LangChain - Conversations with Memory (explanation & code walkthrough)
Science & Technology
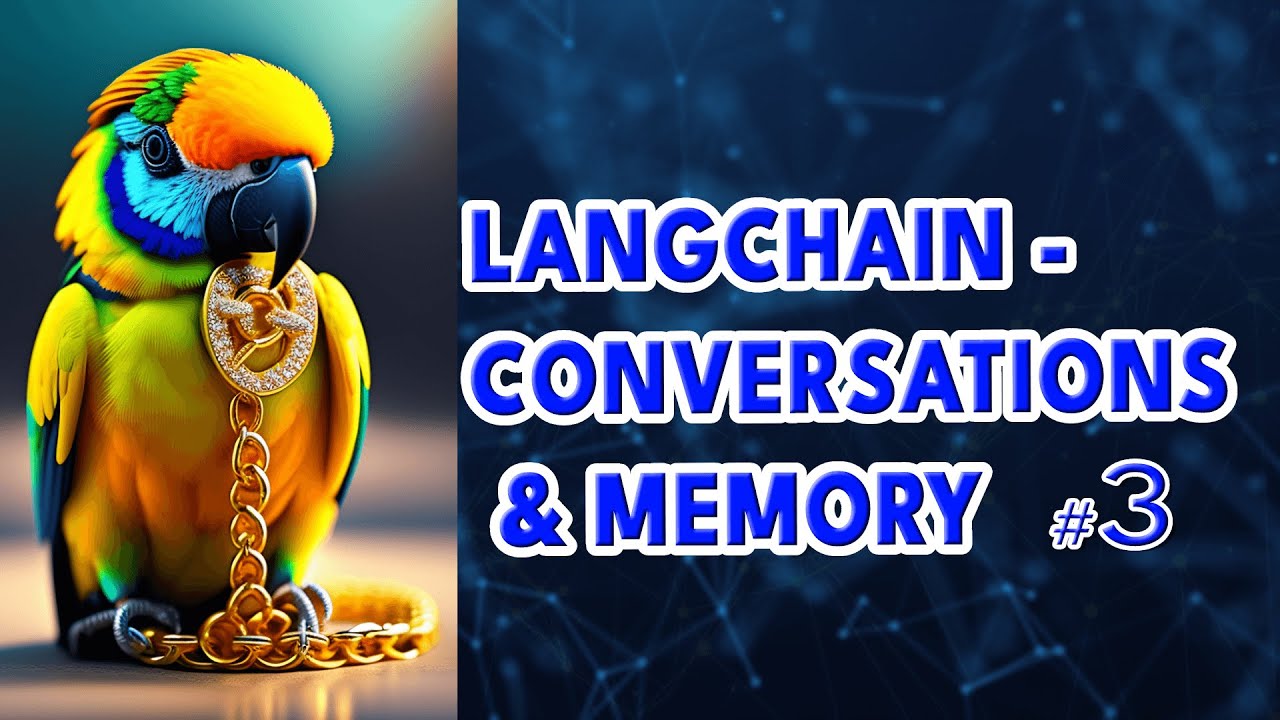
Introduction
In this article, we will explore the importance of memory in building conversational agents with LangChain. As chatbots are expected to exhibit human-like qualities, incorporating memory into their design becomes crucial. This allows chatbots to refer back to previous interactions, facilitating smoother dialogues and minimizing user frustration.
Why is Memory Important?
When users interact with a chatbot, they often treat it as though it possesses human-like understanding and memory. This expectation can lead to frustration when the bot fails to recall prior interactions. Memory significantly enhances the chatbot's ability to reference earlier parts of the conversation, such as places, times, and even co-reference resolution. For instance, if a user mentions a person by name and later refers to them as "he" or "she," memory allows the bot to resolve this co-reference and provide contextually relevant responses.
Large language models (LLMs), by their nature, do not possess memory. They generate responses based solely on the prompts they receive at each instance. Although efforts have been made to develop Transformer models with integrated memory, an efficient, scalable solution remains elusive. Currently, there are two main strategies for managing memory in LLMs: incorporating memory directly into the prompt or utilizing an external lookup.
