Speech Recognition & Voice Synthesis in React (Web Speech API)
Science & Technology
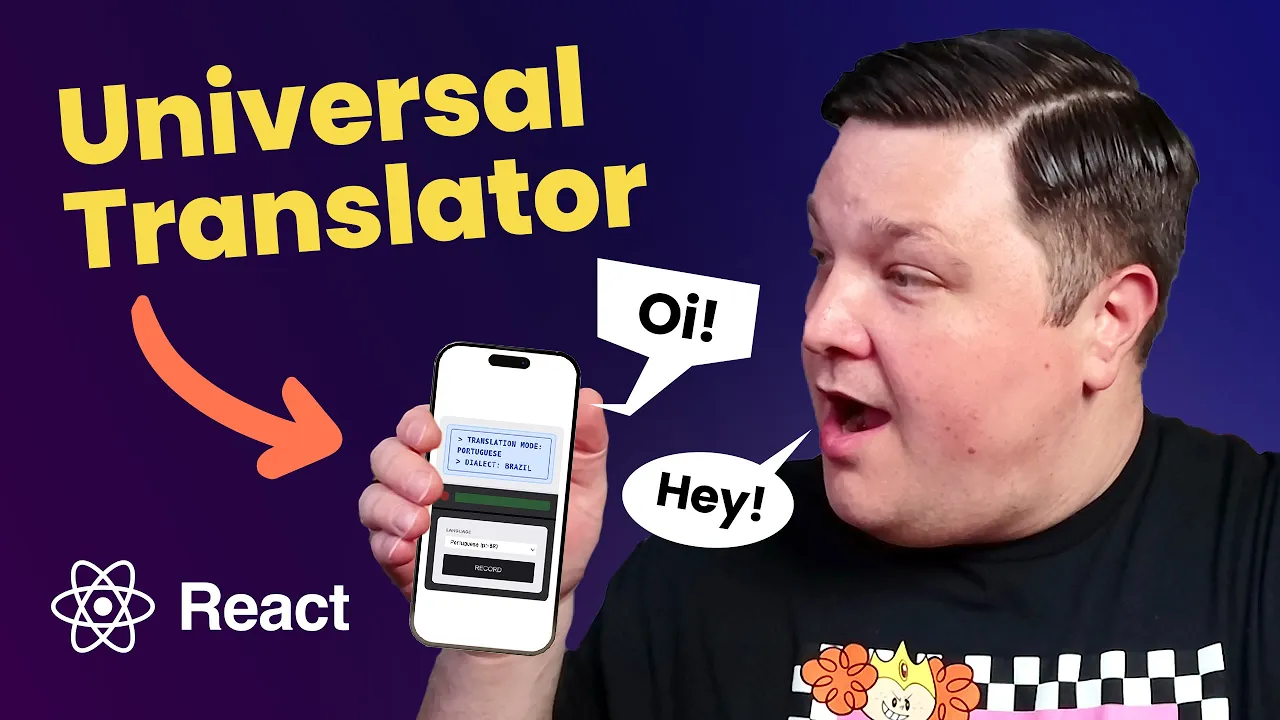
Introduction
Language is a critical component of our globalized world, and having a universal translator would be amazing. In this article, we'll explore how to build our own speech translation application using React and the Web Speech API. We'll use speech recognition to convert spoken words into text and then utilize speech synthesis to translate that text into different languages and have it spoken back to us. Let's dive in!
Step 1: Speech Recognition
To begin, we'll focus on implementing speech recognition in our React application. We'll use the Web Speech API's SpeechRecognition interface for this purpose, specifically the start
method to initiate the recording. However, it's important to note that browser support for these APIs can be limited. Desktop Chrome usually provides the best support, while Safari has some limitations. Firefox may not work at all, so be sure to use a compatible browser during development.
First, we'll add a click event handler to the record button in our application. When the button is clicked, we'll trigger the handleOnRecord
function. Inside this function, we'll access the window.[SpeechRecognition
API](https://www.topview.ai/blog/detail/speech-recognition-in-python "Speech Recognition in Python"), making sure to include the webkitSpeechRecognition
for Safari support. We'll also install the @types/speech-recognition
package to resolve the TypeScript issues related to the API's types.
