Natural Language to SQL | LangChain, SQL Database & OpenAI LLMs
Education
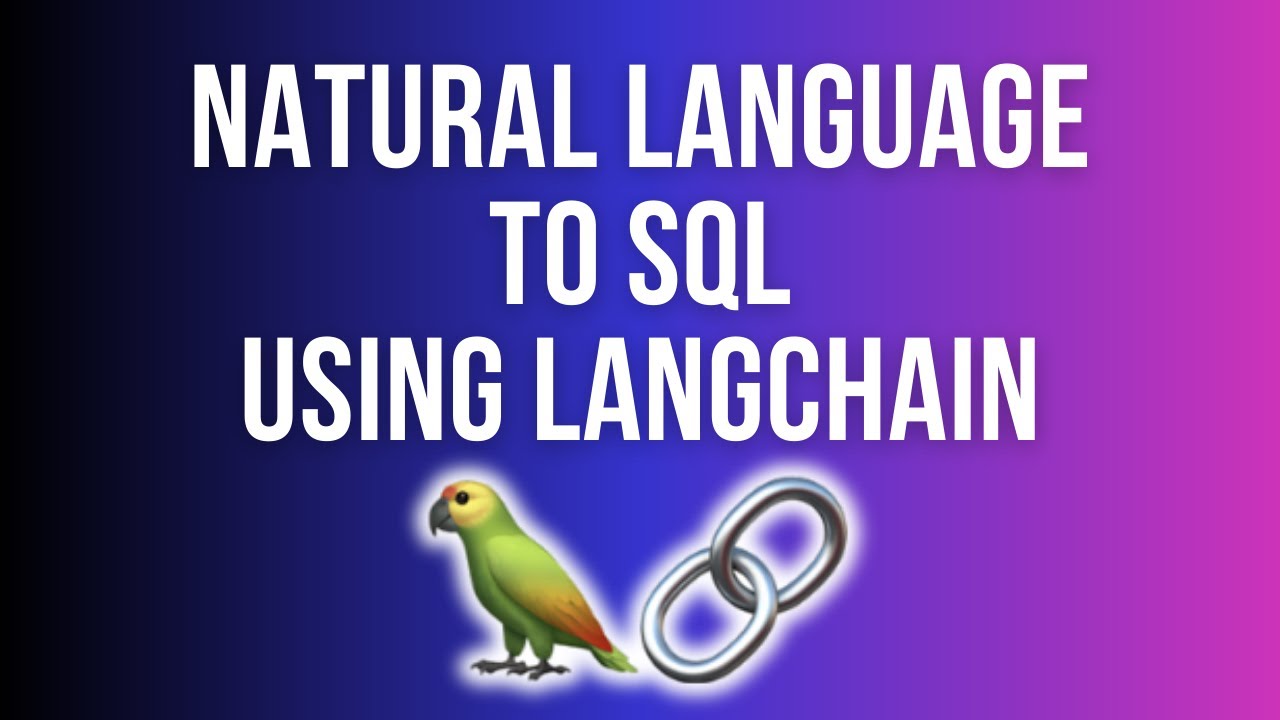
Natural Language to SQL | LangChain, SQL Database & OpenAI LLMs
In the previous video, I introduced a crucial tool called the Pandas DataFrame Agent. Today, we’ll explore a similar agent designed for SQL databases. In this article, I'll guide you on how to query SQL-based databases using natural language with LangChain. So, let's dive right in.
Step 1: Installation
First, we need to install LangChain and OpenAI:
pip install langchain openai
Once installed, make sure you have your OpenAI secret key ready. For security reasons, keep this key confidential.
Step 2: Preparing the SQL Database
If you have an existing SQL database, you can skip this step. If not, you can convert your CSV file into a SQL database using the following code:
## Introduction
import pandas as pd
import sqlite3
df = pd.read_csv('your_file.csv')
conn = sqlite3.connect('your_database.sqlite')
df.to_sql('your_table', conn, if_exists='replace', index=False)
Here’s a simple function to read the SQL database:
import sqlite3
def read_sql_query(query, db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute(query)
for row in cursor.fetchall():
print(row)
conn.close()
You can test it with:
read_sql_query('SELECT * FROM fashion_products LIMIT 10;', 'fashion_db.sqlite')
Step 3: LangChain Integration
LangChain makes it easy to convert natural language queries to SQL. Here’s how to get started:
from langchain import OpenAI
from langchain.chains.sql_db import SQLDatabase
from langchain.chains.sql_db import SQLDatabaseChain
## Introduction
input_db = SQLDatabase.from_uri('sqlite:///fashion_db.sqlite')
## Introduction
llm = OpenAI()
## Introduction
dbagent = SQLDatabaseChain(llm=llm, database=input_db, verbose=True)
Step 4: Querying the Database
Now, you can query your database using natural language:
Number of Rows:
result = dbagent.run("How many rows are there?") print(result)
Entries of a Specific Brand:
result = dbagent.run("How many entries of Adidas are present?") print(result)
Specific Product Details:
result = dbagent.run("How many XL products of Nike have a rating of more than 4?") print(result)
Filtered Product Details:
result = dbagent.run("Give all the details of Adidas which have a size of large and have a rating of more than 4.2.") print(result)
Conclusion
LangChain has significantly simplified the process of querying SQL databases using natural language. It’s incredible how seamlessly it transforms natural language queries into accurate SQL commands, making it an invaluable tool for both developers and businesses.
By leveraging LangChain, companies can vastly improve the accessibility and usability of their data, making data-driven decisions easier and more intuitive.
Keywords
- LangChain
- OpenAI
- SQL Database
- Natural Language Processing (NLP)
- Querying Databases
- Large Language Model (LLM)
- SQL to Natural Language
FAQ
Q: What is LangChain?
A: LangChain is a tool that allows you to create agents that can interact with various data sources like SQL databases via natural language queries.
Q: How does LangChain transform natural language into SQL queries?
A: LangChain uses large language models, like those from OpenAI, to interpret natural language and generate appropriate SQL queries.
Q: Do I need to know SQL to use LangChain?
A: No, LangChain simplifies interaction with databases to the point where you can query them using familiar natural language.
Q: How secure is it to use my OpenAI secret key?
A: Always keep your OpenAI secret key confidential and avoid sharing it publicly. LangChain allows you to integrate OpenAI capabilities securely within your private environment.
Q: Can LangChain handle complex SQL queries?
A: Yes, LangChain can handle complex SQL queries by converting detailed natural language questions into accurate SQL commands.