Random Quote Generator | Javascript Beginner Project Tutorial
Science & Technology
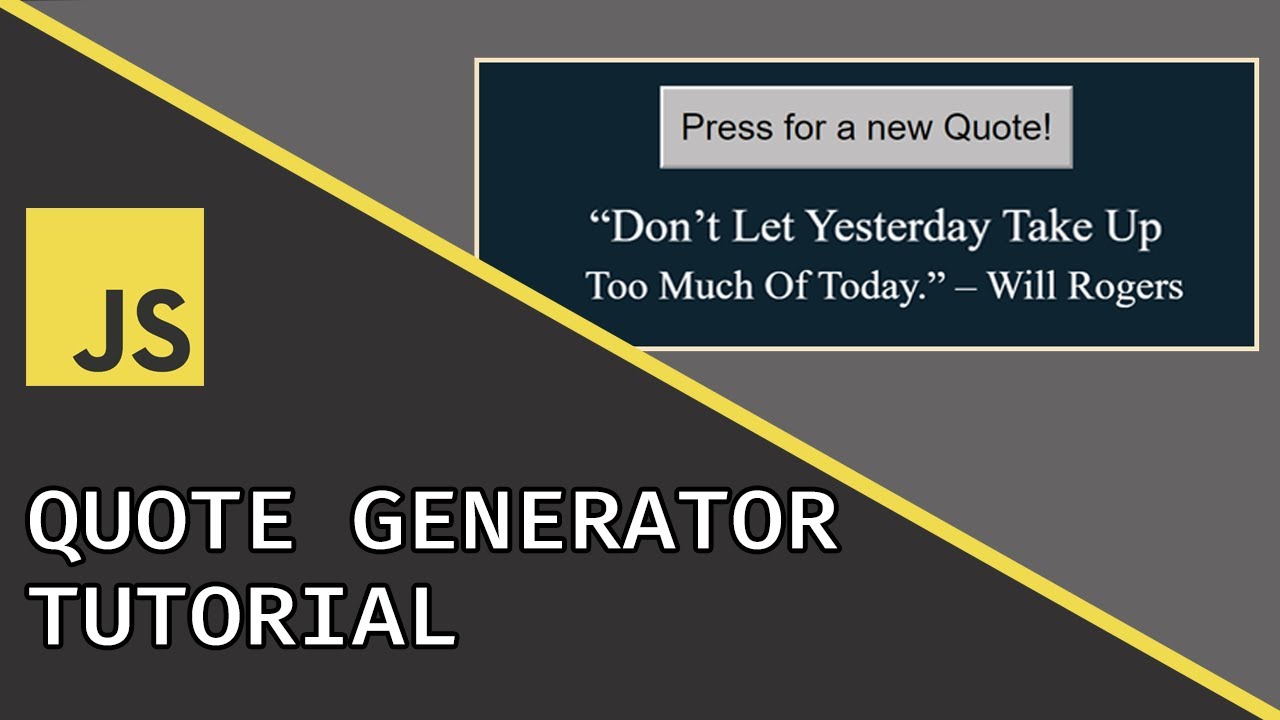
Random Quote Generator | JavaScript Beginner Project Tutorial
Welcome to the 52 JavaScript Projects in 52 weeks challenge! The project of the fifth week will be a code generator. Below is a detailed guide on how to create a Random Quote Generator using HTML, CSS, and JavaScript.
Step 1: Create the HTML Structure
Start by creating the basic HTML structure. Inside the body
tag, create a div
with an id
of container
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Random Quote Generator</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="container">
<button id="btn">Press for a new quote</button>
<div id="output">Press the button to generate a quote</div>
</div>
<script src="scripts.js"></script>
</body>
</html>
Step 2: Style with CSS
Next, create a CSS file named styles.css
to make your HTML elements look better.
body (
background-color: #2c3e50;
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
color: white;
)
#container (
background-color: #0e2431;
border: 5px solid #127a75;
width: 750px;
text-align: center;
padding: 50px 0;
border-radius: 10px;
)
#btn (
margin: 50px 0;
padding: 10px 50px;
font-size: 22px;
color: white;
background-color: rgb(129, 121, 121);
border: none;
border-radius: 5px;
cursor: pointer;
)
#btn:hover (
background-color: #34495e;
)
#output (
font-size: 26px;
)
Step 3: Adding Functionality with JavaScript
Now, create a JavaScript file named scripts.js
to add the functionality to generate and display random quotes.
const button = document.getElementById('btn');
const output = document.getElementById('output');
const quotes = [
"\"The greatest glory in living lies not in never falling, but in rising every time we fall.\" - Nelson Mandela",
"\"The way to get started is to quit talking and begin doing.\" - Walt Disney",
"\"Your time is limited, so don't waste it living someone else's life.\" - Steve Jobs",
"\"If you set your goals ridiculously high and it's a failure, you will fail above everyone else's success.\" - James Cameron",
"\"Life is what happens when you're busy making other plans.\" - John Lennon",
"\"Spread love everywhere you go. Let no one ever come to you without leaving happier.\" - Mother Teresa",
"\"When you reach the end of your rope, tie a knot in it and hang on.\" - Franklin D. Roosevelt",
"\"Always remember that you are absolutely unique. Just like everyone else.\" - Margaret Mead",
"\"Don't judge each day by the harvest you reap but by the seeds that you plant.\" - Robert Louis Stevenson",
"\"The best and most beautiful things in the world cannot be seen or even touched - they must be felt with the heart.\" - Helen Keller"
];
button.addEventListener('click', function() (
const randomQuote = quotes[Math.floor(Math.random() * quotes.length)];
output.innerHTML = randomQuote;
));
Keyword
- HTML structure
- Button
- Output div
- CSS styling
- JavaScript functionality
- Random Quote Generator
- Event listener
- Array of quotes
- Math.random
FAQ
Q1: What does Math.floor
do in JavaScript?
A1: Math.floor
rounds down to the nearest whole number. It's often used in combination with Math.random
to get a random index from an array.
Q2: How can I style elements in the center of the page?
A2: Using CSS Flexbox, you can center elements by setting the body with display: flex
, justify-content: center
, and align-items: center
.
Q3: What does the document.getElementById
method do?
A3: document.getElementById
selects an HTML element with a specific ID so you can manipulate it using JavaScript.
Q4: Why use a separate CSS file? A4: Separating CSS into its own file helps to keep the code organized and improves maintainability.
Q5: Can I add more quotes to the generator?
A5: Yes, you can simply add more items to the quotes
array in the JavaScript file.