Merge Sort | Code and Explanation | C++ Course - 19.1
People & Blogs
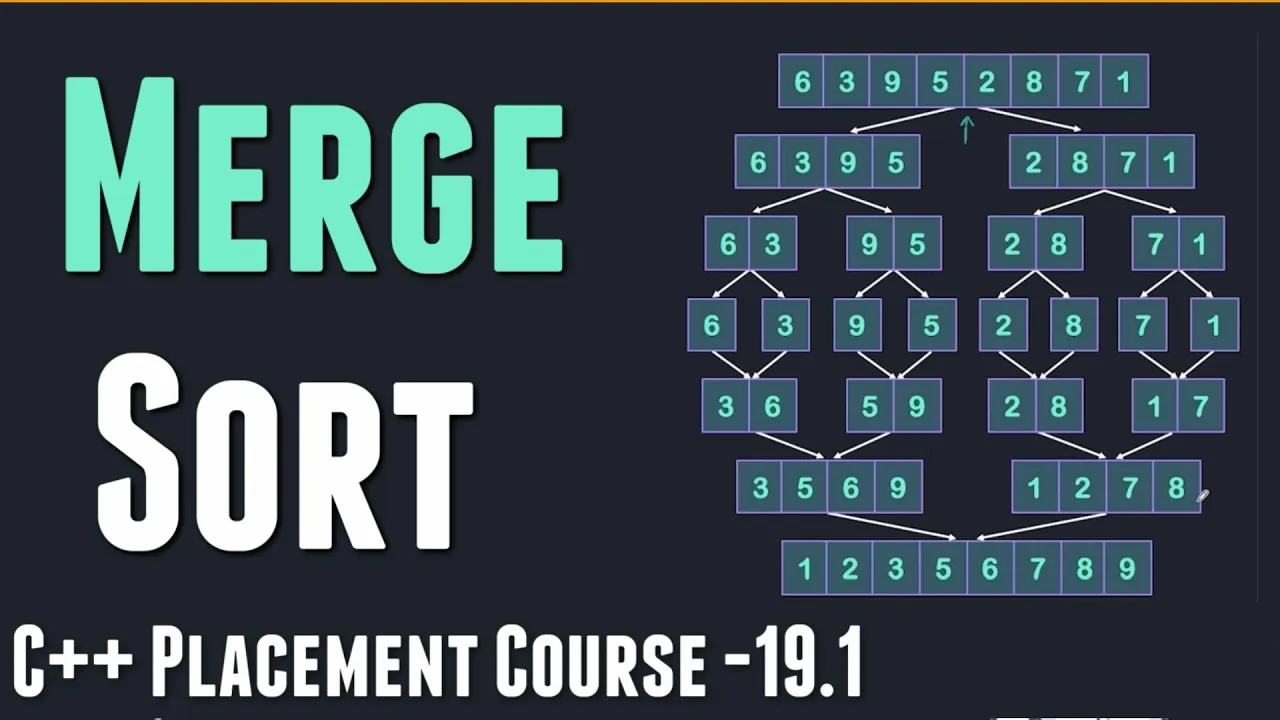
Introduction
In this article, we will delve into the concept of Merge Sort and understand how it works through a step-by-step explanation. We will also explore the code implementation in C++ for better understanding.
Code Explanation
The Merge Sort algorithm follows a divide and conquer approach to solve a problem. It breaks down the problem into smaller sub-problems until we have small enough sub-problems to directly solve them.
To begin with, we determine the midpoint of the given array and divide it into two halves. We then recursively call the Merge Sort function on each half until we reach the base case with only one element.
Next, we merge the sorted halves back together. We compare the elements from both halves and put them in the correct order. This process is repeated until all the elements are sorted.
Finally, we obtain the final sorted array as the result of the Merge Sort function.
Code Implementation
void merge(int arr[], int left[], int right[], int leftSize, int rightSize) (
int i = 0; // Index for left array
int j = 0; // Index for right array
int k = 0; // Index for merged array
// Compare and merge elements from left and right arrays
while (i < leftSize && j < rightSize) {
if (left[i] <= right[j]) {
arr[k] = left[i];
i++;
)
else (
arr[k] = right[j];
j++;
)
k++;
}
// Copy remaining elements from left array
while (i < leftSize) (
arr[k] = left[i];
i++;
k++;
)
// Copy remaining elements from right array
while (j < rightSize) (
arr[k] = right[j];
j++;
k++;
)
}
void mergeSort(int arr[], int size) (
if (size <= 1) {
return; // Base case
)
int mid = size / 2;
int left[mid];
int right[size - mid];
// Divide the array into left and right halves
for (int i = 0; i < mid; i++) (
left[i] = arr[i];
)
for (int i = mid; i < size; i++) (
right[i - mid] = arr[i];
)
// Recursively call mergeSort on left and right halves
mergeSort(left, mid);
mergeSort(right, size - mid);
// Merge the sorted halves
merge(arr, left, right, mid, size - mid);
}
Keyword
Merge Sort, Divide and Conquer, Algorithm, Code, Explanation, C++
FAQ
- What is Merge Sort?
- Merge Sort is a sorting algorithm that follows the divide and conquer approach. It breaks down the problem into smaller sub-problems, sorts them individually, and then merges them to obtain the final sorted result.
- How does Merge Sort work?
- Merge Sort works by recursively dividing the given array into smaller halves until each sub-array has only one element. It then merges the sorted sub-arrays back together until the entire array is sorted.
- What is the time complexity of Merge Sort?
- The time complexity of Merge Sort is O(n*log(n)), where 'n' represents the number of elements in the array. This makes Merge Sort relatively efficient for sorting large datasets.
- Is Merge Sort a stable sorting algorithm?
- Yes, Merge Sort is a stable sorting algorithm. This means that elements with equal values maintain their relative order after the sorting process.
- How does Merge Sort compare to other sorting algorithms?
- Merge Sort has a consistent time complexity and is generally more efficient than other comparison-based sorting algorithms for large datasets. However, it requires additional space for the merging process compared to algorithms like Quicksort.