Next, we'll create a recognition
constant and set it to a new instance of SpeechRecognition
. To test if the speech recognition functionality is working, we can call the recognition.start()
method. This will prompt the browser to request microphone access. Clicking "Allow" will activate the recording indicator.
To capture the text from the recorded speech, we'll define a callback handler using the recognition.onresult
event. This handler will receive an event object, and we can extract the transcript
property from the event to obtain the recorded text. We'll store this transcript value in a text
state using the useState
hook and display it on the page.
Step 2: Translation with OpenAI
Now that we have the recorded text, let's move on to translation using the OpenAI API. We'll specifically use the OpenAI SDK and the chat completions API to translate the text. In our API route, we'll create an async post
function to handle the translation request. Inside this function, we'll use the OpenAI SDK to send the text prompt to the API and receive the translated text as a response. We'll return this translated text as JSON.
Back in our React component, we'll make a fetch request to our API route, passing in the recorded text and the desired translation language. We'll set the method to POST and stringify the JSON body. After receiving the API response, we'll store the translated text in a translation
state using the useState
hook and display it on the page.
Step 3: Voice Synthesis
Now that we have the translated text, let's proceed to voice synthesis, where we'll have the translated text spoken out loud. We'll continue to use the Web Speech API, but this time we'll utilize the SpeechSynthesis interface.
To start, let's create a new instance of SpeechSynthesisUtterance
, which represents the text that will be spoken. We'll then use the SpeechSynthesis
instance to speak the generated utterance. By setting the voice
property of the utterance to an available voice, we can control the language and accent of the synthesized speech. We'll update the available voices using the window.speechSynthesis.getVoices()
method and select an appropriate voice based on language preference.
Within our React component, we'll create a speak
function that takes the translated text as input. We'll abstract the logic for voice synthesis into this function and invoke it after receiving the translation response. The synthesized text will be set as the text
property of the utterance. Finally, we'll trigger the speech synthesis by calling speechSynthesis.speak(utterance)
.
Summary of the Article
Keywords: Language, Globalization, Universal Translator, Web Speech API, React, Speech Recognition, Speech Synthesis, OpenAI SDK, Translation, Voice Synthesis, Language Selector.
FAQ
FAQ:
Q1. What is the Web Speech API? The Web Speech API is a collection of browser-based speech recognition and voice synthesis functionalities that enable developers to integrate speech-related features into web applications.
Q2. Does the Web Speech API work in all browsers? No, browser support for the Web Speech API is limited. Desktop Chrome provides the best support, while Safari has some limitations. Firefox may not support these APIs.
Q3. Which API is used for translation in this article? OpenAI's chat completions API is used for translation in this article. It allows developers to send text prompts and receive translated responses using the OpenAI SDK.
Q4. How can I customize the translation languages? You can customize the translation languages by modifying the available options in the language selector. The OpenAI API supports a wide range of languages for translation.
Q5. Can I use this speech translation app on mobile devices? Yes, you can use this speech translation app on mobile devices. However, browser limitations and performance may vary across different browsers and devices.
Q6. Are there any security concerns with using speech recognition and translation APIs? There may be security concerns when using speech recognition and translation APIs, especially when handling sensitive data. It is crucial to follow best practices for secure web development and protect user privacy.
Q7. Can I improve the accuracy of speech recognition? Yes, you can improve the accuracy of speech recognition by providing clear pronunciation, minimizing background noise, and using proper speech patterns.
Q8. Can I add additional features to this speech translation app? Absolutely! You can enhance this speech translation app by adding features such as language detection, real-time transcription, and integration with other APIs like natural language processing for better translations.
Q9. How can I deploy this speech translation app? You can deploy this speech translation app to a hosting service like Vercel, Netlify, or a cloud-based server. Simply build your React app and deploy the generated build files to the hosting environment of your choice.