Split a String Into the Max Number of Unique Substrings - Leetcode 1593 - Python
Science & Technology
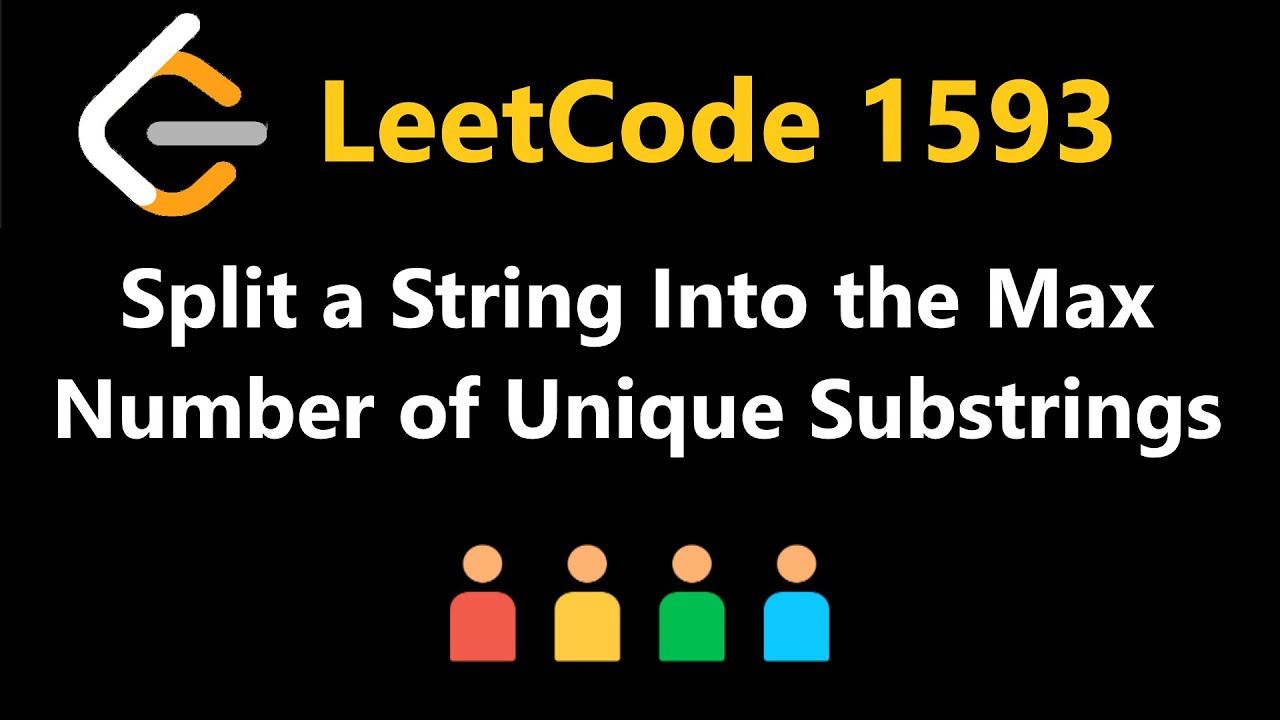
Introduction
In today's article, we'll tackle the Leetcode problem of splitting a string into the maximum number of unique substrings. This problem showcases a common challenge in algorithm design and is particularly suited to approaches like backtracking.
Understanding the Problem
Suppose we are given a string, and we want to determine the maximum number of non-overlapping, distinct substrings we can extract from this string. For instance, consider the string "abacaba". We could split it into several distinct substrings like this:
- "a"
- "b"
- "c"
- "ab"
- "aba"
In this case, the maximum distinct substrings we can form is 5. The challenge lies in determining whether we can find more than five unique substrings.
Initial Thoughts on Approach
Before jumping straight into a brute-force solution, it's crucial to consider more efficient alternatives if they exist. Starting from the first character in the string, we can observe that each substring must begin with the current character. This leaves us with numerous choices to make, each leading us down multiple potential paths in our solution.
For a string of length n
, each choice has a significant effect on the number of valid substrings. Although we could be greedy and try to take each character as its own substring, this approach may lead to a situation where we overlook potentially larger unique substrings that could be formed by combining characters together.
To efficiently recognize and handle duplicates, we can utilize a hash set. This data structure allows us to detect already used substrings in constant time.
Backtracking Approach
Given the complexity of this problem, a backtracking approach is appropriate. Through backtracking, we can explore all possible splits of the string while checking for the uniqueness of each substring.
Recursion: In this problem, we'll use a recursive function that takes the current index of the string and a hash set of previously formed substrings. The goal is to find the maximum number of unique substrings from this index onward.
Base Case: If we've reached the end of the string, we cannot form any more substrings, so we return zero.
Recursive Exploration: We iterate through each possible ending index of the substring starting from the current index. For each substring formed, we check if it's already in the hash set. If it's unique, we add it and recursively call the function to account for the rest of the string.
Backtracking: After exploring a path, we need to remove the added substring from the hash set (this "undoes" our action), allowing other paths to use it if needed.
Here’s how we can implement this backtracking solution in Python:
def maxUniqueSplit(s: str) -> int:
def dfs(index: int, unique_set: set) -> int:
if index == len(s):
return 0
result = 0
for j in range(index, len(s)):
substring = s[index:j + 1]
if substring not in unique_set:
unique_set.add(substring)
result = max(result, 1 + dfs(j + 1, unique_set))
unique_set.remove(substring) # backtrack
return result
return dfs(0, set())
Time Complexity Analysis
Analyzing the time complexity is essential. The total number of possible partitions of the string can lead to an exponential number of recursive calls, especially since we are dealing with two choices at each character—that is, to partition or not to partition.
A rough estimate of the time complexity would be O(n^2 * 2^n)
, where n
is the length of the string. However, the actual performance can be better in practice since not all recursive paths will be explored due to the unique checks.
Conclusion
In summary, this problem emphasizes a well-structured backtracking approach paired with efficient data structure usage for uniqueness checks. Leveraging recursion and hash sets can dramatically simplify the complexity of substring checks, promising a feasible solution even for non-trivial string lengths.
Keywords
- Leetcode
- Unique Substrings
- Backtracking
- Python
- Hash Set
- Recursive Function
- Time Complexity
FAQ
1. What is the problem statement for Leetcode 1593?
The problem requires finding the maximum number of unique substrings that can be formed from a given string.
2. Why is a backtracking approach suitable for this problem?
Backtracking allows exploration of all potential substring partitions while managing the complexity of duplicate checks effectively.
3. How does the hash set help in solving this problem?
The hash set enables constant-time checks for substring uniqueness, preventing the inclusion of duplicates in the count.
4. What is the time complexity of the solution?
The theoretical time complexity is O(n^2 * 2^n)
, where n is the length of the string, though practical performance may be better due to early terminations in recursion.
5. Can this problem be solved using a greedy approach?
While a greedy approach might suggest taking each character separately, it does not guarantee the maximum number of unique substrings can be reached, as it may miss larger distinct combinations.