Use AI (Bolt.new) to create AI service (Code it easy with Suzie - Chapter 4) #bolt.new #googlegemini
People & Blogs
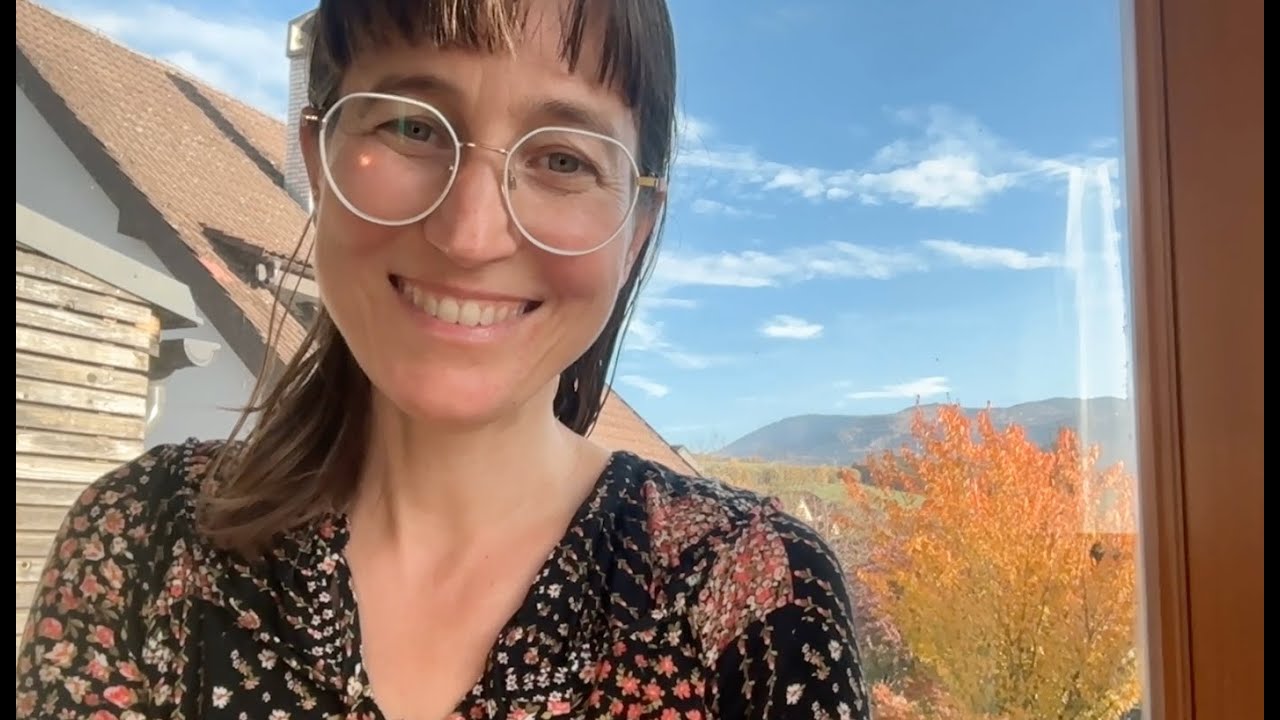
Introduction
Today is a wonderful hot day outside, and to match the fantastic weather, we're diving into a hot topic: artificial intelligence. In this chapter, we will utilize an AI development tool called Bolt.new to create a service that leverages AI, specifically using Google Gemini for travel tips.
Getting Started
To kick things off, we'll write a prompt to create an Angular service that communicates with Google Gemini, providing travel tips. The initial setup will generate an Angular application along with the necessary package and configuration files.
Once the project is ready, you'll notice a prompt that instructs you to replace the API key in the travel.service.ts
file with your actual Google Gemini API key. If you don't have one yet, don’t worry! We will cover how to obtain it shortly.
Setting up the Service
Let's move ahead and copy the service code generated by Bolt.new into our local project. In your project folder, create a new directory called service
and inside it, add a new file named gemini.service.ts
. Paste the code provided by Bolt into this file.
Understanding the Service
This service functions by communicating with the Google Gemini API. Essentially, you can request travel tips through a method called getTravelTips
. This method constructs a prompt, sends it to the Gemini server, and waits for a response. If the request fails, it will log an error in the console.
Next, we need to install the Google Generative AI package. You can run:
npm install @google-generative-ai
Creating the API Key
To utilize Google Gemini, you first need to create an API key. Go to the Google AI Studio and sign in. If you have existing projects, you can select one; otherwise, just click on "Create API Key." Once you have the key, copy it and paste it into the service file, ensuring to keep it secret.
Modifying the Service
Now that we have the service set up, we need to make some modifications. We'll rename the service file from travel.service.ts
to gemini.service.ts
to be more accurate.
Inside the service, we have two methods returning data asynchronously. For simplicity, let's work with promises instead of observables. Make the necessary changes to the imports and simplify the asynchronous logic.
Integrating with the Component
Now, let’s return to the App Component file and inject our newly configured Gemini service. We'll use this service within the existing method that handles user input.
Update the explore
method to call getTravelTips
instead of displaying an alert. Use the await
keyword to ensure the service completes before we attempt to log the results.
Here is what it would look like:
const tips = await this.geminiService.getTravelTips(location);
console.log(tips);
After saving the project, you can test the implementation by running your Angular application and looking for the output in the console.
Conclusion
Congratulations! You have successfully created your first AI service using an AI development tool. This service can provide travel tips based on user inputs via Google Gemini. Feel free to modify the prompt to explore different requests, whether it's querying recipes or pondering philosophical questions.
Play around with the prompts and see the variety of responses you can receive!
Keywords
- AI
- Bolt.new
- Google Gemini
- Angular
- API Key
- Service
- Travel Tips
- Asynchronous Methods
FAQ
Q1: What is Bolt.new?
A1: Bolt.new is an AI development tool that allows users to quickly generate applications based solely on prompts.
Q2: How do I get my Google Gemini API key?
A2: You can obtain your API key by signing into Google AI Studio and creating a new project or selecting an existing one.
Q3: What type of information can I query using the AI service?
A3: You can request various types of information, such as travel tips, recipes, or even philosophical answers, by modifying the prompts sent to the Google Gemini service.
Q4: What programming language is used in this project?
A4: The project is built using Angular and TypeScript for the service implementation.
Q5: How do I view the output of the service?
A5: You can view the output by opening the Developer Tools in your browser and navigating to the console section after running the application.