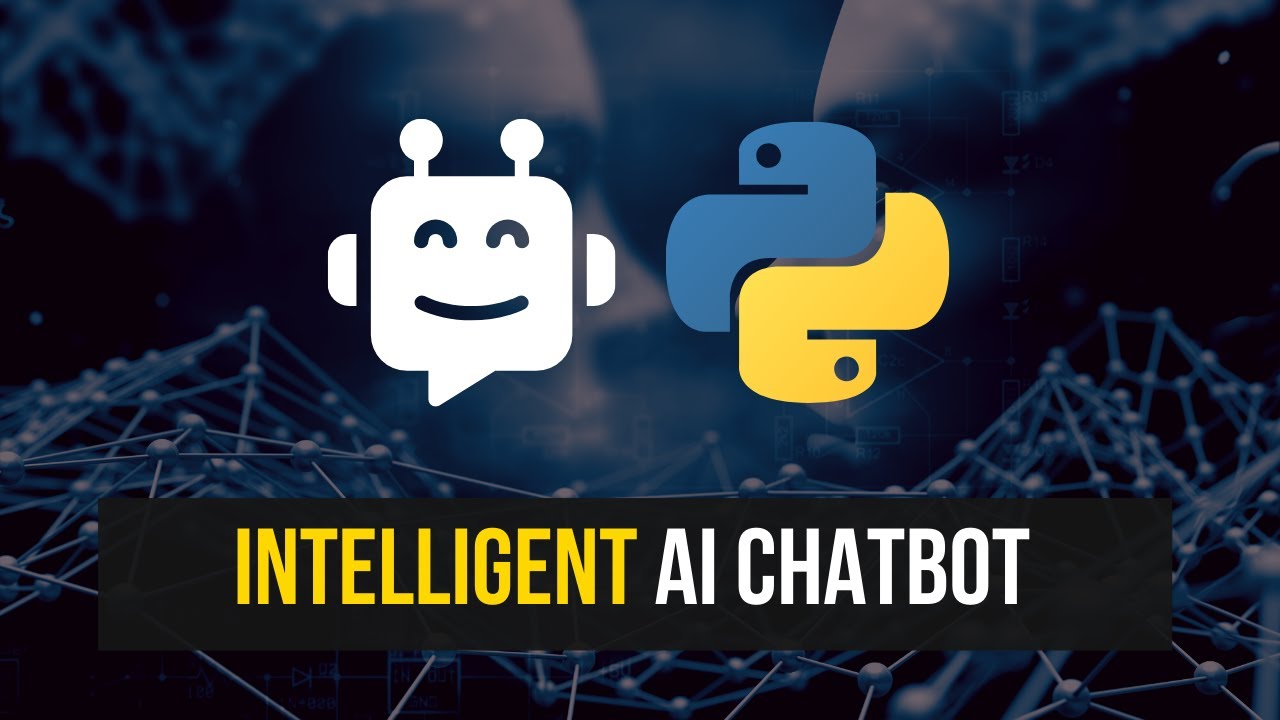
Introduction
In this tutorial, we're going to build a chatbot using natural language processing and neural networks in Python. We'll start by setting up an intents.json file, which contains different categories of user inputs and corresponding responses for the chatbot. Each category includes patterns (possible user inputs) and responses (predefined bot responses). We'll train the chatbot using this intents.json file and then build a chatbot application using the trained model.
Step 1: Preprocessing and Training
First, we need to preprocess the data and train the chatbot model. We do the following steps:
- Import the necessary libraries: random, json, pickle, numpy, nltk, and tensorflow.keras.
- Load and lemmatize the training data from the intents.json file.
- Create bags of words and prepare the training dataset.
- Build a sequential model with dense layers, dropout layers, and the softmax activation function.
- Compile and train the model using the training dataset with 200 epochs and a batch size of 5.
- Save the trained model, words, and classes into separate pickle files.
Step 2: Building the Chatbot Application
Now, let's build the chatbot application using the trained model and the pickle files. We do the following steps:
- Import the necessary libraries: random, json, pickle, numpy, nltk, and tensorflow.keras.
- Load the words, classes, and model from the pickle files.
- Define helper functions for cleaning up sentences, creating a bag of words, predicting the class based on the input sentence, and getting a response from the chatbot.
- Run the chatbot application and interact with it by inputting sentences.
Keyword
neural networks, natural language processing, chatbot, Python, intents.json, preprocessing, training, sequential model, dense layers, dropout layers, softmax activation function, bag of words, prediction, response.
FAQ
What is a chatbot?
- A chatbot is an AI program designed to simulate conversation with human users. It can respond to user queries and engage in natural language dialogue.
How does natural language processing work in chatbots?
- Natural language processing (NLP) is a branch of AI that focuses on the interaction between computers and human language. In chatbots, NLP helps analyze and interpret user inputs to generate appropriate responses.
How are neural networks used in chatbots?
- Neural networks are used in chatbots to process and understand user inputs. They learn from training data, such as patterns of user queries and associated responses, and make predictions based on that learning to generate appropriate replies.
What is preprocessing in chatbot training?
- Preprocessing involves transforming raw text data into a format suitable for training a chatbot. This includes tasks like tokenization, lemmatization, and creating bags of words to represent the input data numerically.
How can I improve my chatbot's performance?
- You can improve a chatbot's performance by providing more training data, adding more patterns and responses to the intents.json file, and fine-tuning the neural network model. Testing and iterating based on user feedback can also help improve the chatbot's performance over time.