Plot Realtime Indicators using Tradingview Charting Library - Javascript, NodeJS
Education
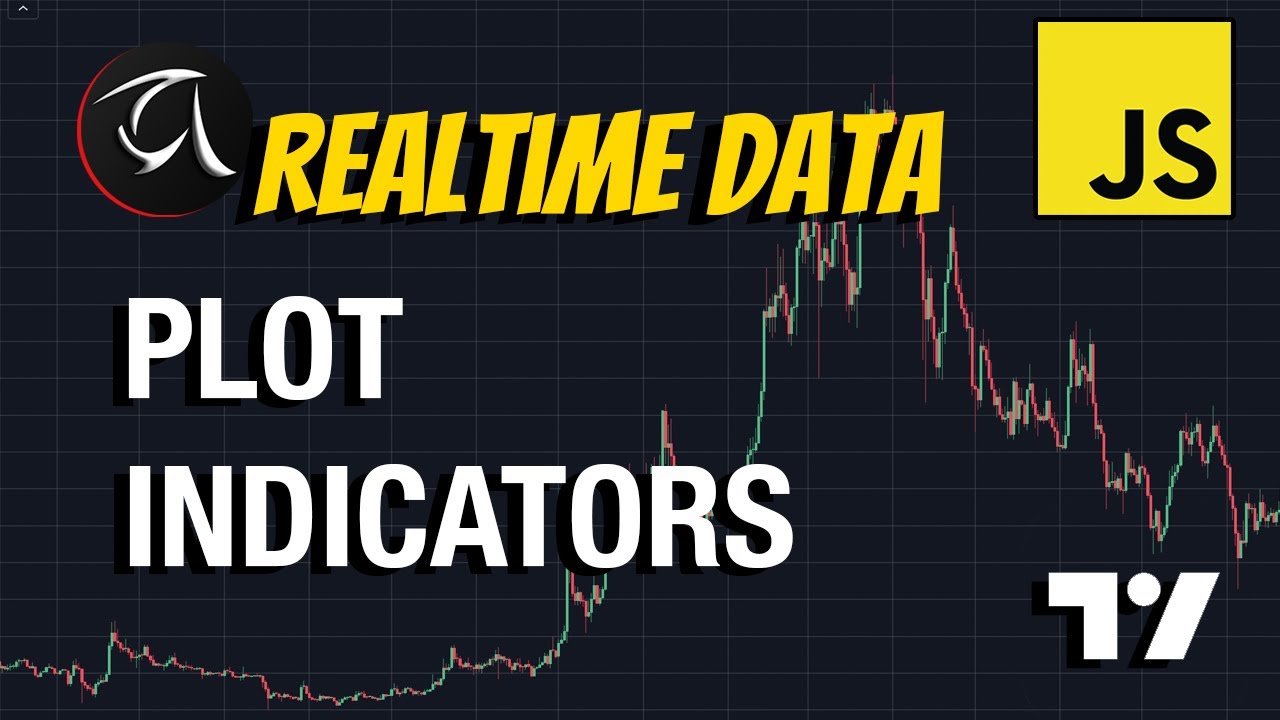
Introduction
In this article, we'll walk you through the process of plotting indicators using real-time data from cryptocurrency exchanges. In contrast to previous tutorials that focused solely on historical data, we will work on integrating live data into our visualizations.
Initial Setup
Before we begin coding, it’s important to outline the project structure. We'll create two main folders: one for the client-side code and another for the server-side (proxy) code. The real-time data requires API calls to the exchange, which cannot be made directly from the browser; hence, we will need to use a proxy server.
- Project Folders:
client
: For the client-side code.proxy
: For the server-side code.
To set up the proxy server, we initialize the project and install the necessary dependencies:
npm init -y
Next, we install the required packages:
- Express: An essential framework for creating the server.
- CORS: To avoid Cross-Origin Resource Sharing issues in browsers.
- Socket.IO: To establish real-time communication between server and client.
- WebSocket (ws): To create a WebSocket connection to the Binance exchange.
- WJS Indicators: For calculating technical indicators.
After installing these dependencies, we will also add Nodemon as a development dependency to automatically restart the server on code changes.
Creating the Express Server
We'll create a simple Express server to listen on port 4000. We’ll also handle CORS configuration. Within the proxy folder, we create a class called Klines
, which will manage candlestick data (both historical and real-time) using a Map to store the candlestick information for better performance.
Setting Up the Klines Class
The Klines
class will include methods to:
- Retrieve the last 1,000 bars of historical data.
- Fetch the real-time updates via WebSocket from Binance.
Inside the constructor, we set the default symbol to BTC/USDT
and the interval to 1 hour
. The initialize
method retrieves the historical data, formats it, and prepares it for the TradingView Library.
The getKlines
method will allow access to the stored candlestick data.
Upon establishing a new Socket.IO connection, we emit the historical candlestick data to the client.
Client-Side Code
Next, we move to the client-side implementation. The client will consist of:
index.html
: To display the chart.- Importing required scripts for Socket.IO and the lightweight charting library.
In a separate file, Kline.js
, we create a class KlineChart
that handles the rendering logic, establishes the chart, and manages the candlestick series. The loadHistoricalData
method will be used to populate the initial candlestick chart.
Establishing Real-Time Data
We will modify the Klines
class on the server-side to implement real-time updates. After retrieving the historical candlestick data, we will set up a WebSocket connection to receive live candlestick updates.
Each time a new candlestick is received, we will update our map structure and emit the data to the client. On the client-side, we will differentiate between historical and real-time data using conditions in our Socket.IO handlers.
Adding Indicators
We will enrich our visualization by adding key indicators like:
- Simple Moving Average (SMA)
- Exponential Moving Average (EMA)
- Relative Strength Index (RSI)
- Moving Average Convergence Divergence (MACD)
Each of these indicators will be implemented in specific sections of the Klines class, followed by creating a corresponding representation in the KlineChart
class on the client side.
*For example, to implement the SMA, we will:
- Import the Simple Moving Average function from WJS Indicators.
- Initialize it with the desired length (e.g., 100).
- Calculate the SMA value for historical data and update it accordingly in real-time using corresponding functions in the client.*
Final Thoughts
After implementing and updating all indicators, you will have a robust chart with real-time updates and various indicators plotted accurately.
Keyword
- Real-time Data
- TradingView Charting Library
- Javascript
- NodeJS
- Candlestick Chart
- Express Server
- Socket.IO
- API Calls
- Technical Indicators (SMA, EMA, RSI, MACD)
FAQ
Q1: What technologies do I need to plot real-time indicators?
A1: You will need NodeJS for server-side, a lightweight charting library for visualization, and WebSocket for real-time data.
Q2: How does the proxy server work in this setup?
A2: The proxy server handles API calls to fetch live data from the exchange and then broadcasts that data to the client using WebSockets.
Q3: Can I add more indicators after this?
A3: Yes, you can easily add more indicators by importing them from the WJS Indicators library and following a similar implementation process.
Q4: Why use a Map instead of an array or object for storing candlestick data?
A4: Using a Map provides better performance for adding and retrieving data, especially when using non-string keys and methods to manage data efficiently.
By following the steps outlined in this article, you'll be able to create a fully functional real-time trading chart with various technical indicators. This project demonstrates the power of combining web technologies to build engaging data visualizations.