Less than 1 minute
People & Blogs
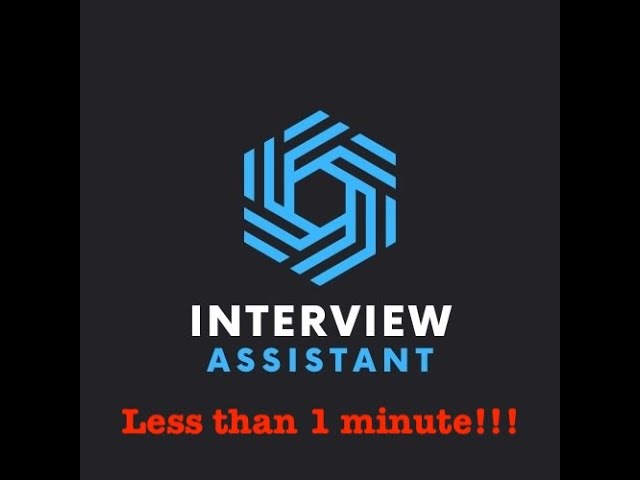
Introduction
In this article, we will design a class in Python that supports three specific operations: inserting a value, removing a value, and retrieving a random value with equal probability from a set of integers. The functionality will ensure that duplicates are not stored, and each operation will be efficient enough to handle a reasonable number of elements.
Class Design
To create the class, we will use two main data structures: a hashmap (dictionary in Python) and a list. The hashmap will store the values along with their indices, while the list will maintain the order of the inserted values, making it easy to fetch random values.
Constructor
We will begin with the constructor, initializing the necessary components:
map
: This will be a dictionary to store the values as keys and their indices in the list as the corresponding values.values
: This will be a list to keep track of the inserted values.
Insert Method
To insert an integer:
- Check if the integer already exists in the
map
. If it does, return without inserting to avoid duplicates. - If it doesn't, append the integer to the
values
list and store its index in themap
.
def insert(self, value):
if value in self.map:
return
self.map[value] = len(self.values)
self.values.append(value)
Remove Method
To remove an integer:
- Check if the integer exists in the
map
. If not, return without removing. - If it exists, find its index in the
values
list. - Swap the last element with the one to be removed to maintain the integrity of the list.
- Remove the last element and update the
map
accordingly.
def remove(self, value):
if value not in self.map:
return
idx = self.map[value]
last_value = self.values[-1]
self.values[idx] = last_value
self.map[last_value] = idx
self.values.pop()
del self.map[value]
Get Random Method
To retrieve a random integer:
Utilize random.choice
to return a random element from the values
list.
import random
def get_random(self):
return random.choice(self.values)
After implementing this class, we will have efficient insert, remove, and get random functionalities, accommodating the requirements laid out at the beginning.
Keywords
Class design, insert operation, remove operation, get random operation, hashmap, list, Python, efficiency, integers.
FAQ
Q1: What operations does this class support?
- The class supports inserting a value, removing a value, and retrieving a random value.
Q2: Can this class handle duplicate values?
- No, the class is designed to avoid storing duplicate values.
Q3: How efficient are the operations?
- The insert and remove operations have an average time complexity of O(1), and getting a random value also operates at O(1).
Q4: What data type does the class handle?
- The class is designed to handle integers.