Voice Assistant with Wake Word in Python
Science & Technology
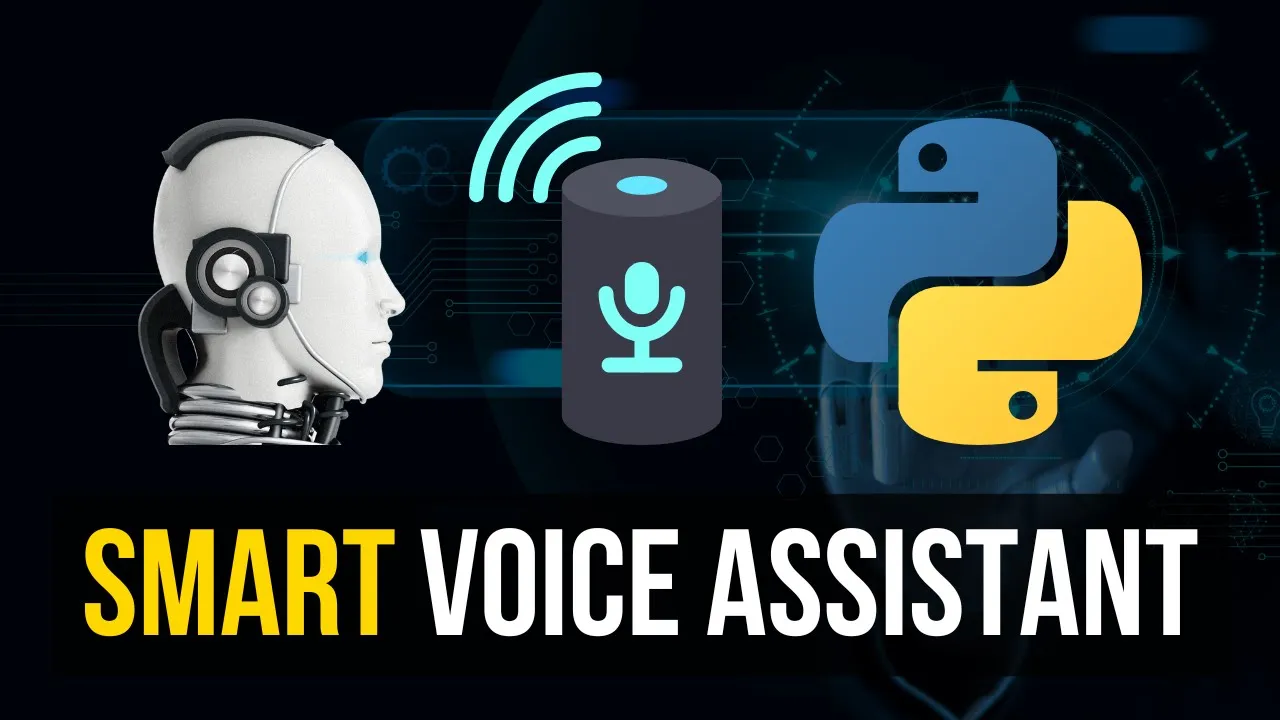
Voice Assistant with Wake Word in Python
In this article, you will learn how to build an intelligent voice assistant in Python that reacts to a wake word. The assistant will not process any sound unless it hears the specific wake word you designate. Here, we'll name our assistant "Jake" and use "Hey Jake" as the wake word. The assistant will activate upon hearing the wake word and perform a set of predefined tasks based on your commands.
Demo Preview
Before diving into the implementation, let's see what we aim to achieve. Running the script initializes a graphical user interface (GUI), showcasing a bot icon. When the bot is inactive, the icon is black. It turns red upon hearing the wake word ("Hey Jake"), indicating it's ready to process commands. Commands such as "What is neural nine?" will be processed and answered, and the bot reverts to an inactive state, turning the icon back to black.
