Playwright - How to pass/share data between tests in JavaScript
Science & Technology
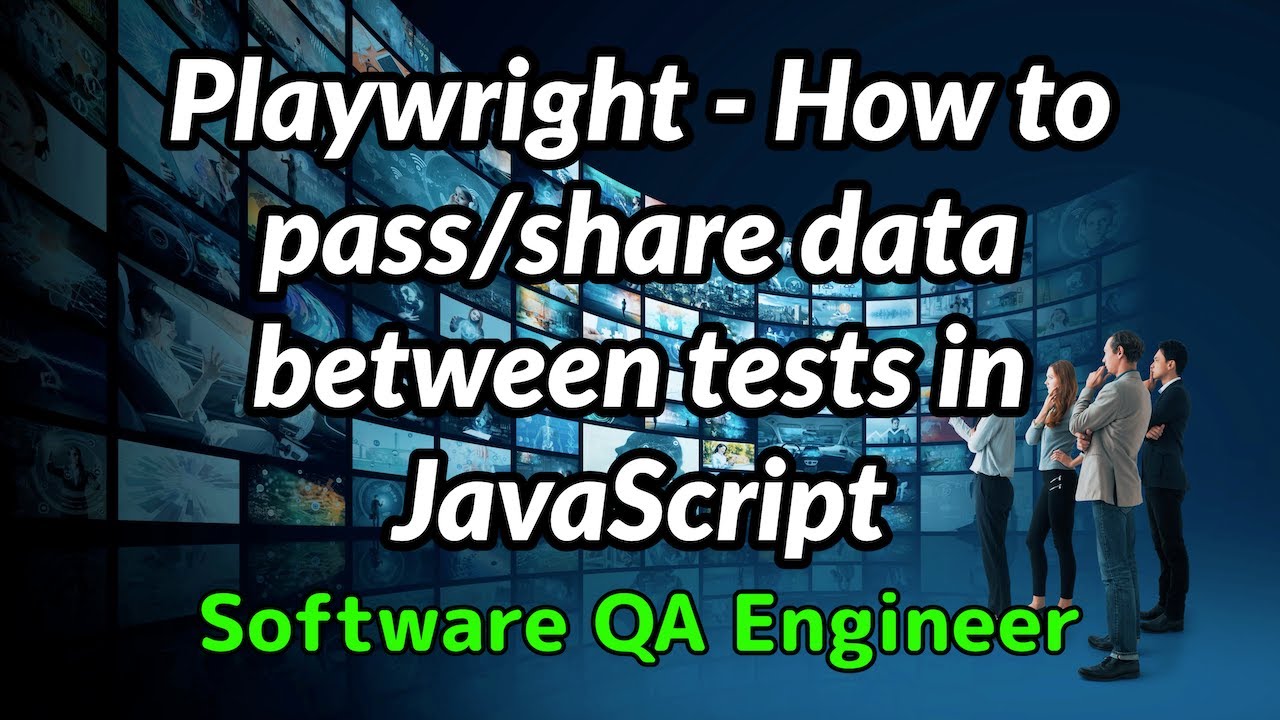
Introduction
When working with Playwright for testing applications, especially when executing tests in a sequential manner, it’s essential to share or pass data between tests. This can enhance efficiency, particularly when multiple tests require the same data—like authorization tokens or booking IDs. In this article, we will explore several methods to accomplish this.
Declaring Variables for Sharing Data
The simplest way to share data between tests is by declaring variables at the top of your spec file. By using let
, you can create variables that are reassignable throughout your tests.
In this example, you’ll create several tests, some of which require the same authorization token while others may need the same booking ID. Here’s how to implement it:
Declare Variables: At the top of your spec file, declare variables to store necessary data such as the authorization token and booking ID.
let authToken = null; let bookingID = null;
Before All Hook: Utilize the
beforeAll
hook to assign values to these variables. For example, you can make a POST request to an API to fetch your authorization token.beforeAll(async () => ( const response = await api.post('/auth/login', { username, password )); authToken = response.token; });
Use in Tests: You can then use these variables in your tests (GET, POST, PUT, PATCH, DELETE), reusing the token and booking ID as required.
test('Create a booking', async () => ( const response = await api.post('/bookings', { // Booking data )); bookingID = response.id; });
Cleanup and Validation: After performing operations, such as creating or deleting a booking, you can validate the results (e.g., check if the response status code is 404 when trying to delete a non-existent booking).
Alternative Methods for Sharing Data
While using variables is the easiest approach, there are other options:
Environment Variables
You can use environment variables for sensitive data like usernames and passwords. This method avoids hardcoding secrets in your codebase. You can set these variables via the command line when executing your tests:
USERNAME=myUser PASSWORD=myPassword npx playwright test
In your test files, you can access these environment variables:
const username = process.env.USERNAME;
const password = process.env.PASSWORD;
Shared Setup with Storage State
A more complex method involves creating a shared setup that is executed before all tests. This could involve logging into the application through the UI and saving the session state, which is then reused across tests.
Here’s a brief example:
beforeAll(async () => (
await page.goto('/login');
await page.fill('input[name="username"]', 'yourUsername');
await page.fill('input[name="password"]', 'yourPassword');
await page.click('button[type="submit"]');
await page.context().storageState({ path: 'state.json' ));
});
This way, when subsequent tests are run, they will have access to the saved storage state.
Important Considerations
- The approaches outlined above work well in sequential test execution. However, if you're running tests in parallel, you will need to modify your functions to prevent data conflicts.
- A potential strategy can be employing lazy loading or checking if the data already exists before re-fetching it, ensuring that the data is only retrieved when necessary.
In conclusion, sharing data among tests can significantly reduce redundancy and improve clarity in your test scripts. Choose the method that best fits your testing structure and needs.
Keywords
- Playwright
- Data sharing
- JavaScript
- Tests
- Variables
- Environment variables
- Storage state
- Sequential mode
- Parallel execution
FAQ
Q1: Can I share data between tests when running in parallel?
A1: The methods described primarily support sequential execution. For parallel tests, consider using lazy loading or check if the data already exists before performing API calls.
Q2: How do I store sensitive information without hardcoding?
A2: You can use environment variables to store sensitive data such as usernames and passwords. Set them in your terminal before running tests.
Q3: What is the easiest way to share an authorization token across tests?
A3: Declare a variable at the top of your spec file and assign it within a beforeAll
hook, making it accessible throughout your tests.
Q4: How can I clean up data after tests?
A4: After creating or modifying data within your tests, include cleanup logic to delete or verify the state of that data (e.g., checking for a 404 status code after deletion).