Memory Management Options
Embedding Memory in Prompts: Using this approach, the entire conversation history can be included in the prompt. However, this poses a challenge owing to the token limit—recent LLMs may handle about 4096 tokens, which isn't sufficient for long conversations.
External Lookup: In forthcoming updates to LangChain, we will explore how to utilize databases or other methods to store and retrieve information, enhancing conversational capabilities.
Types of Memory in LangChain
LangChain provides various memory storage implementations:
- Conversation Buffer Memory: This simple memory type continuously stacks conversation exchanges, allowing the agent to track dialogues easily.
- Conversation Summary Memory: Instead of storing the entire conversation, this memory type summarizes interactions, conserving token usage over time.
- Conversation Buffer Window Memory: This variant keeps only the last few interactions, thus maintaining a manageable token count while allowing for contextual dialogues.
- Combined Summary and Buffer Memory: This combines previous techniques, maintaining both a summary of earlier steps and the most recent conversation interactions.
- Knowledge Graph Memory: This represents entities within conversations graphically, facilitating easy reference and relevance to user queries.
- Entity Memory: Specifically targets the extraction of known entities for easy retrieval and contextual continuity.
Code Walkthrough
Let's explore how these memory techniques can be implemented in LangChain. We begin with the standard setup for initializing the necessary libraries:
## Introduction
$ pip install openai langchain
## Introduction
openai.api_key = "YOUR_API_KEY"
Conversation Buffer Memory
from langchain.memory import ConversationBufferMemory
from langchain import ConversationalChain
memory = ConversationBufferMemory()
conversation_chain = ConversationalChain(memory=memory, verbose=True)
## Introduction
response = conversation_chain.predict("Hi there, I am Sam.") # Agent responds
Conversation Summary Memory
from langchain.memory import ConversationSummaryMemory
summary_memory = ConversationSummaryMemory()
conversation_chain = ConversationalChain(memory=summary_memory, verbose=True)
response = conversation_chain.predict("Hi there, I am Sam.")
Conversation Buffer Window Memory
from langchain.memory import ConversationBufferWindowMemory
window_memory = ConversationBufferWindowMemory(k=2) # Keeps the last 2 interactions
conversation_chain = ConversationalChain(memory=window_memory, verbose=True)
response = conversation_chain.predict("Hi there, I am Sam.")
Knowledge Graph Memory
from langchain.memory import KnowledgeGraphMemory
kg_memory = KnowledgeGraphMemory()
conversation_chain = ConversationalChain(memory=kg_memory, verbose=True)
response = conversation_chain.predict("Hi there, I am Sam.")
Entity Memory
from langchain.memory import EntityMemory
entity_memory = EntityMemory()
conversation_chain = ConversationalChain(memory=entity_memory, verbose=True)
response = conversation_chain.predict("Hi there, I am Sam.")
With these memory types implemented in LangChain, chatbots can generate more contextually aware interactions, enhancing user experience.
Keywords
- LangChain
- Memory
- Conversation Buffer Memory
- Conversation Summary Memory
- Entity Memory
- Knowledge Graph Memory
FAQ
Q: Why is memory necessary in conversational agents?
A: Memory is crucial as it allows chatbots to reference previous conversations, which enhances user experience and minimizes frustration.
Q: What are the different memory options available in LangChain?
A: LangChain offers several memory options, including Conversation Buffer Memory, Conversation Summary Memory, and Entity Memory, among others.
Q: How does Talk function with external databases?
A: Future videos will address how to use external databases for lookup, helping retain conversation context beyond immediate token limits.
Q: Can I implement custom memory solutions in LangChain?
A: Yes, LangChain allows users to create and integrate their own custom memory management systems.
This article covers the principles and implementations of memory in conversational AI using LangChain, providing insights into building more effective chat agents. If you have any questions, feel free to reach out or leave a comment.