Project Dependencies
To build this voice assistant, we need three external Python modules:
speech_recognition
for converting speech to text.neuralintents
for the core assistant functionality.pyttsx3
for turning text responses into speech output.
Install these modules using pip:
pip install speechrecognition
pip install neuralintents
pip install pyttsx3
Setting Up the Assistant Class
Create a new Python class named Assistant
to encapsulate the entire voice assistant logic. Import the necessary libraries and set up the recognizer, speaker, and assistant model.
import sys
import threading
import tkinter as tk
import speech_recognition as sr
import pyttsx3 as tts
from neuralintents import GenericAssistant
class Assistant:
def __init__(self):
self.recognizer = sr.Recognizer()
self.speaker = tts.init()
self.speaker.setProperty('rate', 150)
self.assistant = GenericAssistant('intents.json', intent_methods=('file': self.create_file))
self.assistant.train_model()
self.root = tk.Tk()
self.label = tk.Label(self.root, text='?', font=('Arial', 120))
self.label.pack()
threading.Thread(target=self.run_assistant).start()
self.root.mainloop()
Creating the Intents File
Create a JSON file named intents.json
to determine the bot's capabilities. This file will include tags, patterns, and responses for different commands.
(
"intents": [
{
"tag": "greeting",
"patterns": ["Hi", "Hey", "What's up?", "Hello"],
"responses": ["Hello!", "How can I assist you?"]
),
(
"tag": "neural_9",
"patterns": ["What is neural nine?", "Tell me about neural nine?"],
"responses": ["Neural 9 is a YouTube channel focused on programming."]
),
(
"tag": "name",
"patterns": ["What's your name?", "Who are you?"],
"responses": ["My name is Jake."]
),
(
"tag": "file",
"patterns": ["Create a file"],
"responses": []
)
]
}
Running the Assistant
The run_assistant
function is responsible for continuously listening to audio input, checking for the wake word, and processing subsequent commands.
def run_assistant(self):
while True:
try:
with sr.Microphone() as mic:
self.recognizer.adjust_for_ambient_noise(mic, duration=0.2)
audio = self.recognizer.listen(mic)
text = self.recognizer.recognize_google(audio).lower()
if "hey jake" in text:
self.label.config(fg='red')
audio = self.recognizer.listen(mic)
text = self.recognizer.recognize_google(audio).lower()
if text == "stop":
self.speaker.say("Bye")
self.speaker.runAndWait()
self.speaker.stop()
self.root.destroy()
sys.exit()
if text:
response = self.assistant.request(text)
if response:
self.speaker.say(response)
self.speaker.runAndWait()
self.label.config(fg='black')
except Exception as e:
print(e)
self.label.config(fg='black')
continue
Creating a File
The create_file
method demonstrates how to connect a command to a Python function. In this case, the function creates a text file.
def create_file(self):
with open("some_file.txt", "w") as f:
f.write("Hello World")
Running the Script
Create an instance of the Assistant
class to run the script.
if __name__ == "__main__":
Assistant()
Running this script will activate your voice assistant, Jake. It will listen for the wake word, process commands, and perform actions based on your inputs.
Keyword
- Voice Assistant
- Wake Word
- Python
- Speech Recognition
- Neuralintents
- Pyttsx3
- GUI
FAQ
1. What modules are required to build this voice assistant?
You need speech_recognition
, neuralintents
, and pyttsx3
.
2. How can I install the required modules?
Use pip to install the modules:
pip install speechrecognition
pip install neuralintents
pip install pyttsx3
3. What is the purpose of the intents file?
The intents file specifies the different commands the assistant can handle, including sample phrases (patterns) and responses.
4. How does the assistant activate?
The assistant activates upon hearing the designated wake word, "Hey Jake", and then processes the following command.
5. How can I customize the assistant’s responses?
Modify the responses
field in the intents.json
file to customize the assistant’s replies.
6. Can I add more functionalities to the assistant?
Yes, you can add more functionalities by defining new tags and corresponding functions in the intents.json
file and your script.